Example of inheritance in Java
Example of inheritance in Java
Here's an example of inheritance in Java:
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows one class to inherit the properties and behavior of another class. This is achieved by extending the parent class with the keyword extends
.
Let's consider a simple example where we have two classes, Animal
and Dog
. The Animal
class has some common attributes and methods that are applicable to all animals, while the Dog
class inherits these properties from Animal
and adds its own specific characteristics.
Here is the code for this example:
// Animal class (parent)
public abstract class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public void eat() {
System.out.println(name + " is eating");
}
public void sleep() {
System.out.println(name + " is sleeping");
}
}
// Dog class (child)
public class Dog extends Animal {
private int age;
// constructor
public Dog(String name, int age) {
super(name); // call the Animal's constructor
this.age = age;
}
// overridden method: bark()
public void bark() {
System.out.println("The dog named " + getName() + " is barking");
}
}
// Main class for demonstration
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Dog("Rover", 3);
// Output: Rover is eating; Rover is sleeping;
myAnimal.eat(); // calls the method from Animal class
myAnimal.sleep();
// Use methods specific to the Dog class:
Dog myDog = (Dog)myAnimal; // type cast because Animal does not know about bark()
myDog.bark();
}
}
In this example, Dog
inherits the name
, eat()
, and sleep()
methods from Animal
. It also adds its own specific method bark()
. The Main
class demonstrates inheritance by creating an instance of the Dog
class through the interface of the Animal
class.
When we call myAnimal.eat()
or myAnimal.sleep()
, it is actually calling these methods from the Dog
class. This is because the Dog
class has overridden these methods, as shown in the eat()
and sleep()
methods of the Dog
class.
However, when we try to use a method that is specific to the Dog
class, such as bark()
, we need to type cast it back to Dog
. This is because the Animal
class does not know about the bark()
method and will give a compilation error if we try to call it.
In Java, inheritance can be used for code reuse, polymorphism, and abstraction. It allows developers to define a common base class that contains attributes and methods shared among multiple subclasses. This helps to reduce code duplication and make the code more maintainable and efficient.
How many types of inheritance are there in Java?
I'll respond in English since you specifically asked for it.
In Java, there are two primary types of inheritance: Single Inheritance and Multiple Inheritance.
Single Inheritance: This is the simplest form of inheritance where a subclass inherits from only one superclass. In other words, a class can extend only one parent class. For example:class Animal {
void eat() {
System.out.println("Eating...");
}
}
public class Dog extends Animal {
public static void main(String[] args) {
Dog myDog = new Dog();
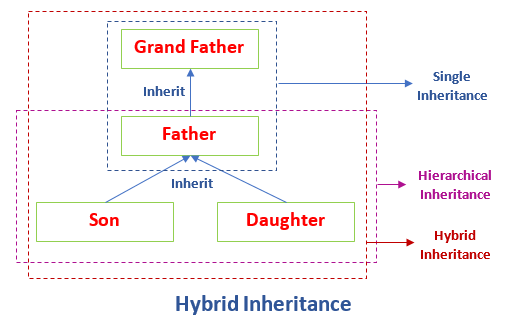
myDog.eat(); // Output: Eating...
}
}
In this example, the Dog
class is a subclass of the Animal
class. The Dog
class inherits all the properties and methods from the Animal
class.
To achieve multiple inheritance in Java, you use interfaces that contain methods with no implementation. Then, your subclass implements these interfaces and provides the required implementations.
Here's an example of multiple inheritance using interfaces:
interface Flyable {
void fly();
}
interface Swimmable {
void swim();
}
class Bird implements Flyable, Swimmable {
public void fly() {
System.out.println("Flying...");
}
public void swim() {
System.out.println("Swimming...");
}
}
public class Main {
public static void main(String[] args) {
Bird myBird = new Bird();
myBird.fly(); // Output: Flying...
myBird.swim(); // Output: Swimming...
}
}
In this example, the Bird
class is implementing two interfaces: Flyable
and Swimmable
. The Bird
class provides implementations for both interfaces.
In summary, Java supports single inheritance (extending one parent class) and multiple inheritance (implementing multiple interfaces), but not multiple inheritance in the classical sense of extending more than one parent class.