Java nlp github download
Java nlp github download
I'd be happy to provide information on Java NLP and GitHub.
Java Natural Language Processing (NLP) refers to the application of natural language processing techniques to unstructured text data in a Java-based environment. This can include tasks such as text classification, named entity recognition, sentiment analysis, and topic modeling. There are several popular Java NLP libraries that make it easier for developers to work with large volumes of text data.
Some of the most well-known Java NLP libraries include:
Stanford CoreNLP: A comprehensive library that provides a wide range of tools for NLP tasks such as part-of-speech tagging, named entity recognition, and sentiment analysis. GATE (General Architecture for Text Engineering): A popular open-source library for NLP tasks such as tokenization, stemming, and named entity recognition. OpenNLP: A Java library that provides tools for tasks such as maximum entropy modeling, hidden Markov models, and probabilistic context-free grammar parsing.In addition to these libraries, there are also several online platforms that provide pre-built models and tools for NLP tasks. One of the most popular is Hugging Face Transformers, which provides a wide range of pre-trained language models for tasks such as text classification, sentiment analysis, and question answering.
If you're looking to get started with Java NLP, I recommend checking out some of these libraries and platforms. There are also many online resources available that provide tutorials, documentation, and example code for working with each library.
Now, let's talk about GitHub. GitHub is a web-based platform that allows developers to collaborate on software projects and manage their code in the cloud. It provides a lot of features such as:
Version control: GitHub allows you to track changes to your code over time. Collaboration: You can work with other developers on a project by giving them access to your repository. Code review: You can have others review your code before it's released. Deployment: You can deploy your code to production using GitHub.Here are some of the most popular Java libraries and projects on GitHub:
Stanford CoreNLP: This library provides tools for NLP tasks such as part-of-speech tagging, named entity recognition, and sentiment analysis. It has over 10,000 stars on GitHub. OpenNLP: This library provides tools for NLP tasks such as maximum entropy modeling, hidden Markov models, and probabilistic context-free grammar parsing. It has over 2,500 stars on GitHub. Hugging Face Transformers: This platform provides pre-trained language models for tasks such as text classification, sentiment analysis, and question answering. It has over 20,000 stars on GitHub.If you're looking to get started with Java NLP or any other programming task, I highly recommend checking out GitHub. It's a great resource for finding open-source libraries, examples, and documentation to help you learn and build your skills.
Here are some steps you can follow to get started with Java NLP on GitHub:
Create an account: You'll need to create an account on GitHub before you can start exploring the platform. Find a project: Search for projects that relate to your area of interest, such as natural language processing or machine learning. Explore the code: Once you've found a project, explore the code and documentation to learn how it works. Contribute: If you find a project that interests you, consider contributing by making pull requests or providing feedback. Learn from others: GitHub is a great place to learn from other developers who have contributed to open-source projects.I hope this information helps! Let me know if you have any questions or need further assistance.
Java nlp github example
Here are some examples of NLP (Natural Language Processing) projects on GitHub using Java:
