google cloud storage java example
google cloud storage java example
I'd be happy to help!
Google Cloud Storage is a service that provides a highly durable and scalable data store for unstructured data, such as images, videos, and documents. Here's an example of how you can use Java to interact with Google Cloud Storage:
Step 1: Set up your project
To get started, you'll need to set up a new project in the Google Cloud Console. If you're not familiar with the process, here are the general steps:
Step 2: Enable the Cloud Storage API
Once you've created your project, you'll need to enable the Cloud Storage API. Here's how:
In the Google Cloud Console, go to the navigation menu and click on "APIs & Services." Click on "Dashboard" and then click on the "Enable APIs and Services" button. Search for "Cloud Storage" in the search bar and click on the result. Click on the "Enable" button to enable the API.Step 3: Install the Google Cloud Client Library
To interact with Cloud Storage from your Java application, you'll need to install the Google Cloud Client Library. Here's how:
Open a terminal or command prompt and navigate to the directory where you want to install the library. Run the following command:mvn add archetype -DarchetypeArtifactId=google-cloud-archetype
Follow the prompts to create a new Maven project. Once the project is created, run the following command: cd my-project && mvn compile
Step 4: Use the Google Cloud Client Library in your Java application
Now that you have the library installed, you can start using it in your Java application. Here's an example:
import com.google.cloud.storage.Blob;
import com.google.cloud.storage.Storage;
import com.google.cloud.storage.StorageOptions;
public class Main {
public static void main(String[] args) throws Exception {
// Create a Storage client instance.
Storage storage = StorageOptions.getDefaultInstance().getService();
// Get the bucket name and the path to the file you want to upload.
String bucketName = "my-bucket";
String filePath = "/path/to/file.txt";
// Create a Blob object using the bucket name, the path to the file,
// and the Storage client instance.
Blob blob = storage.get(bucketName).getBlob(filePath);
// Upload the file to Cloud Storage.
blob.upload();
// Download the file from Cloud Storage.
blob.downloadAsStream();
}
}
Step 5: Run your Java application
Now that you've written your Java code, it's time to run it. Here's how:
Navigate to the directory where your project is located in a terminal or command prompt. Run the following command:mvn exec:java -Dexec.mainClass=com.example.Main
This will compile and run your application.
That's it! You've now successfully used Java to interact with Google Cloud Storage.
How to connect to GCP bucket using Java?
Here is a detailed explanation on how to connect to Google Cloud Storage (GCS) bucket using Java.
Prerequisites:
You need to have the Google Cloud Platform (GCP) account and create a new project.
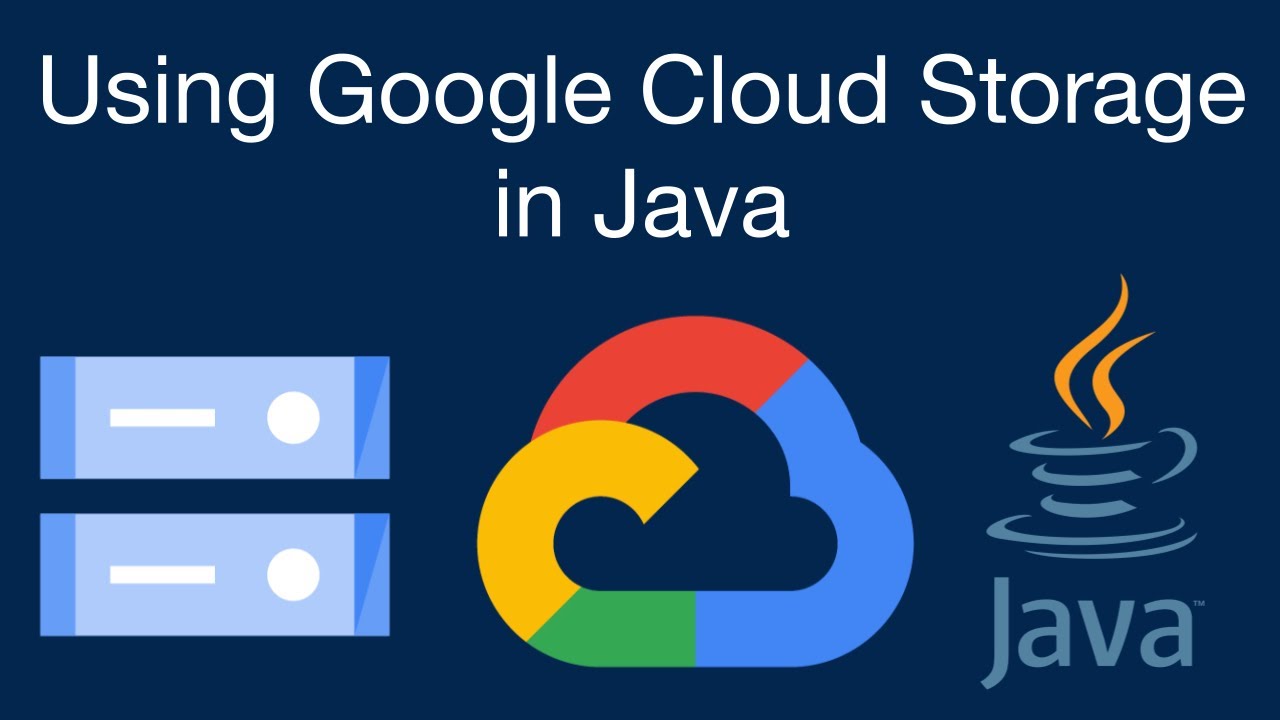
com.google.cloud
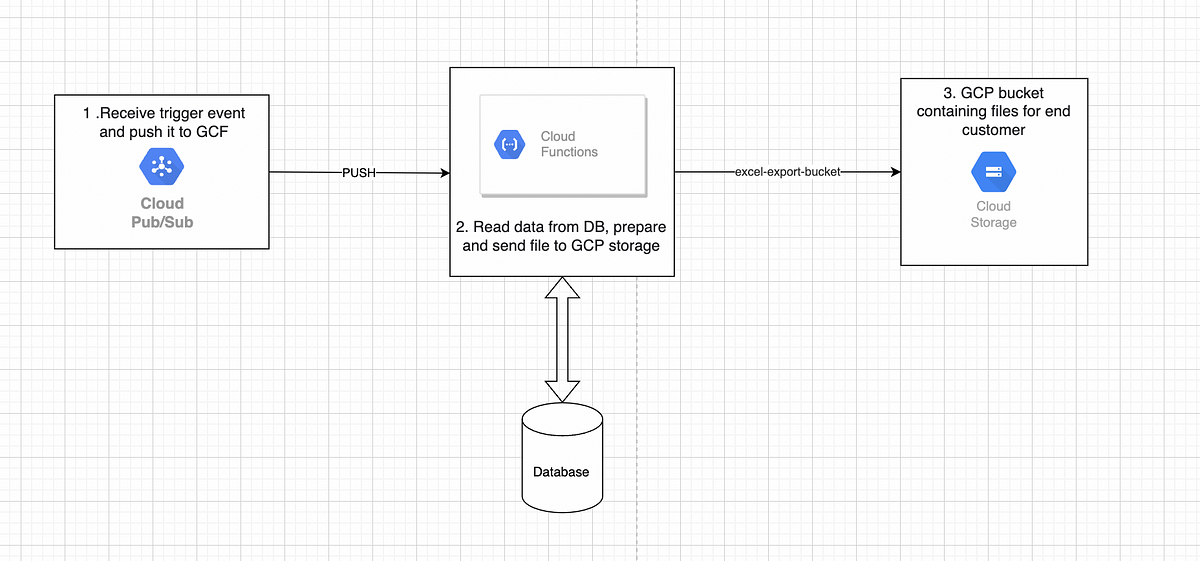
google-cloud-storage
