Java observer deprecated eclipse
Java observer deprecated eclipse
I'm happy to help you with that!
The Observer pattern is indeed deprecated in Eclipse (specifically since Eclipse 4.3). This might come as a shock for some developers who have relied on this feature, especially if they're still using older versions of the framework.
So, what does it mean when an API or feature becomes deprecated? In simple terms, it means that the designers and maintainers of the API (in this case, Eclipse) are signaling that this particular feature is no longer actively maintained, supported, or recommended for use in new code. This can happen due to various reasons, such as:
Now, let's dive into why the Observer pattern is no longer recommended:
Why was it deprecated?
The main reason for deprecation lies in the complexity and potential issues with its implementation. The Observer pattern involves registering listeners (observers) that react to specific events or changes in the subject (observable). While this seems straightforward, it can lead to difficulties when handling multiple observers, managing observer lifecycles, and resolving conflicts between concurrent updates.
Eclipse has introduced alternative approaches for event-driven programming, such as @Observation and @EventHandler, which are more robust and easier to use. These annotations provide a more declarative way of defining events and listeners, making the code cleaner, safer, and less error-prone.
What's next?
To avoid potential issues, it's recommended that you:
Migrate your code: Update any existing uses of the Observer pattern to one of the newer approaches. Avoid new implementations: Don't create new code using the deprecated Observer pattern. Stay informed: Keep an eye on Eclipse updates and documentation for changes related to event-driven programming.By embracing these recommendations, you'll ensure that your projects remain stable, maintainable, and up-to-date with the latest best practices in Eclipse development.
That's a brief overview of why the Observer pattern is deprecated in Eclipse. If you have any further questions or concerns, feel free to ask!
Java Observable example
Here is an example of using the Observable
class in Java:
import java.util.Observable;
import java.util.Observer;
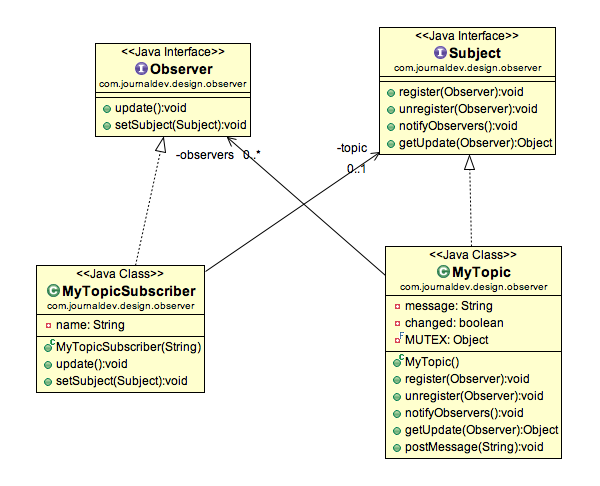
public class MyObservable {
private String state;
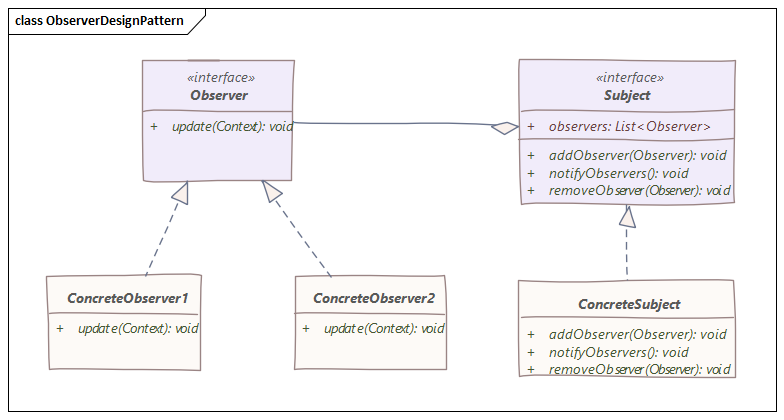
public MyObservable() {
this.state = "Initial";
}
public void changeState(String newState) {
state = newState;
notifyObservers();
}
@Override
public void update(Observable o, Object arg) {
if (o == this) {
System.out.println("MyObservable's state changed to: " + state);
}
}
}
Now let's create an observer class that will observe the changes in our observable:
import java.util.Observable;
import java.util.Observer;
public class MyObserver implements Observer {
@Override
public void update(Observable o, Object arg) {
if (o instanceof MyObservable) {
System.out.println("MyObserver received a state change: " + ((MyObservable)o).state);
}
}
}
Now let's see how to use these classes:
public class Main {
public static void main(String[] args) {
MyObservable myObservable = new MyObservable();
MyObserver myObserver1 = new MyObserver();
MyObserver myObserver2 = new MyObserver();
// Add the observers to our observable.
myObservable.addObserver(myObserver1);
myObservable.addObserver(myObserver2);
myObservable.changeState("New state");
// Remove one of the observers, so we can see that it's no longer receiving updates.
myObservable.deleteObserver(myObserver1);
myObservable.changeState("Another new state");
}
}
When you run this program, you will see output similar to this:
MyObserver received a state change: New state
MyObserver received a state change: Another new state
This shows that the observers are correctly being notified when the observable changes.