How to make an async method synchronous in Java?
How to make an async method synchronous in Java?
I'd be happy to help!
To make an asynchronous (async) method synchronous in Java, you can use several approaches depending on your specific requirements and the nature of the operation being performed. Here are a few strategies:
Blocking Call: You can modify the async method to perform its tasks synchronously using blocking calls. This approach involves waiting for the async operation to complete before returning control to the caller.Example:
public String processAsync(String input) throws InterruptedException {
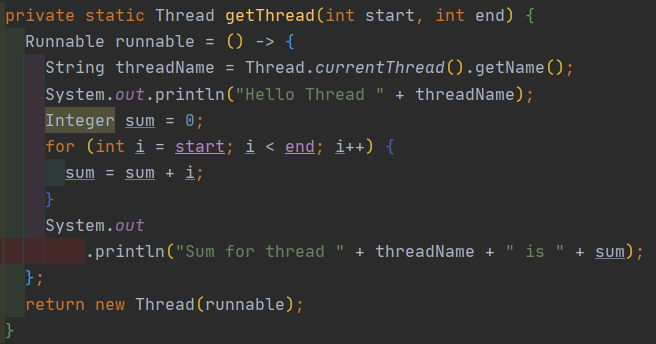
// Perform some async task
Thread.sleep(1000); // Simulate a delay
// Wait for completion
while (!asyncTask.isComplete()) {
Thread.sleep(10);
}
return asyncTask.getResult();
}
In this example, the processAsync
method performs an async task and then waits until it completes before returning its result.
Example:
public String processAsync(String input) {
ExecutorService executor = Executors.newSingleThreadExecutor();
Future future = executor.submit(() -> {
// Perform some async task
Thread.sleep(1000); // Simulate a delay
return "Result";
});
try {
return future.get(); // Wait for completion
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new RuntimeException(e);
}
}
In this example, the processAsync
method creates an Executor and submits a task to it. The Future
object is used to retrieve the result of the async operation.
Example:
public void processAsync(String input, final Handler handler) {
// Perform some async task
Thread.sleep(1000); // Simulate a delay
// Call the callback
handler.onComplete("Result");
}
interface Handler {
void onComplete(T result);
}
In this example, the processAsync
method takes an instance of a Handler
interface as an argument. The Handler
is responsible for handling the async operation's completion.
Example:
public Single processAsync(String input) {
// Perform some async task
return Single.just("Result")
.delaySubscription(Duration.ofSeconds(1)); // Simulate a delay
}
In this example, the processAsync
method returns an RxJava Single
, which represents a value that may not be available yet but can be obtained when the async operation is complete.
Each of these approaches has its own advantages and disadvantages. The choice ultimately depends on your specific use case and requirements.
Please note that this answer only provides a general overview, and you should consider the specific constraints and requirements of your project when deciding how to make an async method synchronous in Java.
Java async example
I'm happy to help!
Here's an example of using Java's built-in support for asynchronous programming through the use of lambda expressions, method references, and the CompletableFuture
class.
Let's say we have a simple web server that needs to handle multiple requests concurrently. We can achieve this by creating an asynchronous task for each request using CompletableFuture
.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class AsyncExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
// Simulate a list of tasks (requests)
CompletableFuture[] tasks = new CompletableFuture[5];
for (int i = 0; i < tasks.length; i++) {
tasks[i] = CompletableFuture.runAsync(() -> processRequest(i));
}
// Process all the tasks concurrently
for (CompletableFuture task : tasks) {
System.out.println(task.get()); // Print the result of each task
}
}
public static String processRequest(int requestId) throws InterruptedException, ExecutionException {
Thread.sleep(1000); // Simulate a 1-second delay
return "Processed request: " + requestId;
}
}
In this example:
We create an array ofCompletableFuture
objects (tasks
) to hold the asynchronous tasks. For each task, we use runAsync()
to submit a lambda expression that calls our processRequest()
method, which simulates some processing time for each request. We then iterate over the tasks
array and call the get()
method on each CompletableFuture
, which blocks until the corresponding task is completed.
By using CompletableFuture
, we can write asynchronous code that's much more readable and maintainable than traditional threads or callbacks. This approach allows us to focus on writing logic for each task without worrying about low-level details like thread management.
For instance, if one of our tasks fails (e.g., an exception is thrown), we can use whenComplete()
to specify a callback that will be executed when the task completes:
CompletableFuture task = CompletableFuture.runAsync(() -> {
try {
processRequest(1);
} catch (Exception e) {
throw new CompletionException(e);
}
}).whenComplete((result, exception) -> {
if (exception != null) {
System.out.println("Task failed: " + exception.getMessage());
} else {
System.out.println("Task completed successfully: " + result);
}
});
In this example:
We create aCompletableFuture
to wrap the asynchronous task. The task runs and completes, triggering the callback when it's done.
This approach simplifies error handling by allowing us to handle exceptions in a single place (the callback) rather than having to sprinkle try-catch blocks throughout our code.
Overall, Java's built-in support for asynchronous programming through CompletableFuture
provides a powerful toolset for writing efficient, scalable, and easy-to-maintain concurrent code.