Java mongodb orm tutorial
Java mongodb orm tutorial
Here is a comprehensive guide to using MongoDB with a Java ORM (Object Relational Mapping) tool:
Why use an ORM?
MongoDB is a NoSQL database that stores data in JSON-like documents called BSON. While MongoDB provides a robust query language, it can be cumbersome to interact with the database directly from your Java code. This is where an ORM comes in - it acts as a layer between your Java application and the MongoDB database, allowing you to work with objects rather than raw data.
What is Morphia?
Morphia is a popular Java ORM library that provides a simple and intuitive way to interact with MongoDB from your Java application. It supports most of the MongoDB features, including queries, aggregations, and CRUD (Create, Read, Update, Delete) operations.
Setting up Morphia
To use Morphia in your Java project, follow these steps:
Add Morphia as a dependency in your pom.xml file (if you're using Maven):
com.mysema
morphia
1.3.2
Create a Morphia instance and configure it to connect to your MongoDB database:
Morphia morphia = new Morphia();
morpha.map("com.example.models", com.example.models);
Map<String, String> config = new HashMap<>();
config.put("hosts", "localhost:27017");
config.put("username", "your_username");
config.put("password", "your_password");
morpha.close();
Defining your Java classes
To use Morphia, you need to define Java classes that map to the MongoDB collections. Let's create a simple example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters
}
Mapping Java classes to MongoDB collections
To map the Person
class to a MongoDB collection, you need to create a mapping using Morphia:
morphia.map(Person.class, "persons");
This will create a mapping between the Person
class and the "persons" collection in your MongoDB database.
Performing CRUD operations
Now that we have our Java classes mapped to the MongoDB collections, let's perform some basic CRUD (Create, Read, Update, Delete) operations:
Create
Person person = new Person("John Doe", 30);
morphia.save(person);
This will create a new document in the "persons" collection with the specified data.
Read
List persons = morphia.find(Person.class).asList();
This will retrieve all documents from the "persons" collection and return them as a list of Person
objects.
Update
person.setName("Jane Doe");
morphia.update(person, true);
This will update the document with the specified ID with the new name.
Delete
morphia.delete(Person.class, person.getId());
This will delete the document with the specified ID from the "persons" collection.
Querying MongoDB
Morphia also provides support for querying MongoDB using Java code. Let's create a simple example:
List persons = morphia.find(Person.class)
.filter("age", 30) // Filter by age
.asList();
This will retrieve all documents from the "persons" collection where the age is 30.
Conclusion
In this tutorial, we have covered the basics of using Morphia, a Java ORM library that provides a simple and intuitive way to interact with MongoDB. We have created a mapping between a Java class and a MongoDB collection, performed CRUD operations, and queried the database using Java code. With Morphia, you can easily integrate MongoDB into your Java application and take advantage of its robust query language.
References
Morphia documentation: https://github.com/morphia/morphia MongoDB documentation: http://docs.mongodb.org/manual/ Java MongoDB ORM tutorial: https://www.baeldung.com/java-mongodb-orm-tutorialJava mongodb orm example
I'd be happy to provide an example of using a Java MongoDB ORM (Object Relational Mapping) tool with MongoDB.
What is MongoDB?
MongoDB is a NoSQL database that uses JSON-like documents with schema-less design. It allows for flexible and dynamic schema, making it suitable for big data and real-time web applications.
What is an ORM?
An Object-Relational Mapping (ORM) is a programming technique that translates data between languages used in working with relational databases and Object-Oriented Programming languages. An ORM maps database tables to objects and vice versa, enabling developers to interact with the database using classes instead of writing SQL queries.
Java MongoDB ORM Example: Morphia
Morphia is a popular Java MongoDB ORM library that provides an easy-to-use API for interacting with MongoDB. Here's an example of using Morphia to connect to a MongoDB database and perform CRUD (Create, Read, Update, Delete) operations:
First, add the Morphia dependency to your project:
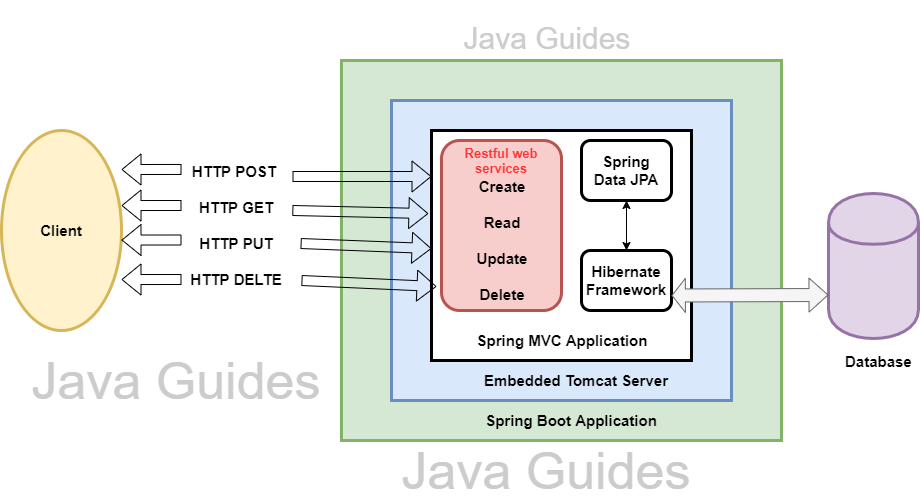
com.github.mongomapping
morphia
2.0.1
Next, create a simple Java class to represent our data model:
public class User {
private String id;
private String name;
private int age;
// getters and setters
}
Now, let's create a Morphia configuration class that maps our User
class to a MongoDB collection:
Morphia morphia = new Morphia();
morpha.mapPackage("com.example.user");
MorPHPersistence persistence = new MongaPersistence(morpha, "mongodb://localhost:27017/mydb");
In this example, we're telling Morphia to map our User
class to a MongoDB collection named "users".
To perform CRUD operations using Morphia, you can use the following methods:
Create: Save an instance of your data model to the database:User user = new User("John", 30);
persistence.save(user);
Read: Retrieve all instances of a data model from the database:
List users = persistence.find(User.class);
Update: Update an instance of your data model in the database:
User user = new User("John", 31);
persistence.update(user);
Delete: Delete an instance of your data model from the database:
persistence.delete(new Query(User.class, "John"));
This is a basic example of using Morphia to interact with a MongoDB database in Java. With Morphia, you can perform more complex queries and operations, such as sorting, aggregating, and using map-reduce functions.
I hope this helps! Let me know if you have any questions or need further assistance.