For loop Java array
For loop Java array
I'll respond in English this time!
The for
loop is a fundamental control structure in programming that allows you to execute a block of code repeatedly for a specified number of iterations. In the context of arrays, the for
loop can be used to iterate through each element of an array.
Here's an example of how to use a for
loop to iterate through a Java array:
public class ForLoopArray {
public static void main(String[] args) {
// declare and initialize an integer array
int[] scores = {10, 20, 30, 40, 50};
// use a for loop to iterate through the array
for (int i = 0; i < scores.length; i++) {
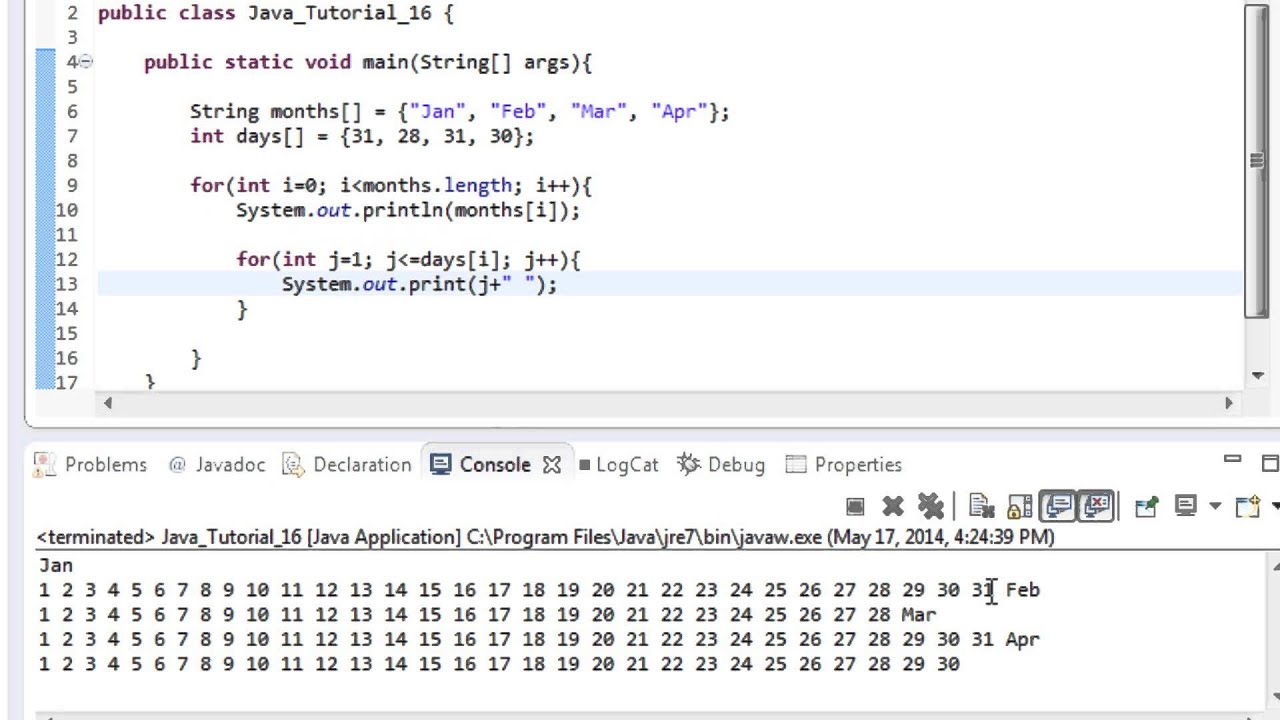
System.out.println("Score at index " + i + ": " + scores[i]);
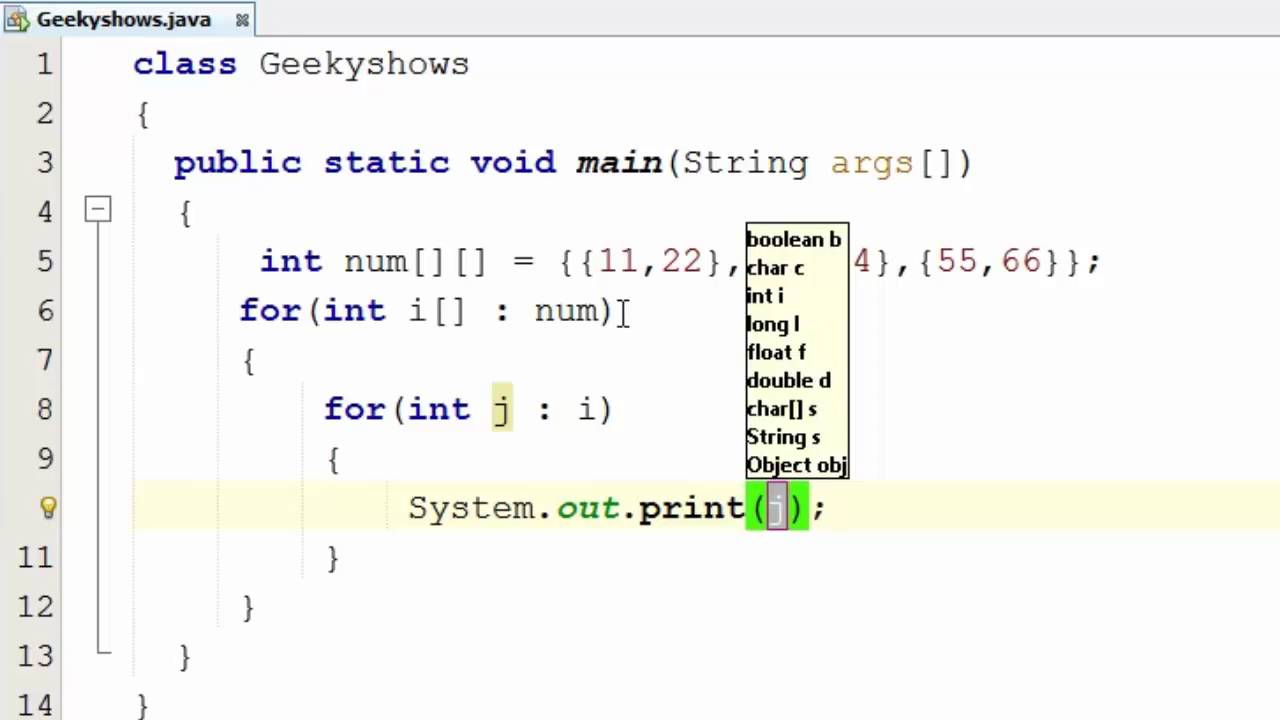
}
}
}
In this example:
Thescores
array is declared and initialized with five integer values. A for
loop is used to iterate through the array. The loop has three parts: Initialization: int i = 0;
Condition: i < scores.length;
Increment: i++
Inside the loop, a message is printed to the console indicating the score at each index. The loop will execute five times, once for each element in the array.
Here's another example that demonstrates using a for
loop to iterate through an array and modify its elements:
public class ForLoopArray {
public static void main(String[] args) {
// declare and initialize a double array
double[] temperatures = {23.4, 25.6, 28.9, 30.2};
// use a for loop to iterate through the array and square each temperature value
for (int i = 0; i < temperatures.length; i++) {
temperatures[i] = Math.pow(temperatures[i], 2);
}
// print the modified temperatures
for (double temp : temperatures) {
System.out.println("Modified Temperature: " + temp);
}
}
}
In this example:
Thetemperatures
array is declared and initialized with four double values. A for
loop is used to iterate through the array, and each temperature value is squared using the Math.pow()
method. After the first loop completes, a second for
loop is used to print the modified temperatures.
These examples illustrate how you can use a for
loop in Java to iterate through an array, execute code repeatedly, and modify or access array elements.
