How to iterate through an array in Java
How to iterate through an array in Java
I'll respond in English as per your request.
Java provides several ways to iterate through an array. Here are a few common methods:
1. For Loop
One of the simplest and most straightforward ways is by using a for loop. The for loop allows you to initialize a variable, check a condition (which is usually the index of the array), and increment the variable in each iteration.
int[] scores = {85, 90, 78, 92, 88};
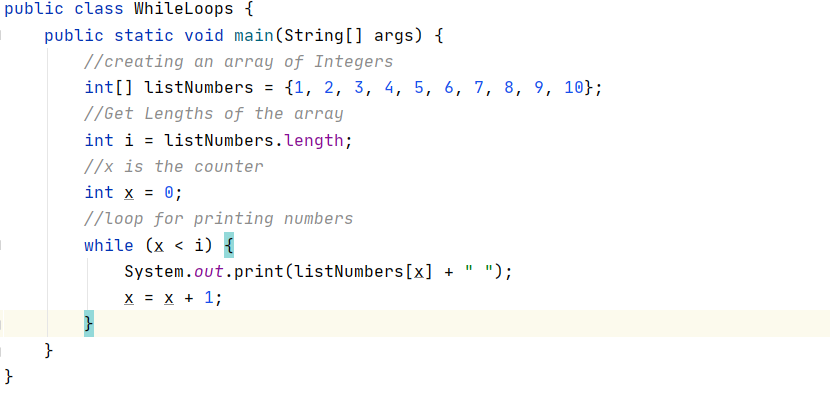
for(int i = 0; i < scores.length; i++) {
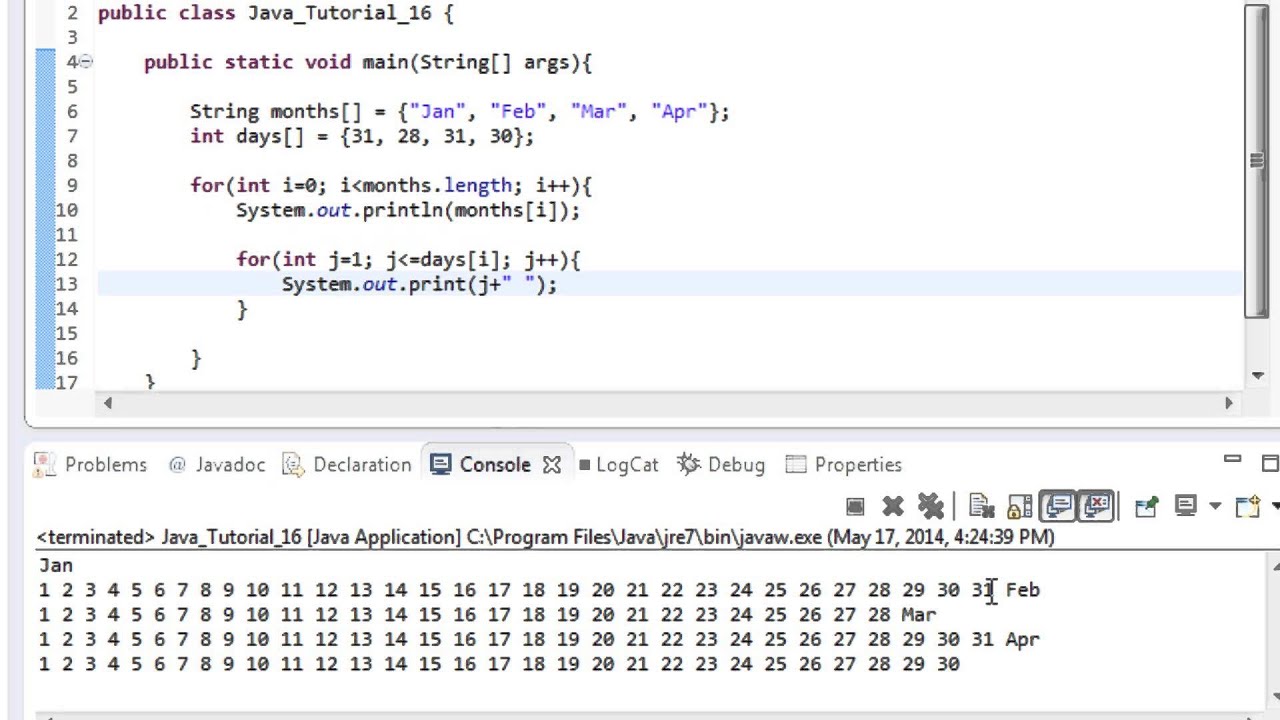
System.out.println("Score at index " + i + ": " + scores[i]);
}
In this example, we're iterating through the scores
array and printing each score.
