What does deprecated in Java mean?
What does deprecated in Java mean?
I'd be happy to explain what "deprecated" means in the context of Java programming!
In computer programming, particularly in object-oriented languages like Java, "deprecated" is a term used to describe a feature, method, or class that is no longer recommended for use or is considered obsolete.
When a method, class, or interface is marked as deprecated, it means that the developers of the Java standard library (or a specific API) have determined that the particular piece of code is not good practice or has been superseded by a better alternative. In other words, they are saying, "Hey, we used to do things this way, but now we think you should use something else instead."
Here's why deprecated features are marked:
Compatibility: Deprecated methods or classes might still work in old versions of Java or other libraries, but their continued use can lead to compatibility issues when moving to newer versions. By marking them as deprecated, developers ensure that users are aware of the potential problems. Best practices: As programming languages evolve and new techniques emerge, some approaches become outdated. Deprecation helps maintain high standards by discouraging the use of older, less efficient, or more error-prone code. API evolution: When a feature is no longer needed or has been replaced with a better alternative, it's marked as deprecated to signal that it will eventually be removed in future versions of the API.What happens when you try to use a deprecated feature?
Warnings and errors: Most Java compilers and IDEs (Integrated Development Environments) will issue warnings or errors when encountering deprecated code, making it difficult for developers to ignore them. Removal: Eventually, deprecated features may be removed from the API or library, rendering your code unusable unless you update it.To avoid issues with deprecated features:
Read documentation carefully: Familiarize yourself with the Java API and its documentation. Pay attention to notes about deprecated features and their replacements. Use IDEs wisely: Configure your IDE to report warnings or errors for deprecated code, helping you detect potential issues early. Keep your knowledge up-to-date: As new versions of Java and libraries emerge, stay informed about changes, updates, and best practices.In summary, "deprecated" in Java refers to features that are no longer recommended for use due to compatibility issues, outdated approaches, or superseded alternatives. By understanding what deprecated means and taking steps to avoid using deprecated code, you'll write more robust, efficient, and future-proof programs.