1.123.0
Step 1: Create a credentials file
Create a credentials file named gcs-credentials.json
with the following content:
{
"type": "service_account",
"project_id": "<YOUR_PROJECT_ID>",
"private_key_id": "<PRIVATE_KEY_ID>",
"private_key": "<PRIVATE_KEY>",
"client_email": "<CLIENT_EMAIL>",
"client_id": "<CLIENT_ID>",
"auth_uri": "https://accounts.google.com/oath2/auth",
"token_uri": "https://oauth2.googleapis.com/token"
}
Replace <YOUR_PROJECT_ID>
, <PRIVATE_KEY_ID>
, <PRIVATE_KEY>
, <CLIENT_EMAIL>
, and <CLIENT_ID>
with your actual GCP credentials.
Step 2: Set up the Google Cloud Storage client
Create a Java class that sets up the Google Cloud Storage client:
import com.google.cloud.storage.*;
public class GcsClient {
public static void main(String[] args) {
// Create credentials from JSON key file
StorageOptions options = StorageOptions.newBuilder().setProjectId("<YOUR_PROJECT_ID>").fromServiceAccountJson("gcs-credentials.json").build();
// Create a client object
Storage storage = StorageOptions.getDefaultInstance().getService();
// Get the bucket reference
Bucket bucket = storage.getBucket("your-bucket-name");
// Use the bucket to perform operations, e.g., uploading files
}
}
Step 3: Authenticate and authorize
Call the create()
method on the Storage
object to authenticate and authorize:
// Get a reference to the GCS client
Storage storage = StorageOptions.getDefaultInstance().getService();
// Create credentials from JSON key file
StorageOptions options = StorageOptions.newBuilder().setProjectId("<YOUR_PROJECT_ID>").fromServiceAccountJson("gcs-credentials.json").build();
// Authenticate and authorize using the credentials
storage = options.getService();
Step 4: List bucket contents or upload files
You can now use the Bucket
object to list the contents of your GCS bucket, upload files, or perform other operations:
// Get a reference to the Bucket object
Bucket bucket = storage.getBucket("your-bucket-name");
// List the contents of the bucket
List blobs = bucket.list();
for (Blob blob : blobs) {
System.out.println(blob.getName());
}
// Upload a file to the bucket
blob.upload(new FileInputStream("local-file.txt"), "local-file.txt");
Step 5: Handle exceptions and errors
Don't forget to handle potential exceptions and errors that may occur during your GCS operations:
try {
// Perform GCS operation, e.g., uploading a file
bucket.upload(fileInputStream, "file.txt");
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
This is the basic process for connecting to a Google Cloud Storage (GCS) bucket using Java. Make sure to replace the placeholders with your actual GCP credentials and bucket name.
Additional tips:
Always use theStorageOptions
class to configure the GCS client, as it provides a more robust way of handling authentication and authorization. Use the Bucket
object to perform operations on your GCS bucket, such as listing contents or uploading files. Don't forget to handle potential exceptions and errors that may occur during your GCS operations.
I hope this helps! Let me know if you have any further questions.