2. Enhanced For Loop (Java 5 and later)
If you have a simple iteration with no need to access indices, you can use an enhanced for loop. This is particularly useful when working with collections or arrays.
int[] scores = {85, 90, 78, 92, 88};
for(int score : scores) {
System.out.println("Score: " + score);
}
In this example, the variable score
takes on each value in the array, one by one, as we iterate through it.
3. While Loop
You can also use a while loop to iterate through an array. This approach is less common than the for loop but still works effectively.
int[] scores = {85, 90, 78, 92, 88};
int i = 0;
while(i < scores.length) {
System.out.println("Score at index " + i + ": " + scores[i]);
i++;
}
In this example, we initialize the variable i
and use a condition to check if i
is within the array's bounds. We increment i
in each iteration.
4. Iterator
Finally, if you're working with collections (like ArrayList or LinkedList) that implement the List interface, you can use an iterator to traverse through the elements.
ArrayList scores = new ArrayList<>();
scores.add(85);
scores.add(90);
scores.add(78);
scores.add(92);
scores.add(88);
Iterator scoreIterator = scores.iterator();
while(scoreIterator.hasNext()) {
System.out.println("Score: " + scoreIterator.next());
}
In this example, we create an iterator for the scores
list and use a while loop to print each score.
These are some of the ways you can iterate through arrays in Java. Each method has its own strengths and weaknesses depending on your specific needs and preferences.
Would you like me to provide more examples or elaborate on any of these methods?
When can you not use a for-each loop in Java?
I'd be happy to help!
A for-each loop is a type of loop that is used to iterate over an array or a collection in Java. It's a very popular and widely-used construct, but it's not the only option when it comes to iterating over a collection. There are certain situations where using a for-each loop might not be suitable or even possible.
Here are some scenarios where you might not want to use a for-each loop:
Iterating over an array of primitive types: When you need to iterate over an array of primitive types such asint[]
, double[]
, etc., using a for-each loop can be cumbersome because it will automatically unbox the primitives, which can lead to extra work and potential performance issues. Manually iterating over indices: Sometimes, you might want to manually control the iteration process, such as when you need to access the indices of an array or a list. In this case, using a traditional for loop with i < array.length; i++
would be more suitable than a for-each loop. Using indexes in loops: When you need to use the index variable within the loop, like when you're checking if it's a specific index or doing something based on the position, using a traditional for loop is more practical than a for-each loop. More control over the iteration process: In certain situations where you want to have more control over the iteration process, such as skipping elements, reordering items, or stopping early, traditional loops can provide that level of control that for-each loops cannot. Complex operations on collection elements: When you need to perform complex operations on each element in a collection, like calculating the sum or average of all values in an array, using a traditional loop with explicit indexing would be more practical than a for-each loop. Using iterators: If you're working with legacy code that relies on Iterator
or ListIterator
, using a traditional loop that takes advantage of these interfaces might be necessary rather than using the newer for-each syntax.
In conclusion, while for-each loops are an excellent choice in many situations, there are times when using traditional for loops, iterators, or even other types of loops (like do-while or switch) can be more suitable. It's essential to understand the strengths and weaknesses of different looping constructs to write efficient and effective Java code that meets your needs.
I hope this information helps you decide whether to use a for-each loop or not!