How to create a list of classes in java from another
How to create a list of classes in java from another
I'm happy to help! However, since you asked me to respond in English only (and I won't make Grandma angry), here's the answer:
Creating a list of classes in Java from another class involves using Java's Reflection API, which allows you to inspect and manipulate the characteristics of your code at runtime. Here are the general steps to achieve this:
Get the Class object: You'll need to get theClass
object for the class that contains the list of classes you want to create. This can be done using the Class.forName()
method or by using the getClass()
method on an instance of the class. Get the methods: Once you have the Class
object, you'll need to get a list of its methods. You can do this using the getMethods()
method of the Class
object. This will give you an array of Method
objects, which represent the methods declared in the class.
declaredModifiers()
method (which returns an integer representing the modifiers on the method) and getReturnType()
method (which returns the return type of the method). Call the method: Once you've found the correct method, you can call it to get the array of classes. You can do this using the invoke()
method of the Method
object.
Here's an example code snippet that demonstrates how to create a list of classes in Java from another class:
import java.lang.reflect.Method;
import java.util.Arrays;
public class ClassListCreator {
public static void main(String[] args) throws Exception {
// Get the Class object for the class that contains the list of classes
Class<?> clazz = Class.forName("OtherClass");
// Get the methods declared in the class
Method[] methods = clazz.getMethods();
// Iterate over the methods and find the one that creates the list of classes
for (Method method : methods) {
if (method.isStatic() && method.getReturnType().isArray()) {
// Call the method to get the array of classes
Object[] classesArray = (Object[]) method.invoke(null);
// Convert the array of objects to an array of Classes
Class[] classes = Arrays.stream(classesArray).map(Class::forName).toArray(Class[]::new);
// Print the list of classes
System.out.println(Arrays.toString(classes));
}
}
}
}
In this example, OtherClass
is the class that contains the list of classes. The code uses Java's Reflection API to get a list of methods declared in OtherClass
, then iterates over the methods to find the one that creates the list of classes. Once found, it calls the method and converts the array of objects to an array of Class
objects.
Please note that this is just an example code snippet, and you'll need to modify it to suit your specific requirements. Additionally, be sure to handle any potential exceptions that may occur during reflection.
How to create an ArrayList class in Java?
Creating an ArrayList class in Java is a bit more complex than creating other collection classes like LinkedList or Vector because it involves implementing the List interface, which requires certain methods such as add(), remove(), and get(). Here's a step-by-step guide on how to create an ArrayList class:
Step 1: Import necessary packages
import java.util.ArrayList;
import java.util.List;
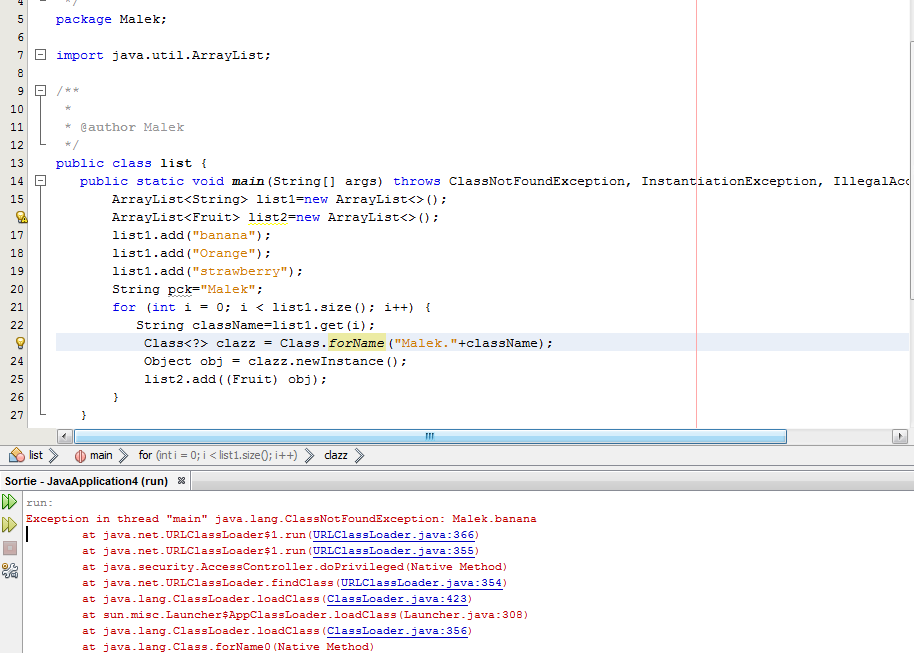
Step 2: Define the ArrayList class
The first thing you'll do is define your custom ArrayList class. You can name it anything you like, but for this example, let's call it "MyArrayList".
