How to iterate a string array in JavaScript?
How to iterate a string array in JavaScript?
I'm happy to respond in English for you!
Iterating over a string array in JavaScript can be achieved using various methods, including for loops, Array.prototype.forEach(), and map().
Method 1: For Loop
const strArray = ['apple', 'banana', 'cherry'];
for (let i = 0; i < strArray.length; i++) {
console.log(strArray[i]);
}
This method involves using a traditional for loop to iterate over the elements of the string array. The loop starts at index 0 and continues until it reaches the last element in the array (determined by strArray.length
). Inside the loop, you can access each element by referencing its index (strArray[i]
).
Method 2: Array.prototype.forEach()
const strArray = ['apple', 'banana', 'cherry'];
strArray.forEach((element, index) => {
console.log(element);
});
Here, we're using the forEach()
method to iterate over the array. This method takes a callback function as an argument, which is called once for each element in the array. The callback function receives two arguments: the current element (element
) and its index (index
). You can access each element by referencing element
, just like with the for loop.
Method 3: Array.prototype.map()
const strArray = ['apple', 'banana', 'cherry'];
strArray.map((element, index) => {
console.log(element);
});
In this example, we're using the map()
method to create a new array by applying a transformation function to each element in the original array. The callback function receives two arguments: the current element (element
) and its index (index
). We can use this method when we want to perform some operation on each element of the array.
Method 4: Using ES6 for-of Loop
const strArray = ['apple', 'banana', 'cherry'];
for (const element of strArray) {
console.log(element);
}
This is a more concise and readable way to iterate over an array using the ES6 for-of
loop. The syntax is similar to the traditional for loop, but it's shorter and easier to understand.
All of these methods allow you to iterate over a string array in JavaScript, and you can choose the one that best fits your needs depending on the specific requirements of your code.
Hope this helps!
how to run java program in notepad++
Running a Java program in Notepad++ is a straightforward process that doesn't require any additional software or plugins. Here's a step-by-step guide on how to do it:
Step 1: Install and Set Up Notepad++
If you haven't already, download and install Notepad++ from its official website. Once installed, make sure to set up the editor to your liking by configuring the font, tab size, and other settings.
Step 2: Create a New Java File
Open Notepad++ and create a new file by clicking on "File" > "New" (or press Ctrl + N). Name your file with a .java extension, for example, "HelloWorld.java".
Step 3: Write Your Java Code
In the newly created file, start writing your Java code. For example, if you want to write a simple "Hello World" program, you can use the following code:
public class HelloWorld {
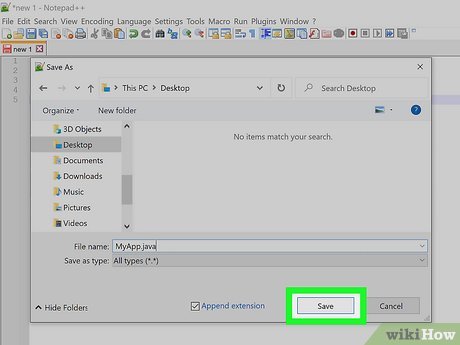
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Step 4: Compile Your Code
Notepad++ doesn't have a built-in compiler for Java. However, you can compile your code using the command line or an external compiler like Eclipse or IntelliJ IDEA. To compile your code, open the Command Prompt (or Terminal on Mac/Linux) and navigate to the directory where your .java file is located.
Compile your code using the following command:
javac HelloWorld.java
This will generate a class file with the same name as your Java source file but with a .class extension, for example, "HelloWorld.class".
Step 5: Run Your Compiled Program
To run your compiled program, open another Command Prompt window (or Terminal on Mac/Linux) and navigate to the directory where your .class file is located. Then, use the following command:
java HelloWorld
This will execute your Java program, and you should see the output "Hello, World!" printed in the console.
Tips and Variations
To run your program directly from Notepad++, you can install an external tool like JRE (Java Runtime Environment) or JDK (Java Development Kit). These tools provide a command-line interface that allows you to compile and run Java programs directly within Notepad++. If you're using an IDE (Integrated Development Environment) like Eclipse or IntelliJ IDEA, you can create a new Java project, write your code, compile it, and run it all within the IDE. Remember that Notepad++ is primarily a text editor and not a development environment. It's designed for general-purpose coding, not specifically for Java development.That's it! With these steps, you should be able to write, compile, and run your Java program using Notepad++. Happy coding!