Is iterator an interface in Java?
Is iterator an interface in Java?
I'll make sure to respond in English only!
To answer your question: No, Iterator is not an interface in Java.
In Java, the Iterator
is a concrete class that implements the Iterable
interface. The Iterable
interface provides methods for accessing the elements of an iterable collection, such as a list or array. An iterator allows you to traverse through a collection (like a List) and access its elements one at a time.
Here's a breakdown:
Iterable is an interface in Java that defines the methods necessary for traversing the elements of a collection.Iterator
is a concrete class that implements the Iterable
interface. It provides a way to iterate over the elements of a collection. An iterator can be used with any implementation of the Iterable
interface, such as ArrayList
, LinkedList
, Vector
, etc.
The reason for this design is that an Iterator
needs to maintain internal state information about where it is in the iteration process (e.g., its current position). This information cannot be encapsulated within the Iterable
interface alone. Hence, we need a concrete class like Iterator
that implements the Iterable
interface and provides the necessary iteration methods.
To illustrate this, consider the following example:
List list = new ArrayList<>();
list.add("Hello");
list.add("World");
// Create an Iterator for the List
Iterator iterator = list.iterator();
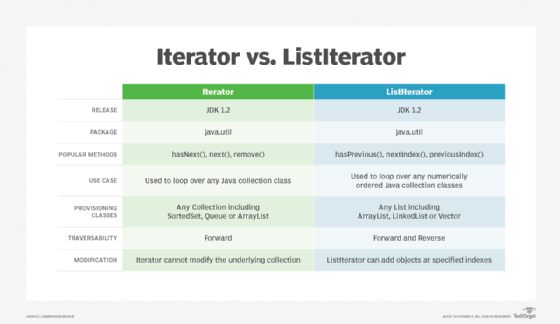
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
In this example:
List
is an implementation of the Iterable
interface. ArrayList
is a specific implementation of the List
interface. The iterator()
method returns an Iterator
object that can traverse the elements of the list.
So, to summarize: Iterator
is not an interface in Java; rather, it's a concrete class that implements the Iterable
interface and provides iteration methods for traversing collections.
java iterator remove
Java's Iterator interface provides a way to iterate over a collection of objects, such as a list, set, or map. The Iterator interface has several methods that allow you to traverse the elements of the collection.
One of the most commonly used methods in the Iterator interface is the remove()
method. This method removes the current element from the collection and moves all subsequent elements along one position.
Here's how it works:
First, you need to obtain an iterator object for a specific collection (such as a list or set). This can be done using theiterator()
method provided by the collection class. Once you have an iterator object, you can use its hasNext()
method to check if there are more elements in the collection that haven't been processed yet. If hasNext()
returns true, it means there's at least one element left in the collection. To remove an element from the collection, call the next()
method first to move to the current element, and then call the remove()
method.
Here's a simple example:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class IteratorExample {
public static void main(String[] args) {
// Create a list of numbers
List numbers = new ArrayList<>();
for (int i = 1; i <= 5; i++) {
numbers.add(i);
}
// Get an iterator object
Iterator iterator = numbers.iterator();
// Iterate over the collection and remove elements that are even
while (iterator.hasNext()) {
Integer num = iterator.next();
if (num % 2 == 0) {
iterator.remove(); // Remove the current element
}
}
System.out.println("Remaining numbers: " + numbers);
}
}
When you run this code, it will remove all even numbers from the list, resulting in a new list containing only odd numbers.
In terms of performance, removing elements from a collection while iterating over it can be expensive. This is because the iterator's state must be updated after each removal operation. In some cases, you might want to use an Iterator
and a separate index variable to avoid this problem:
int index = 0;
while (index < numbers.size()) {
Integer num = numbers.get(index);
if (num % 2 == 0) {
numbers.remove(index); // Remove the element at the current index
} else {
index++;
}
}
This approach can be more efficient, but it's also less convenient because you need to manually handle the indexing.