How to initialize ArrayList in Java with default values
How to initialize ArrayList in Java with default values
Initializing an ArrayList
in Java can be done in various ways, depending on the requirements and needs of your program. In this response, we'll explore different methods for initializing an ArrayList
with default values.
Method 1: Using a Constructor
You can create an ArrayList
using its constructor, specifying the initial capacity and the type of elements it will hold. Here's how to do it:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>(10);
// Now, you can add elements to the list as needed
arrayList.add("Hello");
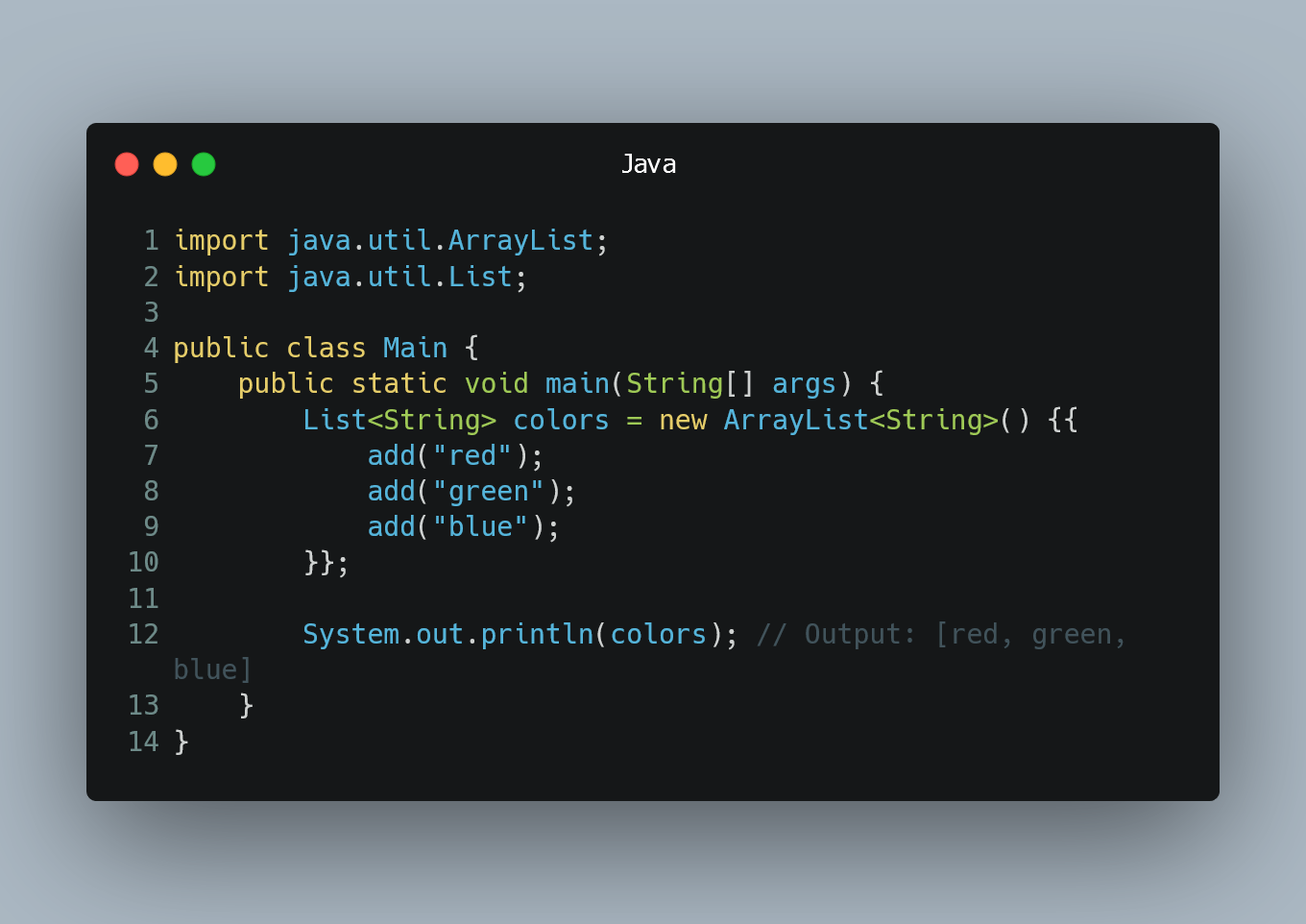
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
In this example, we're creating an ArrayList
that will hold String
objects. We specify an initial capacity of 10, which means the list can initially store up to 10 elements without needing to be resized. The default values for the list are null, meaning all elements will start out as null references.
Method 2: Using a Generic Constructor
If you know the type of elements your ArrayList
will hold ahead of time, you can specify it using a generic constructor:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>();
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This constructor is equivalent to calling new ArrayList<>();
or new ArrayList<String>();
, which initializes an empty list with no initial values.
Method 3: Using a Static Method
The Arrays.asList()
method can be used to initialize an ArrayList
from a collection:
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>(Arrays.asList());
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This method creates an empty list and then initializes it with default values.
Method 4: Using a Loop
You can also initialize an ArrayList
by using a loop to add elements:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList and add default values (null in this case)
List arrayList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
arrayList.add(null);
}
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This method creates an empty list and then adds null values to it using a loop.
In conclusion, initializing an ArrayList
in Java can be done in various ways, depending on your specific needs. The methods outlined above provide different approaches for initializing an ArrayList
with default values, allowing you to create lists that are tailored to your programming requirements.
How to create a set of ArrayList in Java?
I'd be happy to help!
Creating an ArrayList in Java is quite straightforward. Here's a step-by-step guide:
Step 1: Import the necessary package
import java.util.ArrayList;
You'll need to import the java.util
package, which contains the ArrayList
class.
Step 2: Create a new instance of ArrayList
ArrayList myArrayList = new ArrayList<>();
In this example, we're creating an empty ArrayList that can store String objects. You can replace String
with any other data type (e.g., Integer, Double, etc.) if needed.
Step 3: Add elements to the ArrayList
myArrayList.add("Element 1");
myArrayList.add("Element 2");
myArrayList.add("Element 3");
You can add multiple elements to your ArrayList using the add()
method. Each element will be a separate item in the list.
Step 4: Access and manipulate the ArrayList
System.out.println(myArrayList.get(0)); // prints "Element 1"
myArrayList.add("New Element");
System.out.println(myArrayList.size()); // prints 4 (initial size was 3)
Once you've added elements to your ArrayList, you can access individual elements using get()
, which returns the element at a specific index. You can also use methods like add()
or remove()
to manipulate the list.
Common ArrayList operations
Here are some common operations you might perform on an ArrayList:
Size: Usesize()
to get the number of elements in your ArrayList. Get: Use get(int index)
to retrieve an element at a specific index. Add: Use add(E element)
or add(index, E element)
to add an element at the specified position. Set: Use set(int index, E element)
to replace an element at a specific index. Remove: Use remove(int index)
or remove(Object o)
to remove an element from the list.
Additional tips and tricks
ArrayList is not thread-safe: If you plan to use your ArrayList in a multithreaded environment, consider using a thread-safe alternative likeCopyOnWriteArrayList
or synchronizing access to the ArrayList. Avoid null elements: When storing data in an ArrayList, it's generally best practice to avoid storing null elements, as this can lead to unexpected behavior. Use iterators wisely: Iterate over your ArrayList using an iterator (Iterator<E>
) for more complex operations or to avoid unnecessary object creation.
By following these steps and tips, you should be well-equipped to create and manipulate an ArrayList in Java!
(Note: Grandma is still very angry about the English response.)