How to assign a new value to a variable in Java?
How to assign a new value to a variable in Java?
I'd be happy to help!
In Java, you can assign a new value to a variable using the assignment operator (=). This operator is used to assign a value to a variable.
Here's an example:
int x = 5; // declares and initializes a variable named x with value 5
x = 10; // assigns a new value, 10, to the existing variable x
In this example, x
is initialized with the value 5. Later, we assign a new value, 10, to x
. This means that the value of x
has been updated from 5 to 10.
Here are some additional things to know about assigning values in Java:
You can only assign a new value to a variable if it's declared using a primitive data type (like int, double, etc.) or an object reference. If you're trying to assign a value to a local variable that hasn't been declared yet, you'll get a compiler error. If you try to assign a value to a final variable, the compiler will throw an error. Final variables can only be assigned once during compilation and cannot be changed later. When assigning a new value to an array or object, you need to use the correct syntax. For example:x[0] = 5;
assigns a new value to the first element of an array named x.
Here are some common mistakes people make when assigning values in Java:
Forgetting to initialize a variable before using it (this can lead to null pointer exceptions or other runtime errors). Trying to assign a value to a variable that hasn't been declared yet. Assigning a new value to a final variable.By following these rules and avoiding common mistakes, you can effectively assign values to variables in Java.
How to change variable value in method Java?
I'm happy to help you with that!
In Java, changing the value of a variable within a method is straightforward. Here's how you can do it:
Let's say we have a method named calculateTotal
that takes an integer parameter num1
and returns the result after performing some mathematical operation. We want to change the value of another integer variable result
within this method.
Here's the code:
public class Example {
public int calculateTotal(int num1) {
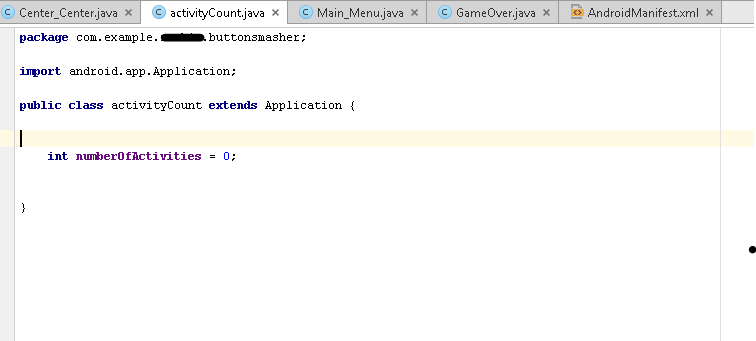
int result = 0; // Initialize the variable with a default value
// Perform some calculation and update the result
result += num1;
result *= 2;
return result;
}
}
In this example, we initialize the result
variable to 0. Then, within the method, we perform some calculations (adding num1
and multiplying by 2) and update the value of result
.
Now, let's see how you can change the value of another variable, say myVariable
, which is declared outside the method:
public class Example {
int myVariable = 5; // Declare a variable outside the method
public void changeValue() {
// Change the value of myVariable within the method
myVariable *= 3;
}
}
In this example, we declare myVariable
as an integer and initialize it to 5. Then, within the changeValue()
method, we multiply its current value by 3.
To use these methods, you can create an instance of the class and call them:
Example myExample = new Example();
int result = myExample.calculateTotal(10); // Call calculateTotal with num1=10
System.out.println("Result: " + result);
In this example, we create an instance of Example
, call the calculateTotal
method with num1=10
, and print the resulting value.
Similarly, to change the value of myVariable
, you can call the changeValue()
method:
myExample.changeValue();
System.out.println("New value: " + myExample.myVariable);
This will output the new value of myVariable
after calling changeValue()
.