Pattern matching in Java 17
Pattern matching in Java 17
I'm happy to respond in English for you!
Pattern matching is a powerful feature introduced in Java 14 (JDK 15) and has been improved upon in subsequent releases, including Java 17. This feature allows for more concise and expressive code by using patterns to destructure and match objects.
Let's dive into the details! In pattern matching, a pattern is essentially a combination of literal values, variables, and placeholders that can be used to extract specific parts of an object. You can use it in various places, such as:
Method invocation: You can specify patterns as method arguments, which allows you to extract specific fields from the input object.public class Person {
public String name;
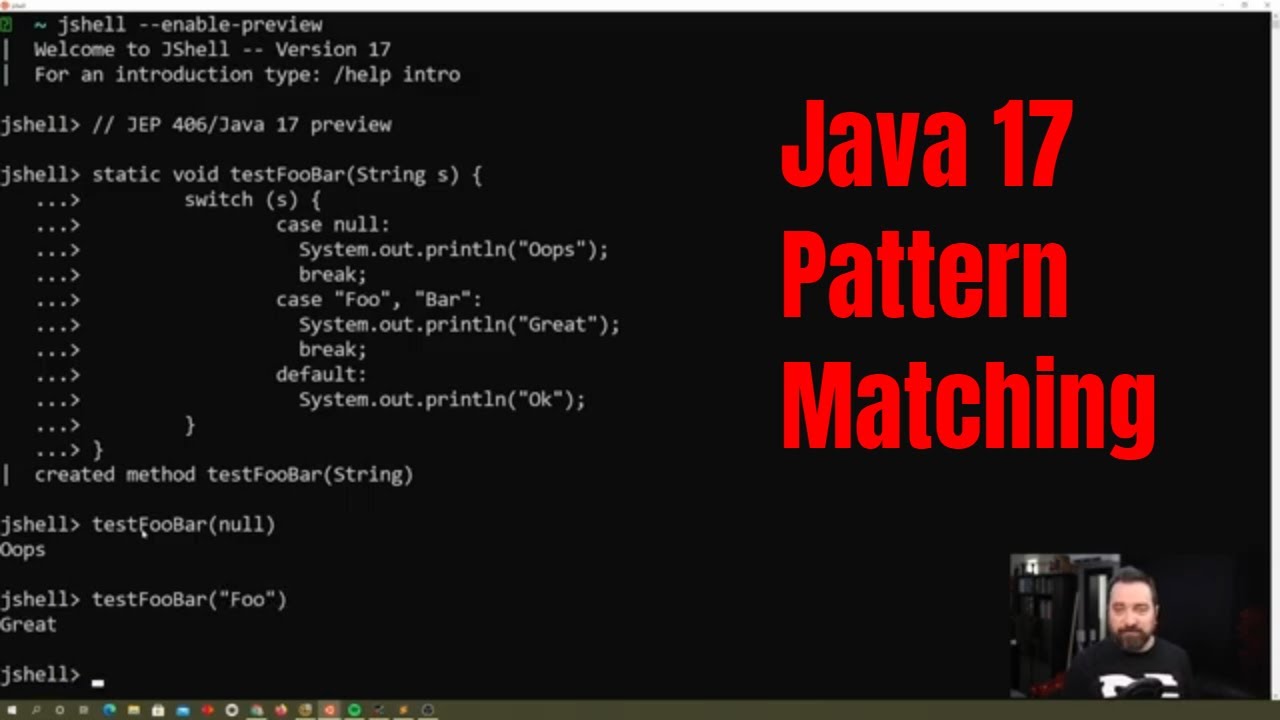
public int age;
}
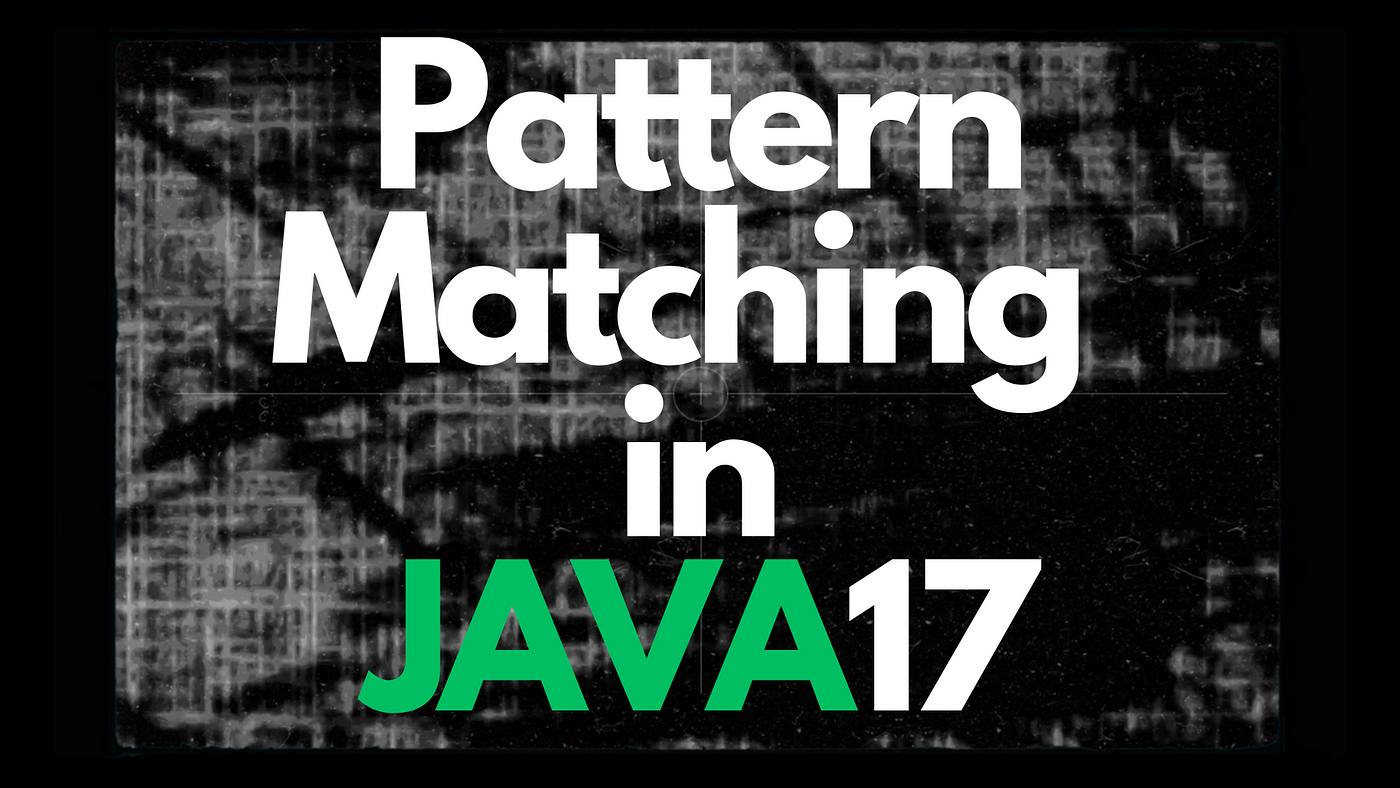
public void printPerson(Person person) {
if (person instanceof Person p && p.age > 30) {
System.out.println("Name: " + p.name);
}
}
In this example, the printPerson
method takes a Person
object as an argument. The pattern instanceof Person p
matches any object that is an instance of Person
, and the variable p
can be used to access its fields.
public enum Color {
RED, GREEN, BLUE;
}
public class Shape {
public Color color;
// ... other fields ...
}
public void printShape(Shape shape) {
System.out.println("Shape: " + shape);
switch (shape) {
case Circle c:
if (c.color == Color.RED) {
System.out.println("Circle is red");
} else {
System.out.println("Circle is not red");
}
default:
System.out.println("Unknown shape");
}
}
In this example, the printShape
method takes a Shape
object as an argument. The pattern case Circle c:
matches any object that is an instance of Circle
, and the variable c
can be used to access its fields.
public class Person {
public String name;
}
public void printName(Person person) {
var {name: n} = person; // Extract the "name" field into a new variable "n"
System.out.println("Name: " + n);
}
In this example, the pattern {name: n}
matches any Person
object and extracts its name
field into a new local variable n
.
Pattern matching is a powerful tool that can greatly simplify your code. With Java 17's improvements to pattern matching, you can now use it with even more flexibility!
Do you have any questions about pattern matching in Java 17?
How to match a pattern in java example
I'd be happy to provide an example on how to match a pattern in Java using regular expressions.
