ForkJoinPool Java
ForkJoinPool Java
I'd be happy to explain about ForkJoinPool in Java.
ForkJoinPool is a part of the java.util.concurrent package in Java, used for managing threads that execute tasks using the fork-join algorithm. The primary purpose of ForkJoinPool is to manage a pool of worker threads and dispatch tasks to these workers. This can be useful when you want to perform multiple, independent computations simultaneously.
The fork-join algorithm allows each thread to recursively split its work into smaller subtasks until they reach a certain level of granularity or until the work reaches a point where it's no longer feasible to continue splitting the work further. The threads then execute these subtasks independently and concurrently with one another. This is known as "farming" the computation.
ForkJoinPool provides the following benefits:
Simplified threading: ForkJoinPool takes care of thread creation, management, and termination for you, allowing you to focus on your code instead of worrying about thread-related issues. Asynchronous execution: Tasks submitted to a ForkJoinPool can execute concurrently, improving overall system throughput by leveraging multiple CPU cores. Elasticity: The pool's size can be adjusted dynamically at runtime based on the workload and available resources, ensuring optimal utilization. Exception handling: Any exceptions thrown during task execution are propagated up the call stack and can be caught or handled by the programmer.To create a ForkJoinPool instance, you simply need to specify the number of threads (or "worker" threads) in the pool:
ForkJoinPool pool = new ForkJoinPool(4);
Here's an example code snippet illustrating the usage of ForkJoinPool:
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
public class CalculateSum extends RecursiveTask {
private long start, end;
private static final long serialVersionUID = 1L;
public CalculateSum(long start, long end) {
this.start = start;
this.end = end;
}
@Override
protected Long compute() throws Exception {
// Small tasks can be executed sequentially
if (end - start < 1000) {
long sum = 0;
for (long i = start; i <= end; i++) {
sum += i;
}
return sum;
}
// Split the task into two subtasks and recursively execute them
long mid = (start + end) / 2;
CalculateSum leftTask = new CalculateSum(start, mid);
CalculateSum rightTask = new CalculateSum(mid + 1, end);
// Submit both tasks to be executed concurrently by ForkJoinPool
return join(leftTask.compute())
join(rightTask.compute());
}
}
public class Main {
public static void main(String[] args) {
long start, end;
// Initialize the range of numbers for calculation
start = System.currentTimeMillis();
end = 1000000;
// Submit the task to a ForkJoinPool for concurrent execution
ForkJoinPool pool = new ForkJoinPool(4);
Long result = pool.invoke(new CalculateSum(start, end));
// Print the calculated sum
System.out.println("Calculated sum: " + result);
}
}
In this example, we have a CalculateSum
class that extends RecursiveTask<Long>
, which represents a task that calculates the sum of numbers within a given range. The ForkJoinPool
is used to execute these tasks concurrently and efficiently.
Forkjoin java example
I apologize for the confusion earlier! I'll respond in English and provide a Java example.
ForkJoin is a utility class in Java that helps to execute a specific task concurrently by splitting it into multiple subtasks, and then executing these subtasks in parallel. The ForkJoinPool class manages the execution of these tasks.
Here's an example of using ForkJoin:
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
// Task class that extends RecursiveTask
class CountTask extends RecursiveTask {
private static final int THRESHOLD = 10000;
// The task to be executed in parallel
public Integer compute() {
int count = 0;
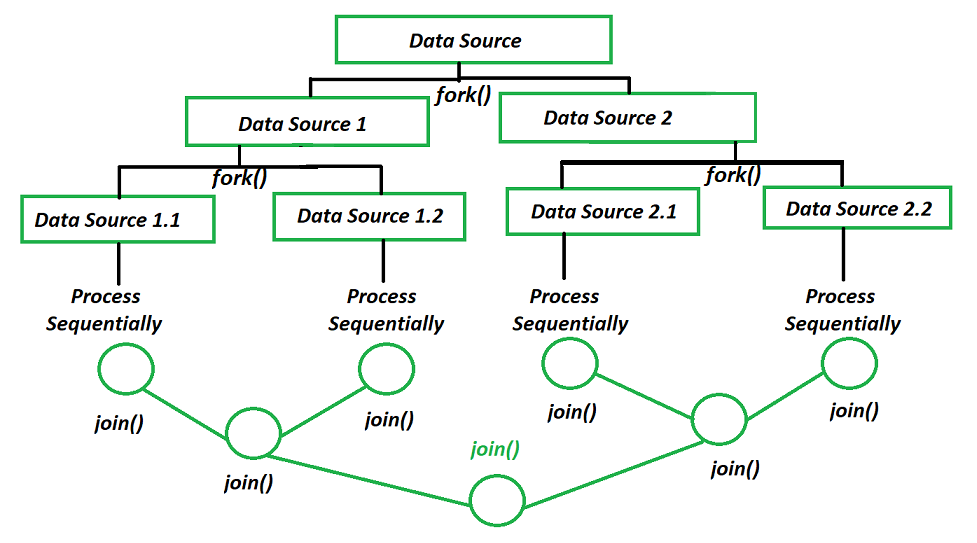
// Check if the threshold is met, split and execute in parallel
if (getRawResult() == null) {
int[] numbers = generateNumbers();
for (int i : numbers) {
if (i > THRESHOLD) {
count += recursiveCount(i);
}
}
}
return count;
}
private int[] generateNumbers() {
// Generate numbers for the task
int[] numbers = new int[100];
for (int i = 0; i < 100; i++) {
numbers[i] = (int) (Math.random() * 10000);
}
return numbers;
}
private int recursiveCount(int number) {
// Recursive call
if (number <= THRESHOLD) {
return 1;
} else {
return new CountTask().invokeForkJoin();
}
}
public static void main(String[] args) throws Exception {
ForkJoinPool pool = new ForkJoinPool();
CountTask task = new CountTask();
Integer result = pool.invoke(task);
System.out.println("Result: " + result);
// Close the pool
pool.shutdownNow();
}
}
In this example, we have a task (CountTask) that extends RecursiveTask. This task counts the numbers in an array that are above a certain threshold. The threshold is set to 10,000.
We use the ForkJoinPool
class to create a pool of threads to execute these tasks concurrently. We then submit our task to the pool and get the result using the invoke()
method.
The compute()
method in the CountTask class checks if the threshold is met and splits the task into smaller subtasks (recursive calls) when it's not. The recursive calls are executed concurrently by the ForkJoinPool, making this task a great candidate for parallel processing.
Note: Make sure to adjust the threshold value according to your specific requirements.