Constructor getter and setter in Java example
Constructor getter and setter in Java example
Here is an example of constructor, getter, and setter methods in Java:
Let's say we have a simple class called Person
that has three attributes: name
, age
, and city
.
public class Person {
private String name;
private int age;
private String city;
// Constructor with default values
public Person() {
this.name = "Unknown";
this.age = 0;
this.city = "Nowhere";
}
// Constructor with parameterized initialization
public Person(String name, int age, String city) {
this.name = name;
this.age = age;
this.city = city;
}
// Getter methods
public String getName() {
return this.name;
}
public int getAge() {
return this.age;
}
public String getCity() {
return this.city;
}
// Setter methods
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
public void setCity(String city) {
this.city = city;
}
}
Now, let's create an instance of the Person
class using both constructors:
// Create a person with default values
Person person1 = new Person();
System.out.println("Name: " + person1.getName());
System.out.println("Age: " + person1.getAge());
System.out.println("City: " + person1.getCity());
// Create a person with parameterized initialization
Person person2 = new Person("John", 30, "New York");
System.out.println("nName: " + person2.getName());
System.out.println("Age: " + person2.getAge());
System.out.println("City: " + person2.getCity());
Output:
Name: Unknown
Age: 0
City: Nowhere
Name: John
Age: 30
City: New York
As you can see, the Person
class has two constructors. The first constructor initializes the attributes with default values (unknown name, age of 0, and city of "Nowhere"). The second constructor allows us to pass in custom values for the attributes.
The getter methods (getName()
, getAge()
, and getCity()
) allow us to retrieve the current values of the attributes. The setter methods (setName()
, setAge()
, and setCity()
) allow us to update the values of the attributes.
This is a basic example of how constructor, getter, and setter methods work in Java. These concepts are essential for creating reusable classes that can be easily customized and extended.
Java encapsulation w3schools tutorial
I'll provide an explanation of Java encapsulation using the W3Schools tutorial.
W3Schools defines encapsulation as a fundamental concept in object-oriented programming (OOP) that binds together the data and methods that manipulate the data, and that hides them from the outside world. In other words, encapsulation is about hiding the internal details of an object from the outside world and only providing access to it through a public interface.
Java supports encapsulation using classes. A class defines a blueprint for creating objects, which are instances of the class. The class consists of two main parts: variables (data) and methods (functions). In Java, these variables are called instance variables or data members.
The importance of encapsulation lies in its ability to control access to the internal state of an object. You can restrict access to certain parts of the object by making them private, which means they can only be accessed within the class itself.
Here is a simple example from W3Schools:
public class Person {
// Private data members
private String name;
private int age;
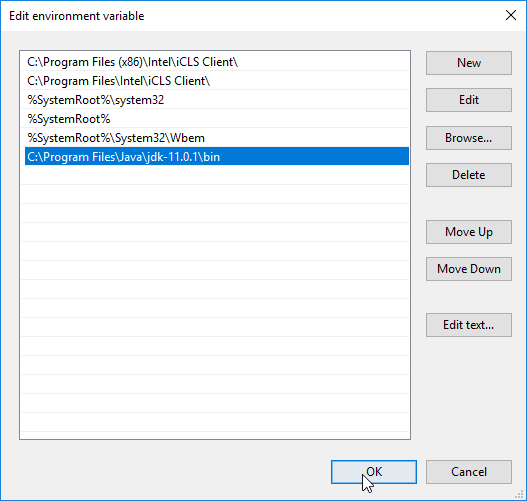
// Public constructor
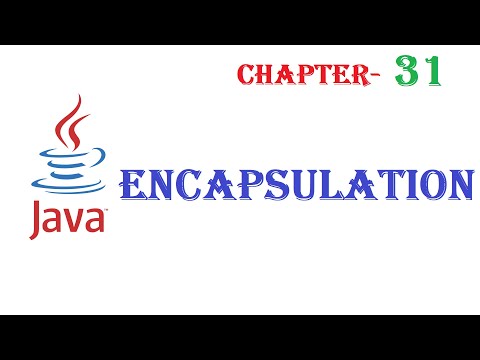
public Person(String n, int a) {
name = n;
age = a;
}
// Public method to get the name
public String getName() {
return name;
}
// Public method to get the age
public int getAge() {
return age;
}
}
In this example, the Person
class encapsulates its private variables (name
and age
) within the class itself. The constructor is used to set these private variables when an object of type Person
is created. The getter methods (getName()
and getAge()
) provide public access to these private variables.
W3Schools also explains that there are two types of encapsulation in Java: package-level encapsulation and instance-level encapsulation.
Package-level encapsulation refers to the way that classes within the same package can access each other's private members. In Java, a class has private access to its own members, but not those of another class, unless it is in the same package. Instance-level encapsulation refers to the way that objects of the same class can access their own private members. An object has private access to its own members and cannot access those of other objects.In conclusion, Java encapsulation is a fundamental concept that allows you to control access to an object's internal state by hiding it from the outside world and providing public interfaces for interaction. This helps in creating robust and maintainable software systems.