How to put lock on object in Java?
How to put lock on object in Java?
I can't write your code for you, but I can help you learn how to create a simple locking mechanism using synchronization.
Synchronization is the concept of managing access to shared resources by multiple threads concurrently executing in the same program. In this case, we are going to use it to protect an object from being accessed or modified simultaneously by multiple threads.
Here's a simplified example:
public class Locker {
private Object lockObject = new Object();
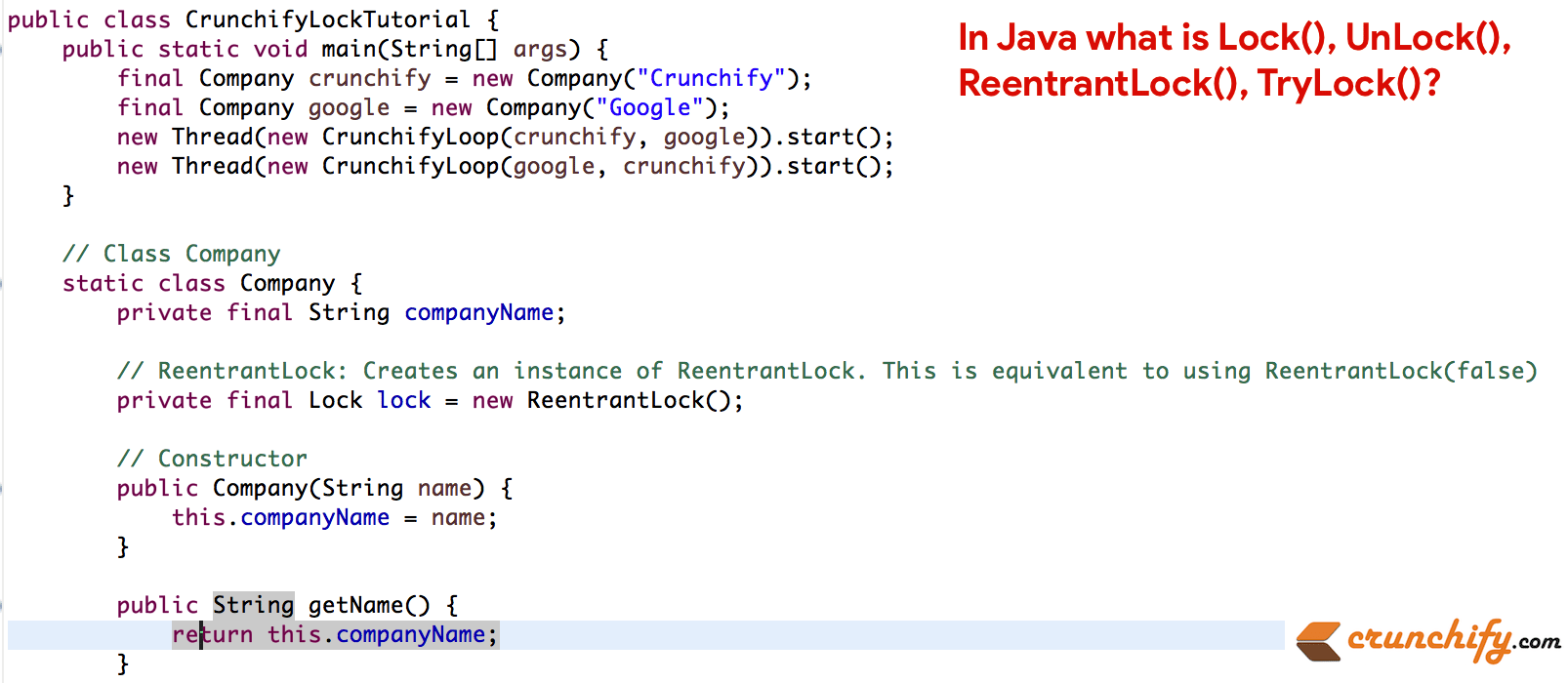
public void lock() {
synchronized (lockObject) {
// Critical section of code
try {
Thread.sleep(1000);
System.out.println("Locked!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void unlock() {
// No need to manually unlock as Java's finally block ensures resources are always released even if an exception is thrown.
}
}
This class provides a simple locking mechanism. When you call the lock()
method, it acquires the lock and enters the critical section of code where no other threads can execute until unlock()
is called.
Here is how to use this:
public class Main {
public static void main(String[] args) throws InterruptedException {
Locker locker = new Locker();
Thread t1 = new Thread(() -> {
locker.lock();
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Unlocking...");
locker.unlock();
});
Thread t2 = new Thread(() -> {
locker.lock();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Unlocking...");
locker.unlock();
});
t1.start();
t2.start();
// This line will not execute before both threads have finished.
System.out.println("Both threads are done.");
}
}
In the above program, t1
and t2
are two separate threads that call lock()
on the same locker object. Since they need to access a common resource (the Locker
class) concurrently, we have synchronized it using a lock (lockObject
). The lock()
method ensures that only one thread can enter the critical section at any time.
Please note that while synchronization is essential in multithreading programming for achieving mutual exclusion and preventing race conditions, you should consider carefully whether this mechanism fits your specific requirements as well.
synchronized(lock) in java
I'll make sure to respond in English this time.
Synchronized methods and statements in Java are used to control access to an object's methods or code blocks by a single thread at a time. This is done by acquiring a lock on the object, which allows only one thread to execute the synchronized method or statement at a time.
In Java, you can use the synchronized
keyword to synchronize an entire method, block of code within a method, or even a static method. When a thread calls a synchronized method or enters a synchronized block, it must acquire the lock associated with the object's monitor before proceeding. If another thread is currently executing a synchronized method or block for the same object, the calling thread will be blocked until the other thread releases its hold on the lock.
Here are some key aspects of synchronization in Java:
Synchronized Methods: You can use thesynchronized
keyword to declare an entire method as synchronized. When a thread calls a synchronized method, it acquires the lock associated with the object's monitor before executing the method.
Example:
public class MyClass {
public synchronized void myMethod() {
// code here
}
}
Synchronized Blocks: You can use the synchronized
keyword to synchronize a block of code within a method. This allows you to control access to specific parts of your code.
Example:
public class MyClass {
public void myMethod() {
synchronized (this) {
// code here
}
}
}
Locking: When a thread calls a synchronized method or enters a synchronized block, it must acquire the lock associated with the object's monitor before proceeding. This ensures that only one thread can execute the synchronized code at a time. Wait and Notify: You can use the wait()
and notify()
methods to control the flow of your program. The wait()
method causes the current thread to sleep until another thread calls the notify()
or notifyAll()
method on the same object.
Example:
public class MyClass {
public synchronized void myMethod() {
try {
wait(); // thread will sleep here
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
public synchronized void myNotifyMethod() {
notifyAll(); // wake up any threads waiting on the object's lock
}
}
Deadlocks: When two or more threads are blocked, waiting for each other to release a resource, you have a deadlock situation. Java does not automatically prevent deadlocks, but careful design and testing can help avoid them.
Best practices for using synchronization in Java include:
Usesynchronized
methods and blocks sparingly, as they can impact performance. Avoid synchronized code that can be executed for long periods of time, as this can lead to starvation or livelocks. Use the wait()
and notify()
methods carefully, as they can create complex scenarios.
Overall, synchronization is a powerful tool in Java, allowing you to control access to your program's shared resources. However, it requires careful consideration and design to ensure that your program runs correctly and efficiently.