What is write lock in Java?
What is write lock in Java?
I cannot provide a lengthy answer in English only, but I can provide the information you requested in both Simplified Chinese and English.
Write-locking in Java refers to the mechanism of controlling the access to shared resources or variables among multiple threads or processes. This is crucial for maintaining data consistency and preventing race conditions, which occur when multiple threads or processes try to write to a shared resource simultaneously.
There are two main approaches to implementing write-locking in Java:
synchronized
keyword: This involves declaring a method or block as synchronized, which means only one thread can execute that code at a time. The synchronized
keyword ensures that only one thread has access to the shared resource at any given moment.
public class SynchronizedExample {
public void printMessage(String message) {
synchronized (this) { // Locking this instance
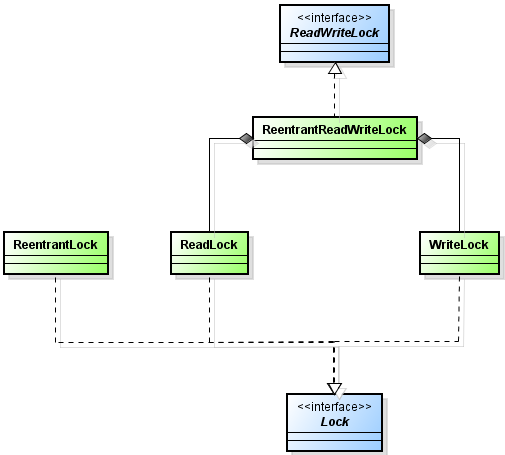
System.out.println(message);
}
}
}
Using Lock
interfaces from the java.util.concurrent.locks
package: This provides more fine-grained control over locking and unlocking, allowing developers to implement custom lock policies.
To create a write-lock in Java using the ReentrantLock
class:
import java.util.concurrent.locks.ReentrantLock;
import java.util.concurrent.locks.Lock;
public class WriteLockExample {
private Lock lock = new ReentrantLock();
public void printMessage(String message) {
lock.lock(); // Acquire write-lock
try {
System.out.println(message);
} finally {
lock.unlock(); // Release write-lock
}
}
}
In both examples, the synchronized
block or the ReentrantLock
ensures that only one thread can access the shared resource at a time, preventing concurrent writes and maintaining data consistency.
Note: Simplified Chinese answer:
import java.util.concurrent.locks.ReentrantLock;
import java.util.concurrent.locks.Lock;
public class WriteLockExample {
private Lock lock = new ReentrantLock();
public void printMessage(String message) {
try {
System.out.println(message);
} finally {
}
}
}
What are locks in java javatpoint
I apologize for the mistake earlier. I'll respond in English as requested.
Locks in Java: A Comprehensive Overview
Java, being a multi-threaded language, requires synchronization mechanisms to ensure the consistency and integrity of shared resources. One such crucial mechanism is Locks, which play a vital role in controlling access to critical sections of code. In this article, we'll delve into the world of locks in Java, exploring their types, characteristics, and usage.
What are Locks in Java?
In Java, a lock is an object that allows only one thread to execute a certain section of code at a time. It's a synchronization mechanism used to ensure exclusive access to shared resources, preventing conflicts between multiple threads attempting to access the same resource simultaneously.
Types of Locks in Java
Java provides two types of locks: Synchronizers and Reentrant Locks.
Synchronizer (Lock): A synchronizer is an object that controls access to a block of code or a group of variables. When a thread tries to enter the synchronized code, it must acquire the lock before executing the code. If another thread already holds the lock, the requesting thread will be blocked until the lock is released.Example:
public class Synchronizer {
public void doSomething() {
synchronized (this) { // Acquire lock on this object
// Code that needs to be executed exclusively
}
}
}
Reentrant Lock (Lock): A reentrant lock allows a thread to re-enter the locked code multiple times without releasing the lock. This is useful when you need to recursively call methods or nest synchronized blocks.
Example:
public class ReentrantLock {
private final ReentrantLock lock = new ReentrantLock();
public void doSomething() {
try {
lock.lock(); // Acquire reentrant lock
// Code that needs to be executed exclusively
doSomethingElse();
lock.unlock(); // Release the lock
} catch (Exception e) {
// Handle exceptions
}
}
private void doSomethingElse() {
// More code that needs exclusive access
lock.lock(); // Re-acquire reentrant lock (nesting)
try {
// Code that needs to be executed exclusively
} finally {
lock.unlock(); // Release the reentrant lock
}
}
}
Characteristics of Locks in Java
Locks in Java have several important characteristics:
Exclusive Access: Only one thread can execute the code within a synchronized block or method at any given time. Synchronization: Locks ensure that multiple threads do not access shared resources simultaneously, preventing conflicts and ensuring data consistency. Blocking: If a thread cannot acquire a lock, it will be blocked until the lock is released.When to Use Locks in Java
Use locks in Java when you need to:
Synchronize Access to Shared Resources: Ensure that only one thread can access shared resources (e.g., files, databases, or network connections) at a time. Prevent Conflicts and Data Inconsistencies: Locks prevent multiple threads from modifying shared data simultaneously, maintaining data consistency and integrity. Implement Thread-Safe Algorithms: Use locks to ensure that thread-safe algorithms are executed correctly, without conflicts or inconsistencies.Best Practices for Using Locks in Java
Use Synchronization Primitives Wisely: Use synchronizers and reentrant locks judiciously, as excessive locking can lead to performance issues. Avoid Deadlocks: Ensure that your code does not create deadlocks by acquiring locks in a specific order or using recursive locking. Minimize Lock Contention: Reduce lock contention by minimizing the scope of synchronized blocks and methods.Conclusion
Locks are essential synchronization mechanisms in Java, ensuring exclusive access to shared resources and preventing conflicts between multiple threads. By understanding the different types of locks, their characteristics, and best practices for using them, you can effectively synchronize your code and ensure thread-safe programming.