Is inheritance allowed in Java?
Is inheritance allowed in Java?
I can respond in English.
Yes, inheritance is indeed allowed in Java. In fact, it's a fundamental concept in object-oriented programming (OOP) that allows you to create a new class based on an existing one. This mechanism enables code reuse, modularity, and facilitates the creation of complex hierarchies of classes.
In Java, there are two types of inheritance: single inheritance and multiple inheritance. Single inheritance is when a subclass inherits directly from only one superclass. For example:
public class Animal { }
public class Dog extends Animal { }
Multiple inheritance is when a subclass can inherit from multiple superclasses. However, in Java, you cannot achieve this through direct extension (i.e., using the extends
keyword). Instead, you need to use interfaces to achieve multi-level inheritance.
Here's an example of multiple inheritance using interfaces:
public interface Animal { }
public interface Mammal { }
public class Dog implements Animal, Mammal { }
Now, let's explore some key aspects of inheritance in Java:
Method Overriding: When a subclass provides its own implementation for a method inherited from the superclass, it is called overriding. For instance:public class Animal {
public void makeSound() {
System.out.println("Animal makes sound");
}
}
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Dog barks");
}
}
In this example, the Dog
class overrides the makeSound()
method of its superclass (Animal
).
public class Calculator {
public int calculate(int num) {
return num;
}
public double calculate(double num) {
return Math.sqrt(num);
}
}
In this example, the Calculator
class has two methods with the same name (calculate
) but different parameter types.
super()
) or create your own constructor in the subclass. Here's an example:
public class Animal {
public Animal() {
System.out.println("Animal is created");
}
}
public class Dog extends Animal {
public Dog() {
super(); // Calls Animal()'s constructor
System.out.println("Dog is created");
}
}
In this example, the Dog
class calls its superclass's constructor (Animal
) using the super()
keyword.
public class Animal {
public void makeSound() { ... }
}
public class Dog extends Animal {
public void bark() {
// Can call Animal()'s method: makeSound()
makeSound();
}
}
In this example, the Dog
class can access its superclass's makeSound()
method because it is declared as public.
In summary, inheritance in Java allows you to create a new class that builds upon an existing one. It enables code reuse and facilitates complex hierarchies of classes. By understanding the concepts of single and multiple inheritance, method overriding and overloading, constructor inheritance, and access modifiers, you can effectively utilize inheritance in your Java programming endeavors.
