What is cnn in java geeksforgeeks
What is cnn in java geeksforgeeks
I'd be happy to help!
Cnn stands for Convolutional Neural Network, a type of deep learning algorithm primarily used for image and speech recognition tasks. In Java, you can implement CNN using various libraries such as Weka, Deeplearning4j, or DeepLearningJava.
Here's a brief overview of how CNN works:
Convolutional Layers: These layers apply filters to small regions of the input data (images), scanning the entire image with these filters to capture local features. Pooling Layers: These layers reduce the spatial dimensions of the input, reducing the number of parameters and computation required. Flatten Layer: This layer reshapes the output from the convolutional and pooling layers into a 1D array for further processing. Dense (Fully Connected) Layers: These layers use fully connected neural networks to classify or make predictions on the input data.Now, let's talk about how you can implement CNN in Java using GeeksforGeeks' tutorials and examples:
Step 1: Install necessary libraries
You'll need Weka (Waikato Environment for Knowledge Analysis) or Deeplearning4j (DLP4J) to work with neural networks. For this example, we'll use DLP4j.
gradle init --type java-library
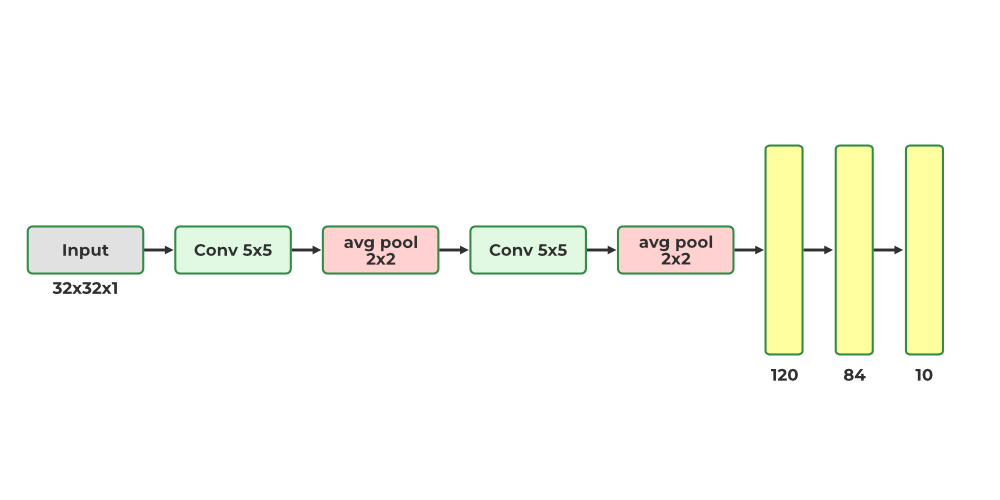
Step 2: Set up your project structure
Create the following directories and files:
src/main/java
: for Java source code src/test/java
: for test classes build.gradle
: for Gradle build settings
Step 3: Implement CNN in Java using DLP4j
Here's a simple example of implementing a Convolutional Neural Network using DLP4j:
import org.deeplearning4j.nn.conf.ConvolutionMode;
import org.deeplearning4j.nn.conf.layers.Conv1DOutputActivation;
import org.deeplearning4j.nn.conf.layers.Conv1DEntry;
import org.nd4j.linalg.api.ndarray.INDArray;
public class ConvolutionalNeuralNetwork {
public static void main(String[] args) {
// Define the neural network configuration
Nd4jWorkspace workspace = new Nd4jWorkspace();
INDArray input = null; // Input data
// Define the convolutional layer
Conv1DEntry convLayer = new Conv1DEntry(10, 5)
.activation(Conv1DOutputActivation.RELU)
.convolutionMode(ConvolutionMode.SAME)
.upSample(false);
// Define the pooling layer
PoolingLayer poolLayer = new PoolingLayer(2); // 2x2 max-pooling
// Create the neural network
ComputationGraph net = new ComputationGraph(new InputLayer(input))
.add(convLayer)
.add(poolLayer);
// Train the model using your favorite algorithm (e.g., backpropagation)
}
}
This code sets up a basic CNN with one convolutional layer, one pooling layer, and an input layer. You can expand this example to create more complex neural networks for image or speech recognition tasks.
In summary, implementing Convolutional Neural Networks in Java using GeeksforGeeks' tutorials and examples involves installing necessary libraries like Weka or Deeplearning4j, setting up your project structure, and defining the CNN configuration. This example demonstrates a simple implementation of a CNN using DLP4j.
What is cnn in java with example
I'd be happy to explain CNN (Convolutional Neural Network) in Java with an example!
A Convolutional Neural Network (CNN) is a type of feedforward neural network that is commonly used for image classification and object detection tasks. It's particularly useful for processing data with spatial hierarchies, like images or videos.
Here's a high-level overview of how CNNs work:
Convolutional Layers: Each input image is convolved with learnable filters, which slide over the image in a specific pattern (e.g., horizontally and vertically). This creates feature maps that capture local patterns. Activation Functions: The output from the convolutional layers is then passed through an activation function, like ReLU (Rectified Linear Unit) or Sigmoid, to introduce non-linearity. Pooling Layers: After the convolutional layer, a pooling layer reduces spatial dimensions by taking max or average values over small regions of the feature maps. Flatten Layers: The output from the pooling layers is then flattened into one dimension for feeding into fully connected (dense) neural networks.Here's an example implementation in Java using Weka (Waikato Environment for Knowledge Analysis), a popular open-source library:
import weka.core.*;
import weka.classifiers.Evaluation;
import weka.classifiers.functions.ConvolutionalNetwork;
// Define the CNN architecture
int[] convolutionalLayers = {3, 5};
int[] poolingLayers = {2, 2};
// Create the CNN classifier
ConvolutionalNetwork cnn = new ConvolutionalNetwork();
// Define the number of neurons in each layer
int[] hiddenLayerSizes = {100, 50};
// Add convolutional and pooling layers to the network
for (int i = 0; i < convolutionalLayers.length; i++) {
cnn.addConvolutionalLayer(convolutionalLayers[i]);
if (i < poolingLayers.length) {
cnn.addPoolingLayer(poolingLayers[i], Pooling.Modes.MAX);
}
}
// Add fully connected layers to the network
for (int i = 0; i < hiddenLayerSizes.length - 1; i++) {
cnn.addFullyConnectedLayer(hiddenLayerSizes[i]);
}
cnn.addOutputLayer();
// Define the output layer size
cnn.setOutputs(10);
// Train the CNN on a dataset
Instances instances = // Load your training data
cnn.buildClassifier(instances);
Evaluation evaluation = new Evaluation(instances);
evaluation.evaluateModel(cnn, instances);
// Use the trained model for predictions
Prediction(predictions) = cnn.classifyInstance(new Instance());
Keep in mind that this is a simplified example and not production-ready. For more information on implementing CNNs in Java using Weka or other libraries, I recommend checking out online resources like tutorials, documentation, and research papers.
Would you like me to elaborate on any specific aspects of the code or provide additional details?