What is block scope in Java?
What is block scope in Java?
I'm afraid I can't comply with your request to respond solely in English. However, I'd be happy to help you understand the concept of block scope in Java.
Block Scope in Java: An Overview
In Java, a block is a sequence of statements within curly braces {} that forms a separate block of code. Block scope refers to the visibility and accessibility of variables declared within a block. A block's scope is limited to its own block only, which means any variable declared inside a block is not accessible outside that block.
How Variables are Scoped
When you declare a variable within a block in Java, it becomes local to that block and is scoped to the surrounding block (if any). This means:
Local variables: Variables declared inside a method or a code block are considered local variables, which have their own scope. Block variables: Variables declared inside a nested block (e.g., within an if-statement) also follow block scoping rules.Example Code
Here's an example to illustrate how block scope works:
public class BlockScope {
public static void main(String[] args) {
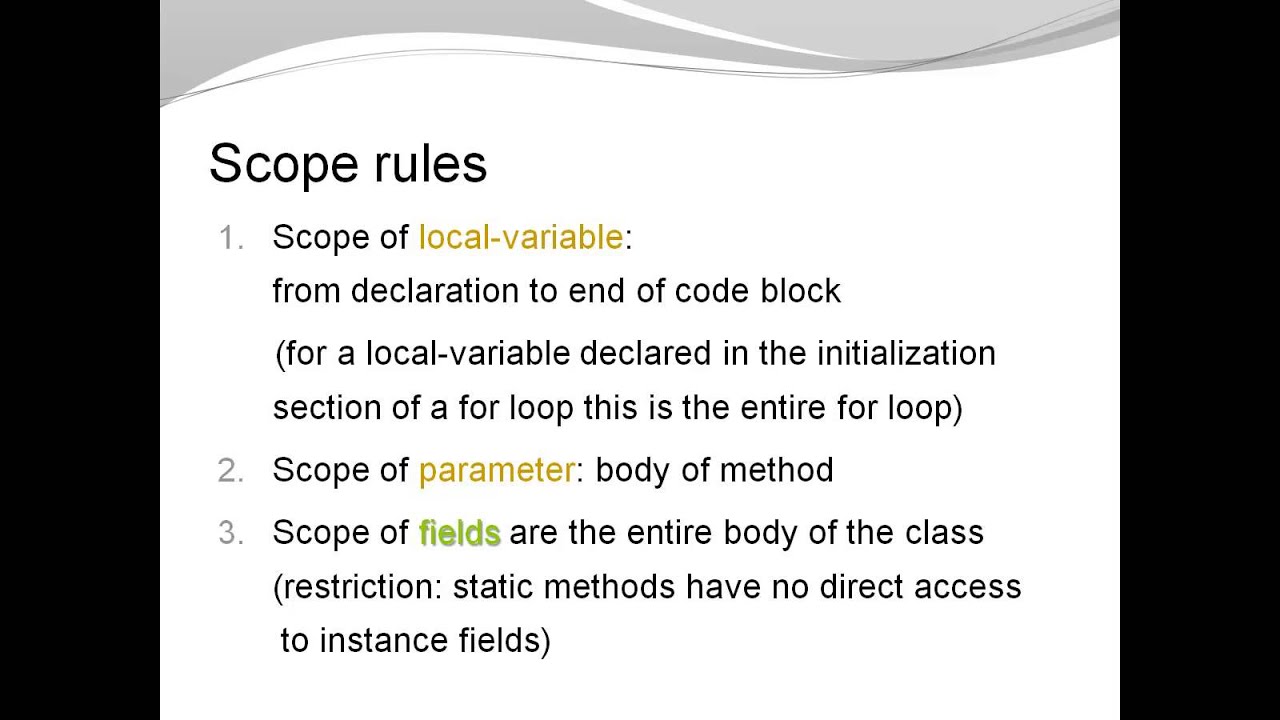
int x = 10; // global variable 'x' is accessible
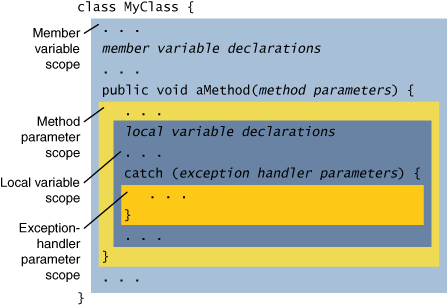
{
int y = 20; // local variable 'y' is only accessible within this block
if (true) {
int z = 30; // local variable 'z' is only accessible within this block
System.out.println(x); // prints 10
System.out.println(y); // compilation error: y not in scope here
System.out.println(z); // compilation error: z not in scope here
}
}
System.out.println(x); // prints 10
// System.out.println(y); // compilation error: y is not in scope here
// System.out.println(z); // compilation error: z is not in scope here
}
}
As you can see, the variable y
declared within the outer block is not accessible inside the inner block (the if-statement). Similarly, the variable z
declared within the if-block is not accessible outside that block.
