What are different types of variables in Java?
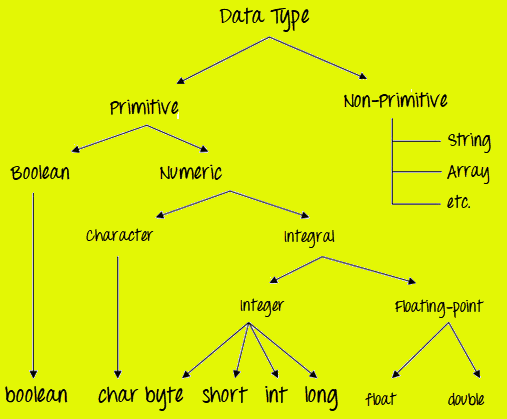
I'm happy to help! However, I'll respond in English as per your request.
In Java, there are several types of variables that serve distinct purposes and characteristics. These variable categories are crucial in programming, allowing developers to effectively manipulate and manage data within their applications. Let's delve into the different types of variables in Java:
Primitive Types: These are the most basic type of variables in Java, which have a fixed size and value. There are eight primitive types: boolean
: A boolean value (true or false). byte
, short
, int
, long
, and char
: Whole number values with varying sizes.
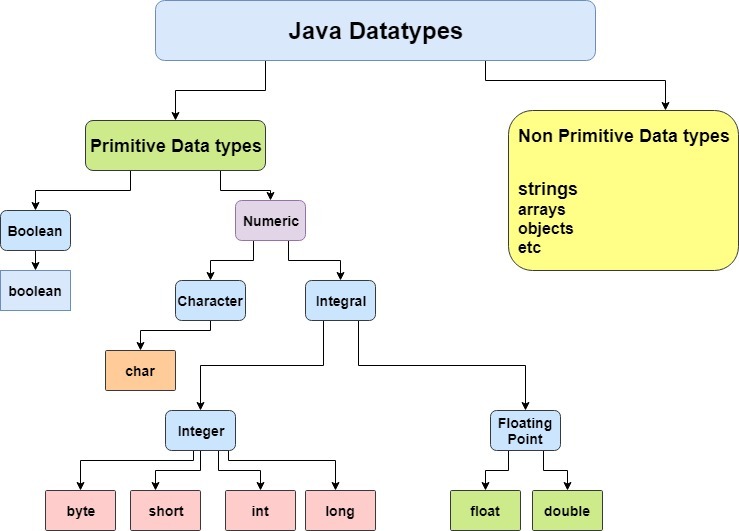
float
and double
: Decimal numbers with varying precision.
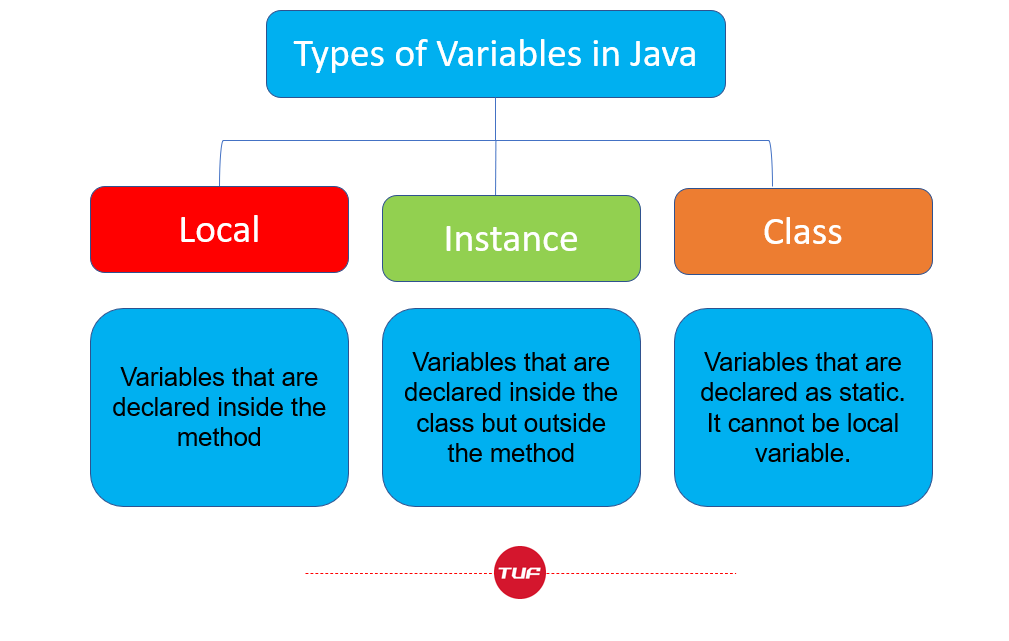
Reference Types: These variables store the memory addresses of objects, rather than their actual values. Java has two main categories under reference types:
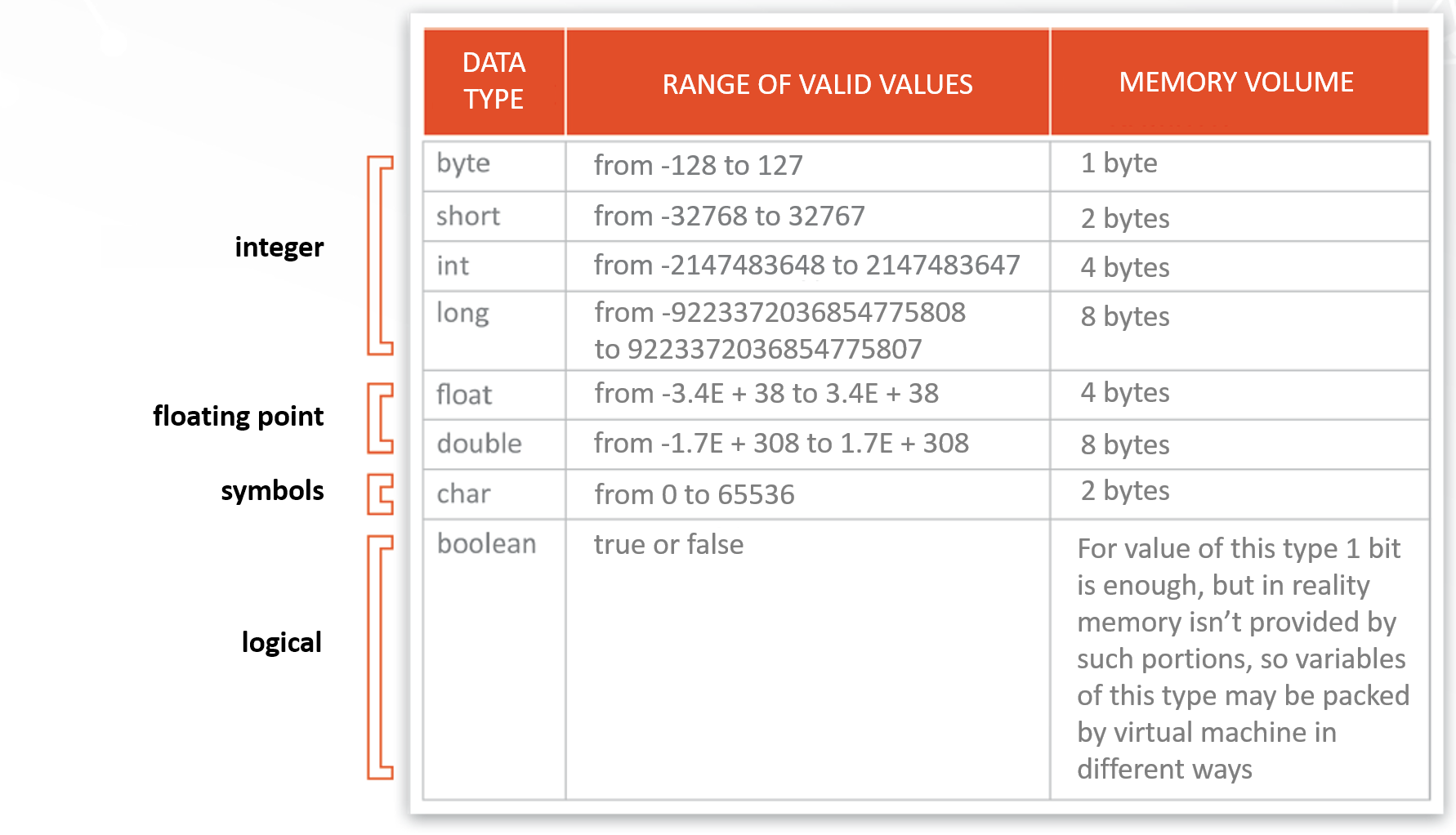
Class Variables (or Instance Variables): These belong to a specific class or object and can be accessed using the dot notation. Array Variables: These store arrays of values, which are essentially collections of primitive or reference variables. Local Variables: These variables are declared within a method and are only accessible within that scope. They are created on the stack at runtime and destroyed when the method finishes executing. Instance Variables (or Class Variables): These belong to an instance of a class, meaning each object of that class has its own copy of these variables. Static Variables: These belong to the class itself, not individual objects. There is only one copy of these variables in memory for all instances of the same class. Final Variables: These cannot be reassigned once they are initialized. They can still be accessed and used like any other variable. Transient Variables: These variables do not get serialized when an object's state needs to be persisted, unlike instance variables. Volatile Variables: These are used to declare variables that might change asynchronously (e.g., multi-threaded programming). The JVM will automatically generate the necessary synchronization code to ensure visibility and ordering of volatile variable accesses. Enum Constants: These represent a set of named values in a program, allowing developers to define their own custom types. Wildcard Variables (_
): These are used to catch any type of value, often used for error handling or debugging purposes.
