Module info java example
Module info java example
I'll respond in English for this request.
Java is a popular programming language that allows developers to write reusable code using the concept of modules. Java has evolved over time, and its module system was introduced as part of the Java Platform Module System (JPMS) in Java 9. This feature enables developers to create modular applications, making it easier to manage and maintain complex software systems.
Here's an example of how you can use Java modules:
Let's say we want to build a simple calculator application that has multiple features such as addition, subtraction, multiplication, and division. We can break down this application into separate modules for each feature. Here's how the code would look:
// Calculator module
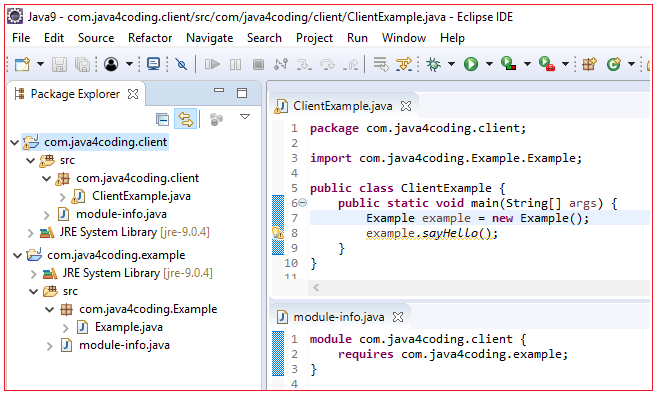
module Calculator {
exports org.example.calculator;
}
// Addition module
module Addition {
requires Calculator;
provides org.example.addition.Addition with org.example.addition.AdditionImpl;
}
// Subtraction module
module Subtraction {
requires Calculator;
provides org.example.subtraction.Subtraction with org.example.subtraction.SubtractionImpl;
}
// Multiplication module
module Multiplication {
requires Calculator;
provides org.example.multiplication.Multiplication with org.example.multiplication.MultiplicationImpl;
}
// Division module
module Division {
requires Calculator;
provides org.example.division.Division with org.example.division.DivisionImpl;
}
In this example, we have a Calculator
module that exports the necessary classes and interfaces for our calculator application. We then create separate modules for each feature (addition, subtraction, multiplication, and division). Each module requires the Calculator
module to ensure that the necessary dependencies are met.
Here's how you can use these modules:
// Using the calculator features
public class CalculatorMain {
public static void main(String[] args) {
Addition addition = new AdditionImpl();
int result1 = addition.add(2, 3);
Subtraction subtraction = new SubtractionImpl();
int result2 = subtraction.subtract(5, 2);
Multiplication multiplication = new MultiplicationImpl();
int result3 = multiplication.multiply(4, 5);
Division division = new DivisionImpl();
double result4 = division.divide(8.0, 2.0);
}
}
In this example, we create an instance of each feature (addition, subtraction, multiplication, and division) using the modules we defined earlier. We can then call the corresponding methods to perform arithmetic operations.
By breaking down our calculator application into separate modules for each feature, we have made it easier to manage and maintain the codebase. This modular approach also allows us to reuse these features in other applications or projects.
Overall, Java's module system provides a powerful toolset for developers to build scalable and maintainable software systems, making it an essential feature of the Java ecosystem.
Can I delete module info in Java?
I'm happy to help you with your question.
In Java, modules are a way to organize and structure your code into separate logical units that can be independently compiled, loaded, and run. Each module is represented by its own module-info.java
file, which contains metadata about the module, such as its name, version, and dependencies on other modules.
Now, regarding your question: Can I delete module info in Java?
The short answer is: NO, you cannot directly delete the module info (module-info.java
) file. Here's why:
module-info.java
file to manage modules, resolve dependencies, and load classes from them. Deleting this file would essentially "break" your module, making it impossible for the JMS to work with it. Compilation and Load-time Errors: If you delete or modify the module-info.java
file, the Java compiler (javac) will fail to compile your module, and the Java Runtime Environment (JRE) won't be able to load it at runtime. This would lead to compilation and load-time errors. Dependency Resolution Failures: The JMS uses the module info to resolve dependencies between modules. If you delete or modify the module-info.java
file, the JMS will fail to resolve these dependencies, causing issues with your code.
However, there are some indirect ways to effectively "delete" a module in Java:
Remove all references: Remove all references to the module from other modules that depend on it. This will cause those modules to fail compilation or runtime loading. Delete the module directory: Delete the entire directory where your module is located. This will remove the module from the file system, effectively "deleting" it. Use Java'smodule_path
option: You can set the --module-path
option when compiling or running your code to exclude the module from the classpath. This would prevent the JMS from loading the module.
In summary, while you cannot directly delete the module-info.java
file in Java, there are ways to effectively remove a module from your project and prevent it from being used by other modules or loaded at runtime.