Java join multiple threads
Java join multiple threads
I'd be happy to help!
Java provides several ways to join multiple threads. Here are some common approaches:
Join() method: Thejoin()
method is a built-in method provided by the Thread
class in Java. It allows one thread to wait until another thread terminates. This can be useful when you want to ensure that one thread has completed its execution before moving on to the next step.
Here's an example:
public class JoinExample {
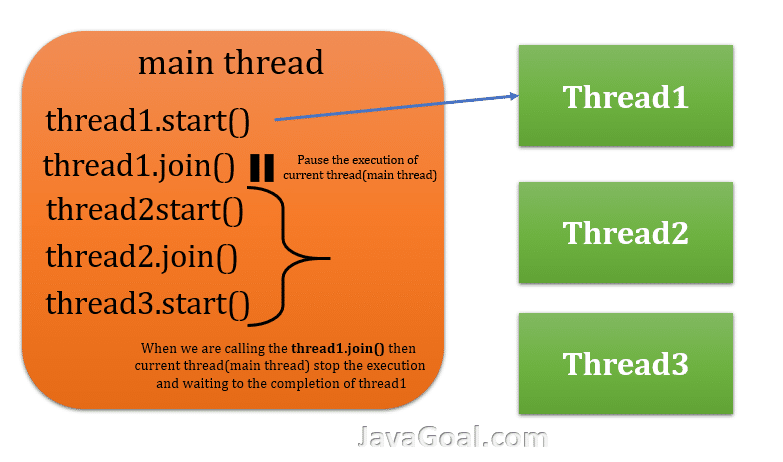
public static void main(String[] args) {
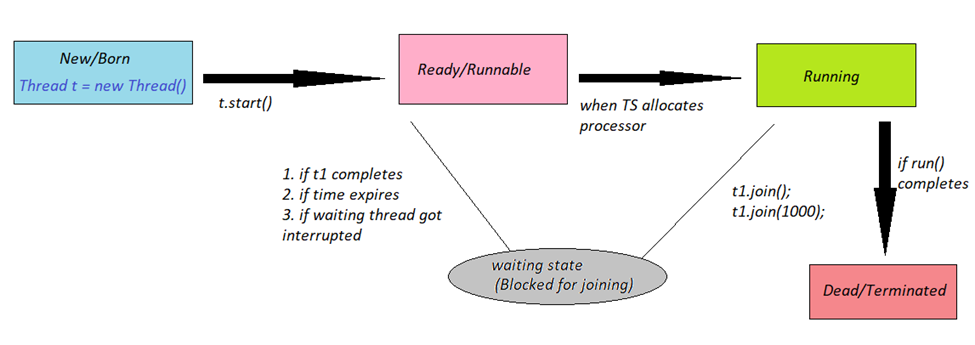
Thread t1 = new Thread(() -> {
for (int i = 0; i < 10; i++) {
System.out.println("Thread 1: " + i);
try {
Thread.sleep(1000); // Simulate some work
} catch (InterruptedException e) {
// Handle interrupt
}
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 10; i++) {
System.out.println("Thread 2: " + i);
try {
Thread.sleep(1000); // Simulate some work
} catch (InterruptedException e) {
// Handle interrupt
}
}
});
t1.start();
t2.start();
try {
t1.join(); // Wait for t1 to finish before proceeding
} catch (InterruptedException e) {
// Handle interrupt
}
System.out.println("Thread 1 finished");
}
}
In this example, the main thread waits until t1
finishes its execution using the join()
method. Then it prints a message indicating that t1
has finished.
ExecutorService
class in the java.util.concurrent
package, which allows you to manage multiple threads more efficiently. You can submit tasks to an ExecutorService
, and then use Future
s to get the results of those tasks.
Here's an example:
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
Future future1 = executor.submit(() -> {
for (int i = 0; i < 10; i++) {
System.out.println("Task 1: " + i);
try {
Thread.sleep(1000); // Simulate some work
} catch (InterruptedException e) {
// Handle interrupt
}
}
return 1;
});
Future future2 = executor.submit(() -> {
for (int i = 0; i < 10; i++) {
System.out.println("Task 2: " + i);
try {
Thread.sleep(1000); // Simulate some work
} catch (InterruptedException e) {
// Handle interrupt
}
}
return 2;
});
try {
int result1 = future1.get(); // Wait for task 1 to finish and get its result
int result2 = future2.get(); // Wait for task 2 to finish and get its result
System.out.println("Task 1 finished: " + result1);
System.out.println("Task 2 finished: " + result2);
} catch (InterruptedException | ExecutionException e) {
// Handle interrupt or execution exception
}
}
}
In this example, the main thread submits two tasks to an ExecutorService
, which manages a fixed-size thread pool. Then it uses Future
s to get the results of those tasks and print them.
ReentrantLock
, CountDownLatch
, and Semaphore
in the java.util.concurrent
package, which can be used to coordinate access to shared resources among multiple threads.
Here's an example using a CountDownLatch
:
import java.util.concurrent.CountDownLatch;
public class LatchExample {
public static void main(String[] args) {
final int NTHREADS = 5;
final CountDownLatch latch = new CountDownLatch(NTHREADS);
for (int i = 0; i < NTHREADS; i++) {
Thread t = new Thread(() -> {
try {
System.out.println("Thread " + i + " started");
Thread.sleep(1000); // Simulate some work
System.out.println("Thread " + i + " finished");
} catch (InterruptedException e) {
// Handle interrupt
}
});
t.start();
}
try {
latch.await(); // Wait until all threads have finished
} catch (InterruptedException e) {
// Handle interrupt
}
System.out.println("All threads finished");
}
}
In this example, the main thread creates NTHREADS
number of threads, and each thread prints a message indicating that it has started and finished. The main thread uses a CountDownLatch
to wait until all threads have finished before printing a final message.
These are just a few examples of how you can join multiple threads in Java. The choice of approach depends on the specific requirements of your application and the level of concurrency you need to achieve.
What is join() in Java thread?
I apologize for the confusion earlier. Here's a detailed explanation of the join()
method in Java threads:
In Java, the join()
method is used to make one thread wait until another thread has finished its execution or reached a certain state (like interrupted or dead). This method is part of the Thread
class and allows threads to synchronize with each other.
When you call join()
on a thread object, the current thread (this
) will block until the specified thread completes. The join()
method takes one parameter: the thread whose completion we are waiting for. If this thread is interrupted during the wait, the exception will be caught and rethrown after the join operation has completed.
Here's an example of how to use join()
:
Thread t = new Thread(() -> {
System.out.println("Starting child thread");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
t.start();
try {
t.join(); // wait for child thread to complete
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Child thread has completed");
In this example, the main thread starts a child thread and then waits for it to complete using join()
. The join()
method will block until the child thread has finished its execution.
The key benefits of using join()
are:
join()
allows threads to synchronize with each other, ensuring that one thread does not continue executing until another thread has completed. Order-of-execution guarantee: By using join()
, you can ensure that the order of execution between threads is as intended.
However, it's important to note that join()
can lead to:
join()
can create deadlocks if multiple threads are waiting for each other to complete.
To avoid these issues, consider using alternative synchronization mechanisms like synchronized
blocks, ReentrantLock
, or Semaphores
. These alternatives provide more fine-grained control over thread synchronization and can help prevent performance issues and deadlocks.
In conclusion, the join()
method is a powerful tool for synchronizing threads in Java. However, it should be used judiciously to avoid performance issues and deadlocks. With proper use, join()
can help ensure that your multi-threaded programs execute as intended.