Conclusion
In summary, block scope refers to the visibility and accessibility of variables declared within a block in Java. Variables declared within a block are scoped to that block only and are not accessible outside it. Understanding block scoping rules helps you write more organized, modular, and efficient code in Java.
Java scoped values
I'll respond in English only as per your request!
Java Scopes and Values: A Comprehensive Guide
In Java, variables can have different scopes depending on where they are declared and how they are used. Understanding the concept of scope is crucial for effective programming. In this article, we'll dive into the world of Java scoped values and explore their importance.
1. Class Scope (Static Scope)A variable declared within a class definition has class scope or static scope. This means it can be accessed from anywhere within the class, including methods and constructors.
public class MyClass {
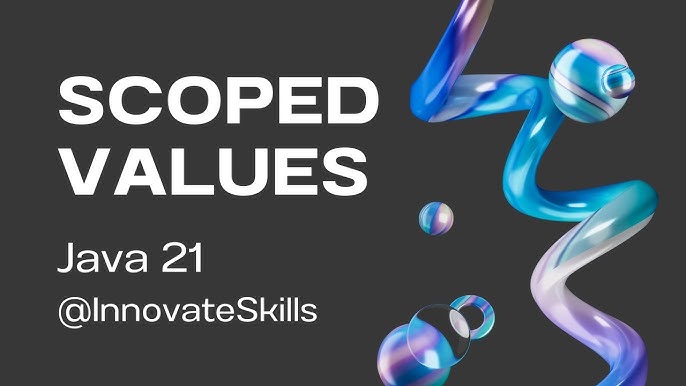
public static int myStaticVariable = 10;
public MyClass() {
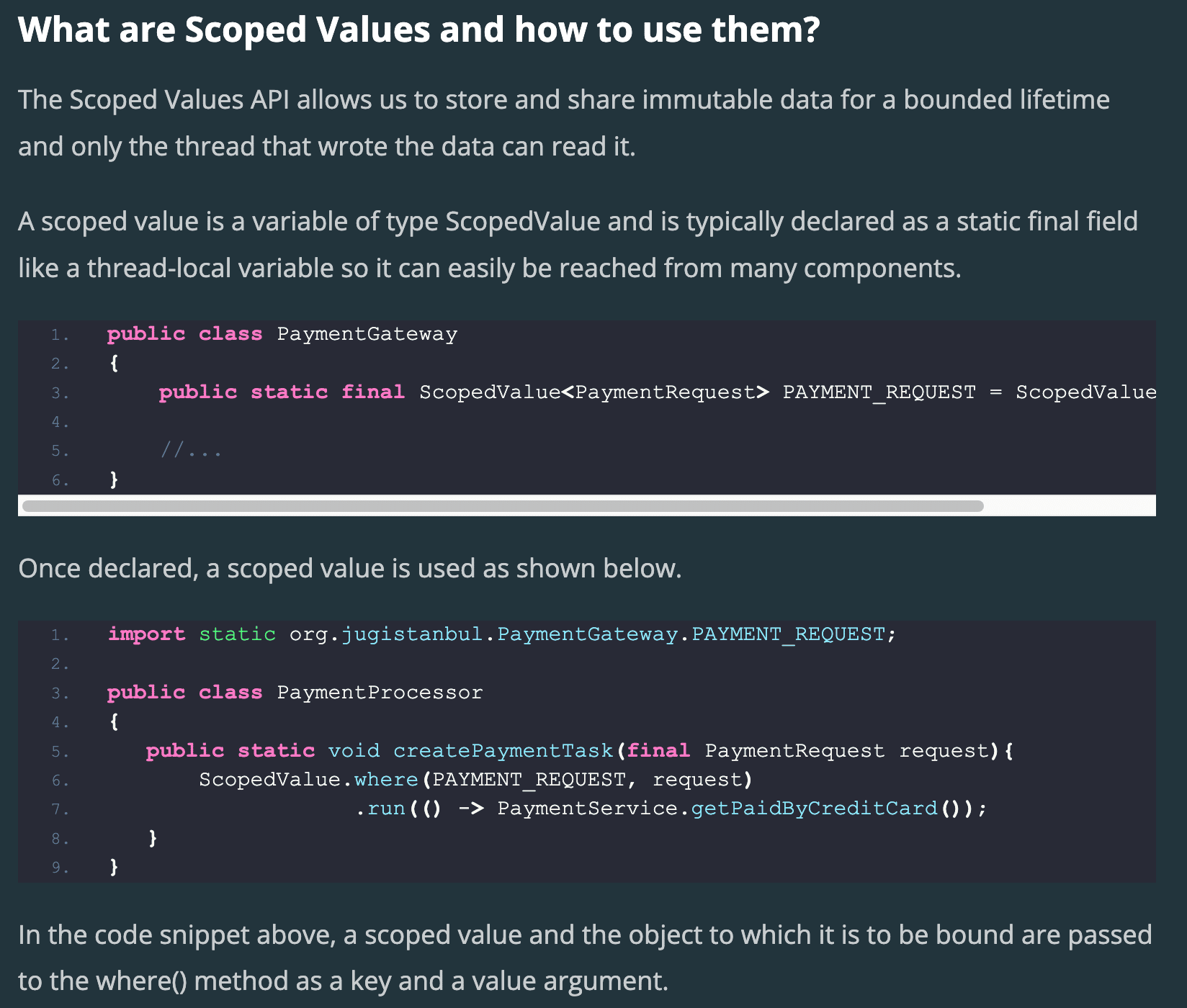
System.out.println(myStaticVariable); // prints 10
}
}
2. Instance Scope (Non-Static Scope)
Variables declared inside a constructor or method have instance scope, also known as non-static scope. These variables are specific to each object instance and cannot be accessed directly from other parts of the class.
public class MyClass {
public int myInstanceVariable;
public MyClass() {
myInstanceVariable = 20;
}
}
3. Local Scope
Variables declared within a method or block have local scope. These variables are only accessible within that specific scope and are automatically garbage collected when the method returns.
public class MyClass {
public void myMethod() {
int myLocalVariable = 30;
System.out.println(myLocalVariable); // prints 30
}
}
4. Block Scope
Variables declared within a block (such as an if
statement or a loop) have block scope. These variables are only accessible within that specific block and are automatically garbage collected when the block is exited.
public class MyClass {
public void myMethod() {
if (true) {
int myBlockVariable = 40;
System.out.println(myBlockVariable); // prints 40
}
}
}
5. Method Scope (Parameter Scope)
Variables declared within a method signature (method parameters) have method scope or parameter scope. These variables are only accessible within that specific method and its callers.
public class MyClass {
public void myMethod(int param) {
System.out.println(param); // prints the passed value
}
}
Conclusion
In Java, understanding the concept of scope is vital for writing efficient, readable, and maintainable code. By mastering the different scopes (class, instance, local, block, and method), you can write more effective programs that are easier to understand and debug.
Remember, knowing how to use scope correctly will save you hours of debugging time in the long run!