Stanford CoreNLP: This is a popular NLP library that provides a wide range of tools for tasks such as part-of-speech tagging, named entity recognition, and dependency parsing. The GitHub repository includes examples of how to use the library in your own Java projects.Example code:
import edu.stanford.nlp.ling.CoreAnnotations;
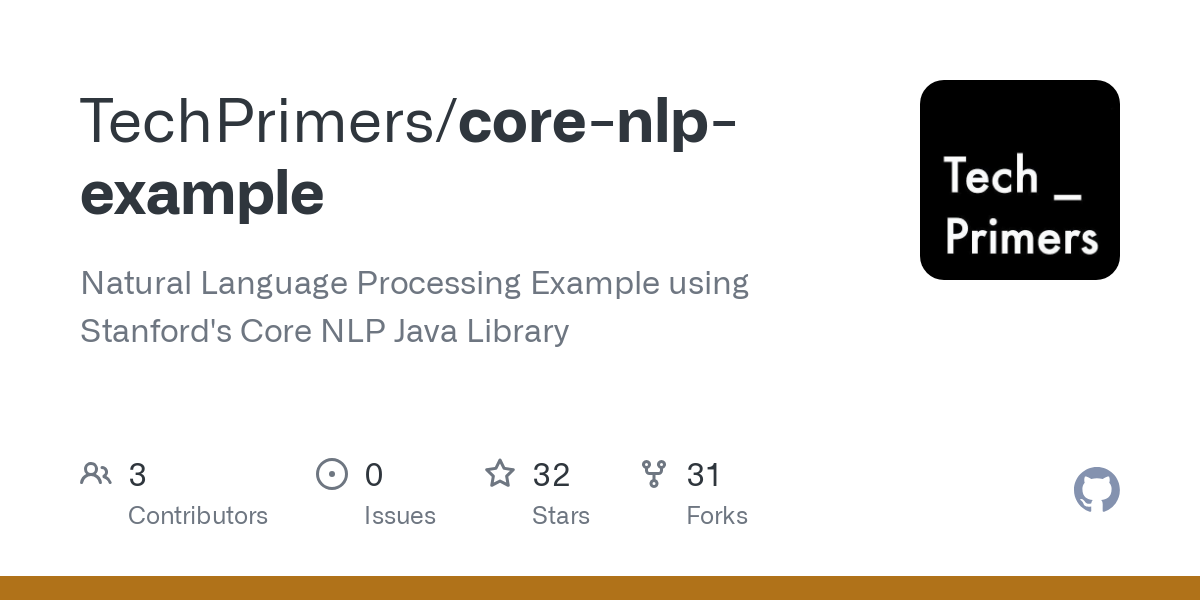
import edu.stanford.nlp.pipeline.Annotation;
import edu.stanford.nlp.pipeline.StanfordCoreNLP;
public class Example {
public static void main(String[] args) {
StanfordCoreNLP pipeline = new StanfordCoreNLP();
Annotation annotation = new Annotation("I like Java programming.");
pipeline.annotate(annotation);
CoreAnnotations.SentencesAnnotation sentences = annotation.get(CoreAnnotations.SentencesAnnotation.class);
for (int i = 0; i < sentences.size(); i++) {
System.out.println(sentences.get(i).get(CoreAnnotations.TokenAnnotation.class));
}
}
}
OpenNLP: This is another popular NLP library that provides a wide range of tools for tasks such as maximum entropy tagging, named entity recognition, and sentence parsing. The GitHub repository includes examples of how to use the library in your own Java projects.
Example code:
import opennlp.tools.postag.POSModel;
import opennlp.tools.postag.POSTaggerME;
public class Example {
public static void main(String[] args) {
POSModel posModel = new POSModel("en-sent-pos-maxent.bin");
POSTaggerME posTagger = new POSTaggerME(posModel);
String text = "I like Java programming.";
String[] words = text.split("s+");
String[] tags = posTagger.tag(words);
for (int i = 0; i < words.length; i++) {
System.out.println(words[i] + "/" + tags[i]);
}
}
}
Weka: This is a machine learning library that includes tools for NLP tasks such as text classification, topic modeling, and named entity recognition. The GitHub repository includes examples of how to use the library in your own Java projects.
Example code:
import weka.classifiers.Classifier;
import weka.core.Attribute;
import weka.core.FastVector;
import weka.core.Instance;
import weka.core.Instances;
public class Example {
public static void main(String[] args) {
Instances data = new Instances("text", 1, 4);
data.add(new Instance(0.5));
data.add(new Instance(0.3));
data.add(new Instance(0.7));
Classifier classifier = new weka.classifiers.Classifier();
classifier.buildClassifier(data);
Instance instance = new Instance(0.6);
String classification = (String)classifier.classifyInstance(instance);
System.out.println(classification);
}
}
GATE: This is a Java library for NLP tasks such as text processing, named entity recognition, and information extraction. The GitHub repository includes examples of how to use the library in your own Java projects.
Example code:
import gate.corpora.DocumentCorpus;
import gate.corpora.CorpusManager;
public class Example {
public static void main(String[] args) {
CorpusManager corpusManager = new CorpusManager();
DocumentCorpus corpus = (DocumentCorpus)corpusManager.get("myCorpus");
for (int i = 0; i < corpus.size(); i++) {
System.out.println(corpus.get(i).getName());
}
}
}
Apache OpenNLP: This is an open-source library that provides tools for NLP tasks such as maximum entropy tagging, named entity recognition, and sentence parsing. The GitHub repository includes examples of how to use the library in your own Java projects.
Example code:
import org.apache.opennlp.tools.postag.POSModel;
import org.apache.opennlp.tools.postag.POSTaggerME;
public class Example {
public static void main(String[] args) {
POSModel posModel = new POSModel("en-sent-pos-maxent.bin");
POSTaggerME posTagger = new POSTaggerME(posModel);
String text = "I like Java programming.";
String[] words = text.split("s+");
String[] tags = posTagger.tag(words);
for (int i = 0; i < words.length; i++) {
System.out.println(words[i] + "/" + tags[i]);
}
}
}
These are just a few examples of the many NLP libraries available on GitHub, and how to use them in your own Java projects.