In Java, it's essential to understand the different variable categories and how they interact with one another. By doing so, you'll be better equipped to create efficient, scalable, and maintainable code.
What are the 8 types of variables in java w3schools
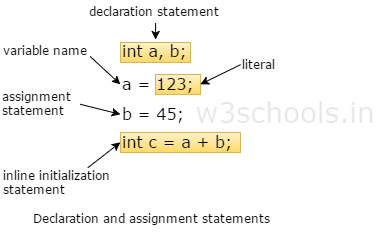
I'm glad you asked!
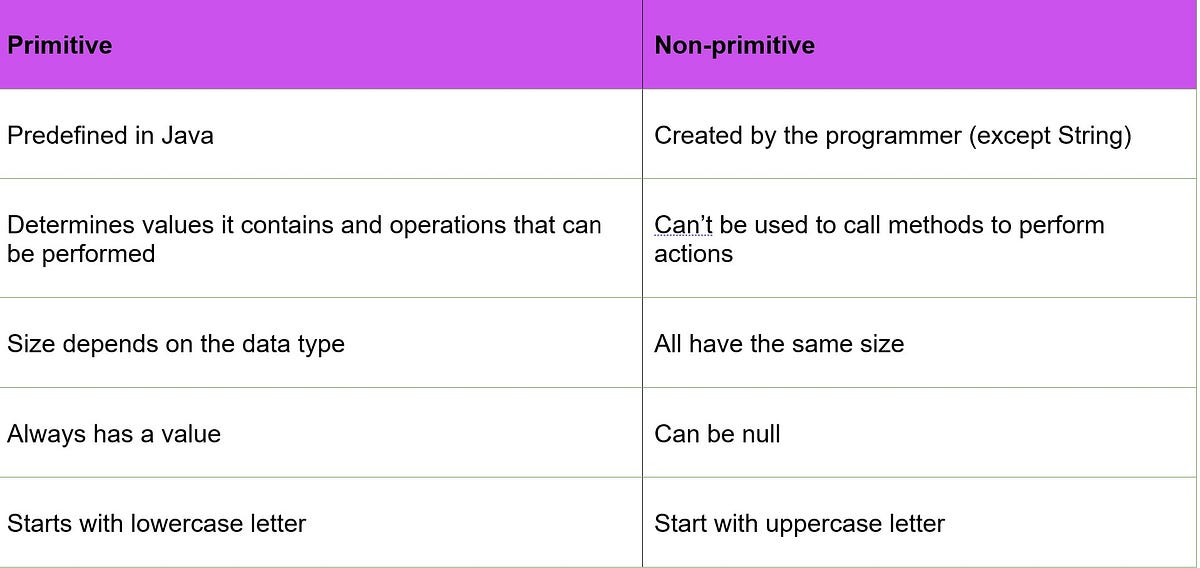
In Java, a variable is a storage location that holds a value. There are eight (8) main types of variables in Java, and understanding these categories can help you write more effective code. Here's a rundown of each type from W3Schools:
Local Variables: These variables are declared within a method or block of code and have their own scope. They cease to exist when the method or block is completed.
Example: int x = 10;
Instance Variables (also known as Non-Static Fields): These variables are part of an object's state and are created once for each instance of a class. Each instance has its own copy of these variables.
Example: public int age;
Static Variables: These variables belong to the class itself, not individual instances. There is only one copy of these variables, shared by all instances of the class.
Example: public static int counter = 0;
Class Variables (also known as Static Fields): These are similar to instance variables but apply to the class as a whole rather than individual objects.
Example: public static final int MAX_SIZE = 100;
Array Variables: These store multiple values of the same data type in a single variable. You can think of them as containers holding many values.
Example: int[] scores = {90, 80, 70};
Reference Variables (also known as Object References): These hold the memory addresses of objects created elsewhere in the code.
Example: Student student1 = new Student();
Boolean Variables: These can have only two values: true or false.
Example: boolean isAdmin = true;
Enum Variables: These allow you to define a set of named constants and their corresponding integer values.
Example: enum Color { RED, GREEN, BLUE };
It's essential to understand the differences between these variable types when working with Java. Using the correct type can prevent errors, improve code readability, and enhance overall program efficiency.