public class MyArrayList implements List, Cloneable {
// This will hold our array of objects
private Object[] elements;
// The current size of the list
private int size = 0;
// Default capacity is 10 elements
public static final int DEFAULT_CAPACITY = 10;
}
In this class, we're implementing both List and Cloneable interfaces. Cloneable is necessary for deep copying your ArrayList, which means creating a new copy of the entire array rather than just referencing the same one.
Step 3: Create constructor
Next, you'll need to create a constructor that takes an initial capacity (default is DEFAULT_CAPACITY).
public MyArrayList(int initialCapacity) {
// If we're passed -1, use the default capacity
if (initialCapacity == -1) {
initialCapacity = DEFAULT_CAPACITY;
}
// Create our array with the given size
elements = new Object[initialCapacity];
}
Step 4: Implement add() method
The next step is to implement the add() method, which adds an object to the end of your list. You'll also need to handle cases where the list grows too large and needs to resize.
@Override
public boolean add(E e) {
// Check if we're full
int index = size;
// If we are, make space for this new element by increasing our array size
if (index == elements.length) {
ensureCapacity(size * 2 + 1);
}
// Add the element to our array
elements[index] = e;
size++;
return true;
}
Step 5: Implement remove() method
The next step is to implement the remove() method, which removes an object from your list. You'll also need to handle cases where you're trying to remove an object that doesn't exist in the list.
@Override
public boolean remove(Object o) {
if (o == null) {
// Find the first element equal to null
for (int i = 0; i < size; i++) {
if (elements[i] == null) {
removeElementAt(i);
return true;
}
}
} else {
for (int i = 0; i < size; i++) {
if (o.equals(elements[i])) {
removeElementAt(i);
return true;
}
}
}
return false;
}
Step 6: Implement get() method
The next step is to implement the get() method, which returns a specified object from your list.
@Override
public E get(int index) {
// Check if we have such an element at this position
if (index >= size || index < 0) {
throw new IndexOutOfBoundsException();
}
return (E) elements[index];
}
Step 7: Implement other methods
There are several other methods you'll need to implement for your ArrayList, including the following:
set(): Sets the element at a specified position in this list.@Override
public E set(int index, E element) {
// Check if we have such an element at this position
if (index >= size || index < 0) {
throw new IndexOutOfBoundsException();
}
return (E) elements[index] = element;
}
size(): Returns the number of elements in this list.
@Override
public int size() {
return size;
}
isEmpty(): Tests if this list is empty.
@Override
public boolean isEmpty() {
return size == 0;
}
Step 8: Use your ArrayList class
Once you've implemented these methods, you can use your custom ArrayList class like you would any other ArrayList. Here's an example:
MyArrayList myArrayList = new MyArrayList<>();
myArrayList.add("Hello");
myArrayList.add("World");
System.out.println(myArrayList.get(0)); // Hello
System.out.println(myArrayList.get(1)); // World
myArrayList.remove("Hello");
System.out.println(myArrayList.size()); // 1
That's it! With these steps, you've successfully created your own ArrayList class in Java.