So, is inheritance allowed in Java? Absolutely!
How to access parent class variable in child class in Java
I'm happy to help you with that! Here's a detailed explanation of how to access the variables of a parent class in a child class in Java:
In object-oriented programming, inheritance is a mechanism where a child class inherits the properties and behavior of its parent class. In Java, this is achieved through the use of the extends
keyword when creating a new class.
Let's consider an example:
Suppose we have a parent class called Animal
, which has two variables: name
and sound
. We can create a child class called Dog
, which inherits from Animal
.
public class Animal {
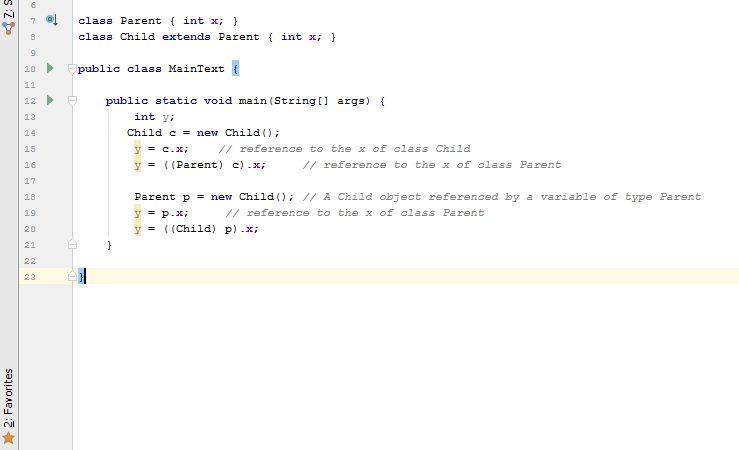
private String name;
private String sound;
public Animal(String name, String sound) {
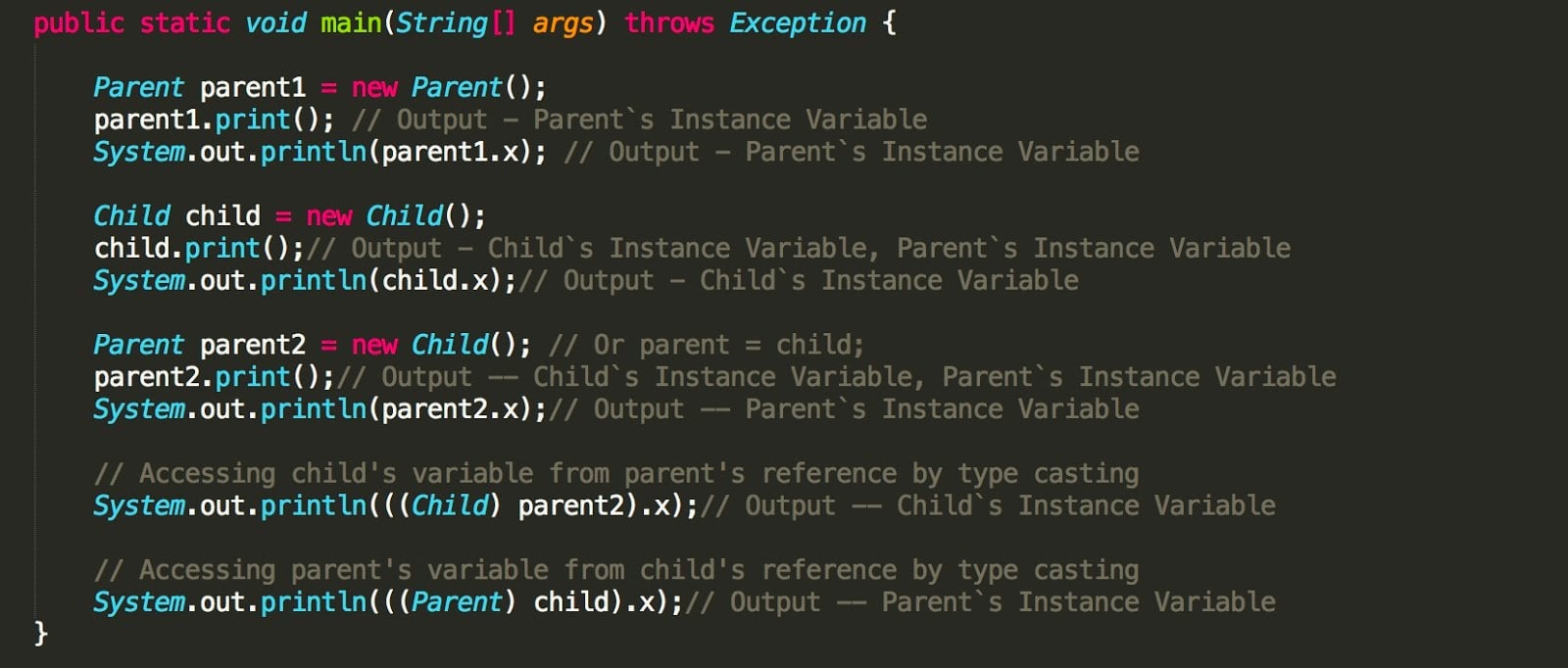
this.name = name;
this.sound = sound;
}
public String getName() {
return name;
}
public String getSound() {
return sound;
}
}
public class Dog extends Animal {
public Dog(String name, String sound) {
super(name, sound);
}
public void bark() {
System.out.println(getSound()); // Accessing the sound variable from the parent class
}
}
As you can see, in the Dog
class, we are accessing the sound
variable using the method getSound()
which is inherited from the Animal
class. This is an example of polymorphism, where an object of a child class can behave like its parent class.
However, if we try to access the variables directly without going through accessor methods (like getName()
or getSound()
), Java will prevent us from doing so. This is because the variables are private, and the Dog
class does not have direct access to them.
public class Dog extends Animal {
public Dog(String name, String sound) {
this.name = name; // Error: cannot assign a value to a final variable
this.sound = sound;
}
}
As you can see, even if we try to assign a new value to the name
and sound
variables directly in the Dog
class, Java will not allow us to do so. This is because the variables are declared as private in the Animal
class, and the Dog
class does not have direct access to them.
To overcome this limitation, we can provide accessor methods (getters) in the parent class that allow the child class to access its variables. In our example, we provided getter methods getName()
and getSound()
which the Dog
class can use to access the name
and sound
variables respectively.
In conclusion, to access parent class variables in a child class in Java:
Ensure that the variable is declared as public or protected (not private) in the parent class. Use accessor methods (getters) provided by the parent class to access its variables.By following these guidelines, we can ensure that our code adheres to principles of encapsulation and polymorphism, which are essential features of object-oriented programming.