What is inheritance in Java with realtime example?
What is inheritance in Java with realtime example?
I'll respond in English this time!
Inheritance is a fundamental concept in object-oriented programming (OOP) and is used to create a new class based on an existing class. The new class, known as the subclass or derived class, inherits all the attributes and methods of the original class, known as the superclass or parent class.
Here's a real-time example of inheritance in Java:
Suppose you're building a software application that simulates different types of vehicles, such as cars, buses, trucks, motorcycles, etc. You want to create a common base class that defines attributes and methods shared by all vehicles, like speed, acceleration, and braking ability.
Let's define the Vehicle superclass:
public abstract class Vehicle {
protected int speed; // default 0
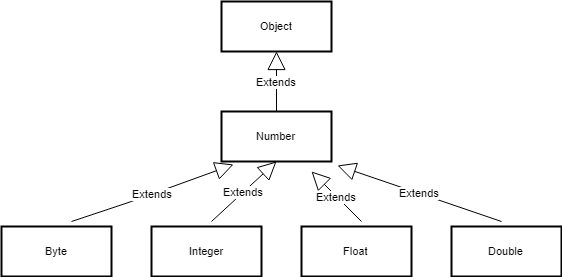
protected int acceleration; // default 1
public void accelerate() {
speed += acceleration;
System.out.println("Accelerating to " + speed + " mph!");
}
public void brake() {
speed -= 5; // slow down by 5 mph
System.out.println("Braking, slowing down to " + speed + " mph...");
}
}
Now, let's create a Car subclass that inherits from Vehicle:
public class Car extends Vehicle {
private String brand;
private int numDoors;
public Car(String brand, int numDoors) {
this.brand = brand;
this.numDoors = numDoors;
}
public void honkHorn() {
System.out.println("Vroom! Honking horn on my " + brand + " with " + numDoors + " doors!");
}
}
As you can see, the Car class inherits all the attributes and methods from the Vehicle superclass. It also adds its own custom attribute (brand) and method (honkHorn).
Here's an example of creating a new car object and calling its inherited and custom methods:
public static void main(String[] args) {
Car myCar = new Car("Toyota", 4);
myCar.accelerate(); // inherits accelerate() from Vehicle
myCar.brake(); // also inherits brake()
myCar.honkHorn(); // calls custom honkHorn() method
}
When you run the program, it will output:
Accelerating to 1 mph!
Braking, slowing down to -4 mph...
Vroom! Honking horn on my Toyota with 4 doors!
Inheritance enables code reuse and facilitates creating a hierarchy of related classes. In this example, the Car class inherits common vehicle behavior (speed, acceleration, braking) from the Vehicle superclass, while adding its own unique characteristics (brand, numDoors). This encapsulates the shared behaviors and allows for more flexibility in designing and extending classes.
This is just a basic example to illustrate inheritance in Java. In real-world scenarios, you'll often see more complex inheritance hierarchies with multiple levels of subclassing, polymorphism, and method overriding.
What is a multilevel inheritance in Java?
I'd be happy to explain multilevel inheritance in Java!
In object-oriented programming (OOP), inheritance is a mechanism that allows one class to inherit the properties and behavior of another class. In Java, this is achieved using the extends
keyword.
Multilevel inheritance refers to the process of inheriting from a subclass, which itself inherits from a superclass. This creates a hierarchical relationship between the classes involved.
Here's an example to illustrate this concept:
// Superclass (Grandparent)
class Animal {
public void sound() {
System.out.println("The animal makes a sound.");
}
}
// Subclass (Parent)
class Mammal extends Animal {
public void feedBaby() {
System.out.println("Mammals feed their babies.");
}
}
// Multilevel subclass
class Human extends Mammal {
public void walk() {
System.out.println("Humans walk upright.");
}
// Overriding the sound method from Animal
@Override
public void sound() {
System.out.println("Humans talk.");
}
}
In this example:
Animal
is the superclass, which has a sound()
method. Mammal
is a subclass of Animal
, which inherits the sound()
method and adds its own feedBaby()
method. Human
is a subclass of Mammal
, which inherits both the sound()
method from Animal
and the feedBaby()
method from Mammal
. Additionally, it defines its own walk()
method.
When you create an instance of Human
, you can access all the methods from the hierarchy:
public class Main {
public static void main(String[] args) {
Human human = new Human();
human.sound(); // Output: Humans talk.
human.feedBaby(); // Output: Mammals feed their babies.
human.walk(); // Output: Humans walk upright.
}
}
Multilevel inheritance provides a way to reuse code, promote modularity, and create complex relationships between classes. However, it can also lead to a phenomenon known as the "diamond problem" if not handled correctly.
In conclusion, multilevel inheritance in Java allows for a hierarchical structure of classes, enabling code reuse and facilitating more complex relationships between objects.