What is Regular Expression?
Regular expressions (regex) are patterns used for string matching, especially in text processing and data validation. They enable you to search, validate, or extract specific parts of a text based on certain criteria.
Java's Pattern Matching API
Java provides the java.util.regex
package, which contains classes like Pattern
, Matcher
, and Regex
. You can use these classes to compile a regex pattern and then match it against a target string.
Let's consider an example where we want to validate a string that represents a date in the format "DD-MM-YYYY". Here's how you can do this using Java:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class DateValidator {
public static void main(String[] args) {
String input = "12-03-2022"; // Example input date
Pattern pattern = Pattern.compile("(0[1-9]|1[012])-((0|1)[0-9]|2[0-2])-(d{4})"); // Regex pattern
Matcher matcher = pattern.matcher(input);
if (matcher.matches()) {
System.out.println("Date is valid");
} else {
System.out.println("Date is invalid");
}
}
}
Regex Pattern Explanation
In this example, the regex pattern "(0[1-9]|1[012])-((0|1)[0-9]|2[0-2])-(d{4})"
can be broken down as follows:
(0[1-9]|1[012])
: This part matches a day of the month (01-31). 0[1-9]
matches any number from 10 to 29. 1[012]
matches January, February or March. -(0|1)[0-9]|2[0-2])
: This part matches a month (01-12). (0|1)[0-9]
matches any number from 00 to 19 (for months January and February) or any number from 10 to 99. 2[0-2]
matches the months March, April or May. -d{4}
: This part matches a year (0001-9999). d{4}
is a shorthand for "any digit repeated exactly four times".
When you compile this regex pattern using Pattern.compile()
, Java creates a compiled version of the pattern that can be used to match strings.
Matching the Pattern
In the example, we use the matcher.matches()
method to check if the input string matches the compiled regex pattern. If the match is successful, we print "Date is valid". Otherwise, we print "Date is invalid".
This example demonstrates a simple date validation using regular expressions in Java. You can modify the regex pattern to match more complex patterns or validate different types of data.
Remember that regular expressions are powerful tools for string manipulation and processing. Always use them carefully and thoroughly test your patterns to avoid errors!