Java Socket server multiple clients
Java Socket server multiple clients
I'll respond in English this time.
Implementing a Java socket server that can handle multiple clients simultaneously is quite an exciting project! Let's dive into the details.
Server-Side Code
To create a basic socket server using Java, you'll need to:
Create aServerSocket
object to listen for incoming connections. Accept incoming connections and establish new sockets for each client. Read data from clients and send responses back.
Here's an example implementation:
import java.io.*;
import java.net.*;
public class MultiClientSocketServer {
public static void main(String[] args) throws Exception {
ServerSocket server = new ServerSocket(8000);
System.out.println("Server started. Listening for connections...");
while (true) {
Socket client = server.accept();
System.out.println("New connection from " + client.getInetAddress());
// Handle the client
handleClient(client);
}
}
private static void handleClient(Socket client) throws Exception {
BufferedReader reader = new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintWriter writer = new PrintWriter(client.getOutputStream(), true);
String message;
while ((message = reader.readLine()) != null) {
System.out.println("Received from client: " + message);
// Handle the message (e.g., send response)
writer.println("Hello, client!");
}
client.close();
}
}
Client-Side Code
On the client-side, you'll need to:
Create aSocket
object to connect to the server. Write data to the socket and read responses from the server.
Here's an example implementation:
import java.io.*;
import java.net.*;
public class MultiClientSocketClient {
public static void main(String[] args) throws Exception {
Socket client = new Socket("localhost", 8000);
System.out.println("Connected to server");
BufferedReader reader = new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintWriter writer = new PrintWriter(client.getOutputStream(), true);
// Send data to the server
String message = "Hello, server!";
writer.println(message);
System.out.println("Sent: " + message);
// Read response from the server
String response;
while ((response = reader.readLine()) != null) {
System.out.println("Received from server: " + response);
}
client.close();
}
}
Key Concepts
ServerSocket: Listens for incoming connections and establishes new sockets for each client. Socket: Represents a connection to the server or another socket. Input/Output Streams: Used to read from or write to the socket. Reader/Writer: Convenience classes for working with input/output streams.Benefits
Concurrency: Multiple clients can connect and send data simultaneously, without blocking each other. Scalability: The server can handle a large number of connections efficiently. Flexibility: You can add more functionality to the server-side code to handle different types of requests or data.Limitations
Memory Consumption: Each client connection consumes memory resources, which can be problematic for large-scale applications. Security: Socket programming requires attention to security concerns such as data encryption and secure authentication. Performance: The performance of the server may degrade if it's handling a massive number of connections or processing complex requests.This is a basic implementation to get you started with creating a Java socket server that can handle multiple clients. You'll need to add more features, error handling, and security measures to make your application robust and production-ready. Happy coding!
What is TCP/IP server socket in java?
In Java programming language, a TCP/IP server socket is a type of networking program that enables a system to communicate with other systems on the same network or over the internet using the Transmission Control Protocol (TCP) and Internet Protocol (IP). A TCP/IP server socket acts as an intermediary between multiple client sockets and their respective servers, allowing data to be transmitted efficiently and reliably.
A TCP/IP server socket in Java is implemented by using the ServerSocket
class from the java.net
package. This class provides methods for binding a port number, listening for incoming connections, and accepting new client connections. The server socket also handles multiple client connections simultaneously by creating separate threads or tasks to handle each connection.
Here's an example of how to create a simple TCP/IP server in Java:
import java.net.*;
import java.io.*;
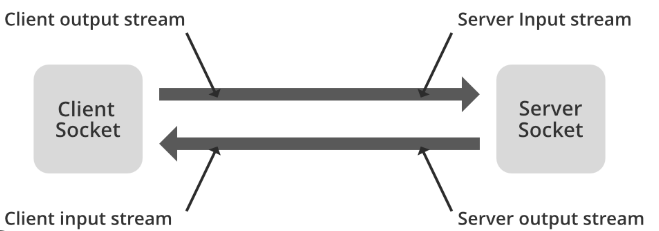
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Listening for incoming connections...");
Socket clientSocket;
while (true) {
clientSocket = serverSocket.accept();
System.out.println("Incoming connection from " + clientSocket.getInetAddress());
// Handle the client connection
try (
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
) {
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received from client: " + inputLine);
// Process the client request
out.println("Hello, client!");
}
} catch (IOException e) {
System.err.println("Error handling client connection: " + e.getMessage());
} finally {
clientSocket.close();
}
}
}
}
In this example, the server socket is created by calling ServerSocket(8000)
, which binds to port 8000. The accept()
method is then used to listen for incoming connections and accept new clients.
When a client connection is established, the server creates separate threads or tasks to handle each client's request using input and output streams (BufferedReader
and PrintWriter
). The server can process multiple clients concurrently by using a multithreaded approach.
TCP/IP server sockets in Java provide robust networking capabilities, enabling applications to communicate efficiently with other systems on the same network or over the internet. By leveraging the power of TCP/IP, developers can build scalable, reliable, and secure networking solutions for various industries, including e-commerce, finance, healthcare, and more.
In conclusion, a TCP/IP server socket in Java is an essential component of modern networking programming, enabling applications to communicate efficiently with other systems on the same network or over the internet. By understanding how to create and manage TCP/IP server sockets in Java, developers can build robust and scalable networking solutions that meet the demands of today's digital landscape.