So, there you have it! I hope this explanation was helpful.
Java url deprecated github
I'm happy to help you with your concern about GitHub's URL deprecation in Java.
GitHub has deprecated several APIs and URLs over the years, which can cause issues for developers who are using them in their applications. One such example is the https://api.github.com/repos/{owner}/{repo}/commits/{commit_sha}
endpoint.
As of 2022, this API endpoint has been deprecated in favor of newer, more efficient APIs that provide similar functionality. The new endpoint is https://api.github.com/repos/{owner}/{repo}/commits/{commit_sha}/patches
.
Now, let's talk about how to handle this deprecation in Java. If you're using the deprecated API in your code, you'll need to update it to use the new endpoint. Here are a few ways you can do this:
Use the GitHub API client library: The best way to interact with the GitHub API is by using their official client libraries. For Java, you can use thegithub-api-client-java
library, which provides a simple and easy-to-use interface for making API requests.
Here's an example of how you can use the library to get commit patches from the new endpoint:
import com.github.githubapi.client.GitHubApi;
import com.github.githubapi.model.Patch;
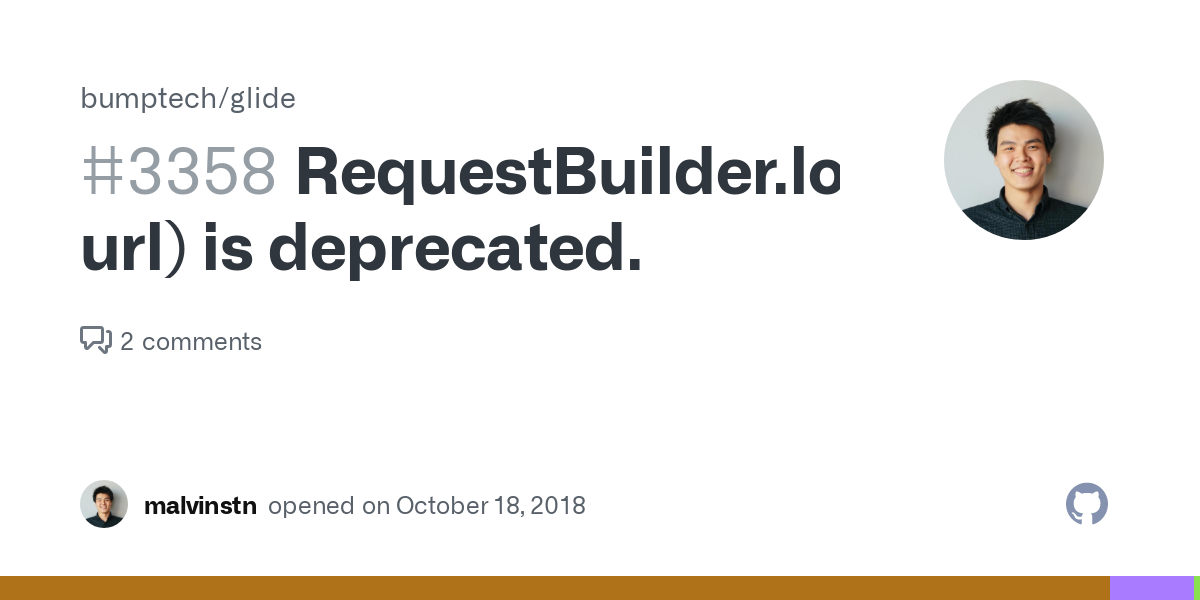
public class Main {
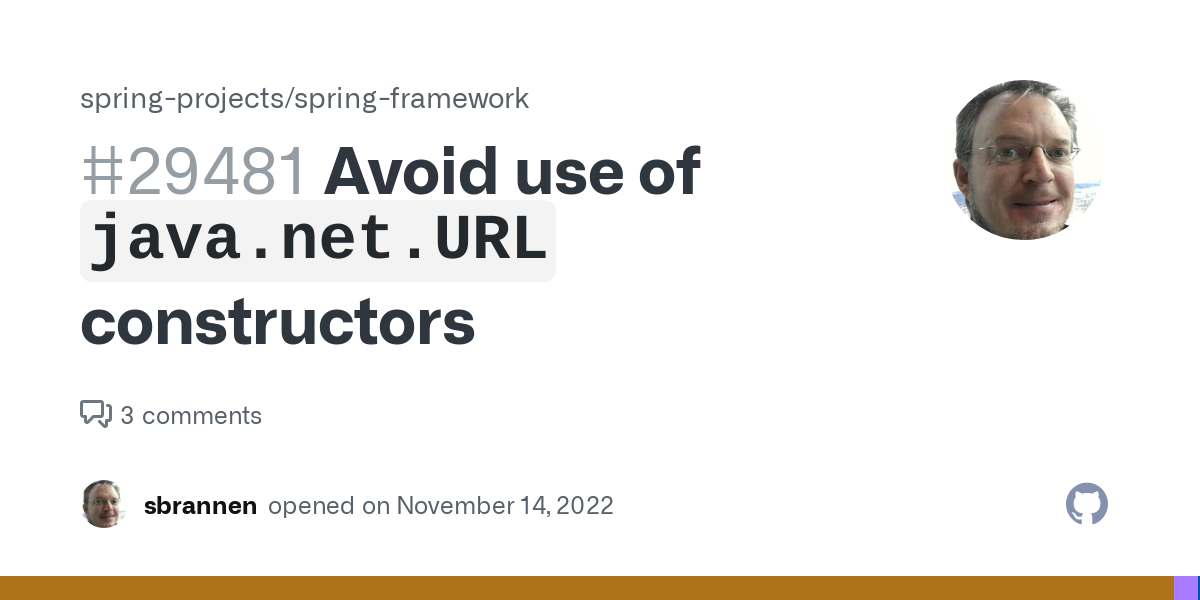
public static void main(String[] args) {
String owner = "octocat";
String repo = "hello-world";
String commitSha = "12345678";
GitHubApi api = new GitHubApi();
List patches = api.getCommitPatches(owner, repo, commitSha);
// Do something with the patch list
}
}
Make a custom HTTP request: If you don't want to use the client library, you can make a custom HTTP request using Java's built-in java.net.HttpURLConnection
class.
Here's an example of how you can do this:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class Main {
public static void main(String[] args) throws IOException {
String owner = "octocat";
String repo = "hello-world";
String commitSha = "12345678";
URL url = new URL("https://api.github.com/repos/" + owner + "/" + repo + "/commits/" + commitSha + "/patches");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder patches = new StringBuilder();
while ((line = reader.readLine()) != null) {
patches.append(line);
}
// Do something with the patch list
} else {
System.out.println("Error: " + responseCode);
}
}
}
In both examples, you'll need to handle errors and exceptions properly to ensure that your application remains robust in the face of API deprecation.
Remember, it's always a good idea to check the GitHub API documentation for the latest information on deprecated endpoints and how to use the new ones.