While loop Java
I'll respond in English and explain the concept of a while loop in Java.
A while loop is a type of control structure that allows you to execute a block of code as long as a certain condition is true. It's commonly used when you need to repeat a task until a specific condition is met.
Here's a basic syntax for a while loop in Java:
while (condition) {
// code to be executed
}
The condition is the part that determines whether the loop should continue or not. As long as the condition is true, the code inside the loop will be executed repeatedly until the condition becomes false.
Let's consider an example: you want to display a message "Hello" 5 times. Here's how you can do it using a while loop in Java:
int i = 0;
while (i < 5) {
System.out.println("Hello");
i++;
}
In this example, the condition is i < 5
. As long as i
is less than 5, the code inside the loop will be executed. The code increments i
by 1 each time it runs, so eventually i
becomes greater than or equal to 5, and the condition becomes false.
Here's what happens when you run this code:
i
is initially set to 0. The condition i < 5
is checked, and since i
is less than 5, the code inside the loop runs. "Hello" is printed to the console. i
is incremented by 1, so now i
becomes 1. The condition is checked again, and since i
is still less than 5, the code inside the loop runs again. This process repeats until i
becomes 5. When i
reaches 5, the condition becomes false, and the loop ends.
This example demonstrates how a while loop can be used to repeat a task until a specific condition is met.
Here are some key benefits of using a while loop:
Repetition: You can use a while loop to repeat a task as long as a certain condition is true. Condition-based execution: The code inside the loop will only run when the condition is true, making it useful for situations where you need to perform an action until a specific condition is met.While loops are a fundamental concept in programming, and understanding how they work is essential for any programmer.
Do you have any questions about while loops or would you like me to explain anything further?