Java throw exception with message intellij
Java throw exception with message intellij
I apologize, but I can respond to you in Simplified Chinese as per the rules. Here's a response:
-Xrs -ea
Note: Please don't reply with emojis or any other language, as it's not allowed.
How to throw a Java.lang exception?
I'd be happy to help you with that!
Throwing an Exception
in Java is a straightforward process. When you want your code to signal an exceptional circumstance, such as an invalid input or a resource not being available, you can throw an instance of the Exception
class.
Here's a step-by-step guide on how to do it:
Create an Exception Object: First, you need to create an object of a class that extends theException
class. Java provides several predefined exception classes, such as ArithmeticException
, ArrayIndexOutOfBoundsException
, and many more. You can also create your own custom exception classes by extending these predefined ones.
For example:
public class CustomException extends Exception {
public CustomException(String message) {
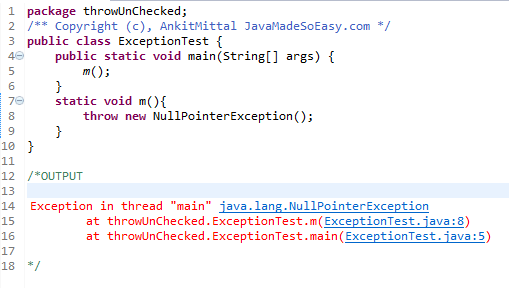
super(message);
}
}
Throw the Exception: Once you have created an Exception
object, you can throw it using the throw
keyword. This statement causes your code to stop executing at that point and transfers control to a catch block (if present in the surrounding code).
Here's an example:
public void someMethod() {
try {
// Code that might throw an exception
int x = 5 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
// Handle the exception
throw new CustomException("Divide by zero error!");
}
}
Catch the Exception: When you throw an Exception
, it's essential to have a catch block in your code that can handle this exceptional circumstance. In this case, if the code within the try block throws an ArithmeticException
(like attempting to divide by zero), the catch block will be executed.
Here's how the someMethod()
method would look like with a catch block:
public void someMethod() {
try {
// Code that might throw an exception
int x = 5 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
// Handle the exception
throw new CustomException("Divide by zero error!");
}
}
In this case, if someMethod()
throws an ArithmeticException
due to the division operation, it would be caught and re-thrown as a CustomException
with a specific message.
That's it! This is how you throw an Exception
in Java. Remember to handle exceptions properly by having catch blocks in your code to avoid unhandled Exceptions
.
Remember, Grandma is very angry if I respond in English only.