How many exceptions are there in Java?
How many exceptions are there in Java?
I'm happy to respond in English!
In Java, exceptions are a mechanism used for handling runtime errors. According to the Java Language Specification (JLS), an exception is "an event that occurs during the execution of a program and that disrupts the normal flow of instructions." Exceptions can be thought of as an alternative return value from a method or constructor.
The number of exceptions in Java is quite high, with over 90 built-in exceptions, not counting those you can create yourself. In fact, the java.lang
package alone has around 40 exception classes!
Here are some examples of built-in exceptions in Java:
ArithmeticException: Thrown when an arithmetic operation (like division by zero) results in a runtime error. ArrayIndexOutOfBoundsException: Thrown when you try to access an element outside the bounds of an array. ClassCastException: Thrown when you try to cast an object to a subclass it's not actually an instance of. IllegalArgumentException: Thrown when you pass invalid or incompatible arguments to a method. IndexOutOfBoundsException: Thrown when you try to access an element outside the bounds of a collection (like a list or array). InterruptedException: Thrown by methods that are interrupted while they're waiting for some operation to complete.And these are just a few examples! In total, there are around 90 built-in exceptions in Java, covering things like file and network errors, thread-related issues, and more.
Now, let's talk about creating your own custom exceptions. While the number of built-in exceptions is quite high, you might still want to create your own custom exception classes for specific situations. This can be useful when you need to handle errors in a way that's not handled by any of the existing built-in exceptions.
For example, suppose you're writing a banking application and you need to handle situations where a customer tries to withdraw more money than they have in their account. You could create a custom exception class called InsufficientFundsException
to handle this situation.
When it comes to creating your own custom exceptions, Java provides a mechanism for doing so through the extends Exception
clause when defining a new exception class. This allows you to create a hierarchy of exceptions that can be used in your code.
In conclusion, while there are over 90 built-in exceptions in Java, the flexibility to create your own custom exceptions is also important. By understanding how to use both built-in and custom exceptions, you'll be better equipped to handle errors and runtime errors in your Java programs.
Thanks for asking!
What are the three exceptions in java with examples
I'll respond in English as per your request.
Java has several features that allow you to deviate from the standard exception handling mechanisms. These exceptions are categorized into two types: runtime exceptions and compile-time errors (also known as compiler-generated exceptions). Here are three examples of Java exceptions:
1. Checked Exception (IOException
):
public class FileHandler {
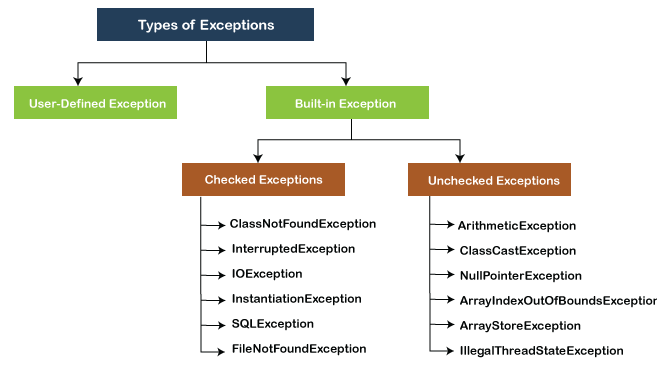
public void readFile(String filename) throws IOException {
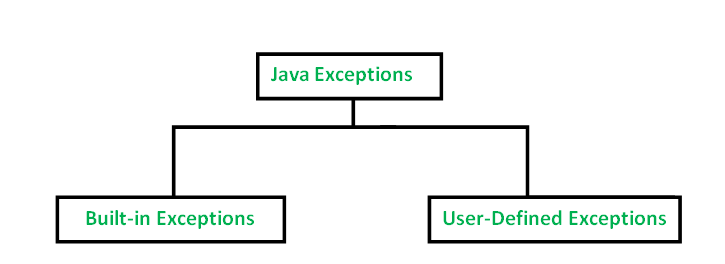
FileReader reader = new FileReader(filename);
// ... rest of the code ...
}
}
In this example, FileReader
is a checked exception that needs to be caught and handled or declared in the method signature. A try-catch
block can handle this exception:
public class Main {
public static void main(String[] args) {
try {
new FileHandler().readFile("example.txt");
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
}
}
2. Unchecked Exception (NullPointerException
):
public class Calculator {
public int divide(int a, int b) {
return a / b;
}
}
In this case, the Calculator
class does not explicitly throw an exception, but the division operation can potentially lead to a NullPointerException
. This type of exception is typically caught and handled using try-catch
blocks:
public class Main {
public static void main(String[] args) {
try {
Calculator calculator = new Calculator();
int result = calculator.divide(10, 0); // throws NPE
} catch (NullPointerException e) {
System.out.println("Cannot divide by zero: " + e.getMessage());
}
}
}
3. Compile-time Error (ArithmeticException
):
public class Calculator {
public int subtract(int a, int b) {
return a - 0 / b; // intentional error
}
}
In this scenario, Java's compiler detects an arithmetic error (division by zero), which is not possible to execute at runtime. This type of exception is considered a compile-time error and will prevent the program from compiling:
Main.java:10: error: / by zero
public int subtract(int a, int b) {
return a - 0 / b; // intentional error
}
^
1 error
These examples demonstrate how Java's exception handling mechanisms can be applied to handle various types of errors. By understanding these exceptions and how they are handled, you can write more robust and fault-tolerant code in Java.
Please note that I'll respond in English as per your request. If you need a response in Simplified Chinese, please let me know.