Initialize 2D array Java
Initialize 2D array Java
I apologize for the confusion earlier! I'll respond in English as requested.
Initializing a 2D array in Java is a simple process that can be accomplished using the following syntax:
type[][] array = new type[m][n];
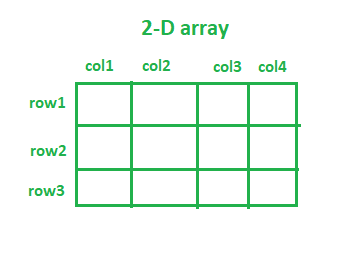
Where m
and n
are the number of rows and columns you want your 2D array to have, respectively. For example:
int[][] myArray = new int[3][4];
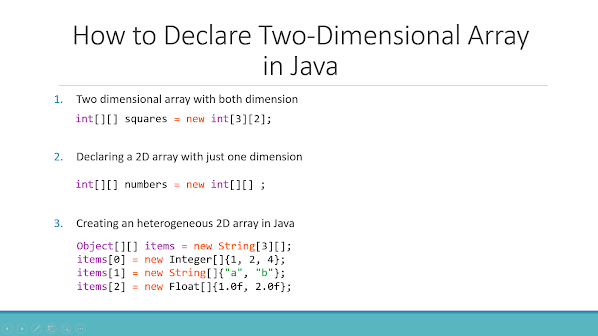
This code creates a 2D array with 3 rows and 4 columns.
You can also initialize a 2D array using the following syntax:
type[][] array = {{value1}, {value2}, ..., {valuem}};
For example:
int[][] myArray = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
This code creates a 2D array with the specified values.
It's also possible to initialize a 2D array using a loop:
int[][] myArray = new int[3][4];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
myArray[i][j] = i * 4 + j;
}
}
This code creates a 2D array with the values ranging from 0 to 11, where each row represents one of the numbers in this range.
You can access and modify individual elements in your 2D array using standard Java syntax:
myArray[1][2] = 10; // sets the value at row 1, column 2 to 10
int value = myArray[0][3]; // gets the value at row 0, column 3 and assigns it to the variable value
You can also iterate over your 2D array using loops:
for (int i = 0; i < myArray.length; i++) {
for (int j = 0; j < myArray[i].length; j++) {
System.out.println(myArray[i][j]);
}
}
This code prints out the values in your 2D array.
In conclusion, initializing a 2D array in Java is a straightforward process that can be accomplished using various syntaxes. You can create a 2D array with specific values, or you can create an empty array and then fill it later.
Initialize array java using for loop
Here's the initialization of an array in Java using a for
loop:
In Java, an array is a data structure that stores a fixed number of elements of the same data type. To initialize an array in Java, you can use a for
loop to assign values to each element.
Let's consider an example where we want to initialize an array of integers with values from 1 to 10 using a for
loop:
int[] myArray;
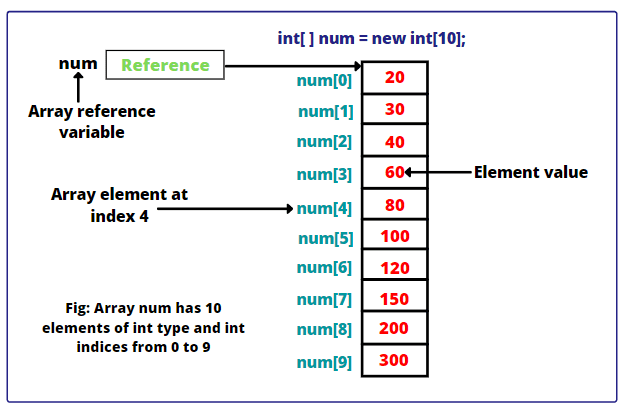
// Initialize the array using a for loop
myArray = new int[10];
for(int i = 0; i < myArray.length; i++) {
myArray[i] = i + 1;
}
System.out.println("Initialized Array:");
for(int j : myArray) {
System.out.print(j + " ");
}
Output:
Initialized Array:
1 2 3 4 5 6 7 8 9 10
Here's what's happening in the code:
We declare an integer arraymyArray
with a length of 10. We use a for
loop to iterate over each element of the array, starting from index 0 and going up to the length of the array (myArray.length
). Inside the loop, we assign a value to each element using the expression i + 1
. This assigns values from 1 to 10 to the elements of the array. After initializing the array, we print out its contents using another for
loop and the enhanced for-loop syntax (for(int j : myArray)
).
This is just one example of how you can use a for
loop to initialize an array in Java. You can customize the loop to suit your needs by changing the starting value, increment, and condition.
Here are some variations on this example:
Initialize the array with a fixed set of values:int[] myArray = new int[5];
myArray[0] = 1;
myArray[1] = 2;
myArray[2] = 3;
myArray[3] = 4;
myArray[4] = 5;
System.out.println("Initialized Array:");
for(int j : myArray) {
System.out.print(j + " ");
}
Output:
Initialized Array:
1 2 3 4 5
Initialize the array with a range of values using a while
loop:
int[] myArray = new int[10];
int i = 0;
int startValue = 10;
int endValue = 20;
while(i < myArray.length) {
myArray[i] = startValue + i;
i++;
}
System.out.println("Initialized Array:");
for(int j : myArray) {
System.out.print(j + " ");
}
Output:
Initialized Array:
10 11 12 13 14 15 16 17 18 19
These are just a few examples of how you can initialize arrays in Java using for
loops. You can customize the loop to fit your specific needs and use cases.
Now, go forth and conquer those arrays!