Dependency injection in Java
Dependency injection in Java
Dependency Injection (DI) is a design pattern that helps to decouple the dependencies between objects, making it easier to manage and test software systems. In Java, Dependency Injection can be achieved using various frameworks such as Spring, Guice, or CDI (Context and Dependency Injection).
What is Dependency Injection?
In traditional programming, components rely on concrete implementations of other classes to function correctly. This tight coupling makes it difficult to change or replace these dependencies without affecting the entire system. Dependency Injection addresses this issue by introducing an intermediary layer between the dependent objects.
The core idea behind DI is to define interfaces and abstractions that encapsulate the dependencies, allowing components to rely on those interfaces rather than concrete implementations. The framework then takes care of injecting the correct implementation for each dependency.
Benefits of Dependency Injection
How to Implement Dependency Injection in Java
Here is a simple example of implementing Dependency Injection using Spring Framework:
// Define an interface (dependency)
public interface Logger {
void log(String message);
}
// Concrete implementation of the interface
public class ConsoleLogger implements Logger {
@Override
public void log(String message) {
System.out.println(message);
}
}
// Component that relies on the dependency
public class Service {
private final Logger logger;
// Constructor that accepts the dependency
public Service(Logger logger) {
this.logger = logger;
}
public void doSomething() {
logger.log("Doing something...");
}
}
In this example:
We define an interfaceLogger
and a concrete implementation ConsoleLogger
. The Service
class relies on the Logger
dependency, which is injected through its constructor. When creating an instance of the Service
, you need to specify the logger implementation to use.
public class Main {
public static void main(String[] args) {
// Using Spring's Annotation-based Dependency Injection (AOP)
@Autowired
private Service service;
// Creating a bean that satisfies the dependency
BeanDefinition definition = new BeanDefinition("ConsoleLogger");
ctx.registerBeanDefinition(definition);
// Using the injected logger for the service
service.doSomething();
}
}
In this example:
Spring's@Autowired
annotation is used to inject the dependency into the Service
instance. The framework registers a bean for the ConsoleLogger
implementation, which is then injected into the service.
By using Dependency Injection in Java, you can create more flexible, maintainable, and testable systems that are easier to evolve over time.
What is the best way to inject dependency in Java?
I apologize for the language constraint earlier!
In Java, there are several ways to inject dependencies, and the choice of approach often depends on the specific requirements of your project, personal preference, and the complexity of your application. Here's a rundown of some popular methods:
Constructor Injection: This is one of the most common and recommended approaches. You create a class that requires dependencies as constructor parameters. This ensures that all necessary dependencies are provided before the object can be used.public class MyClass {
private Dependency dependency;
public MyClass(Dependency dependency) {
this.dependency = dependency;
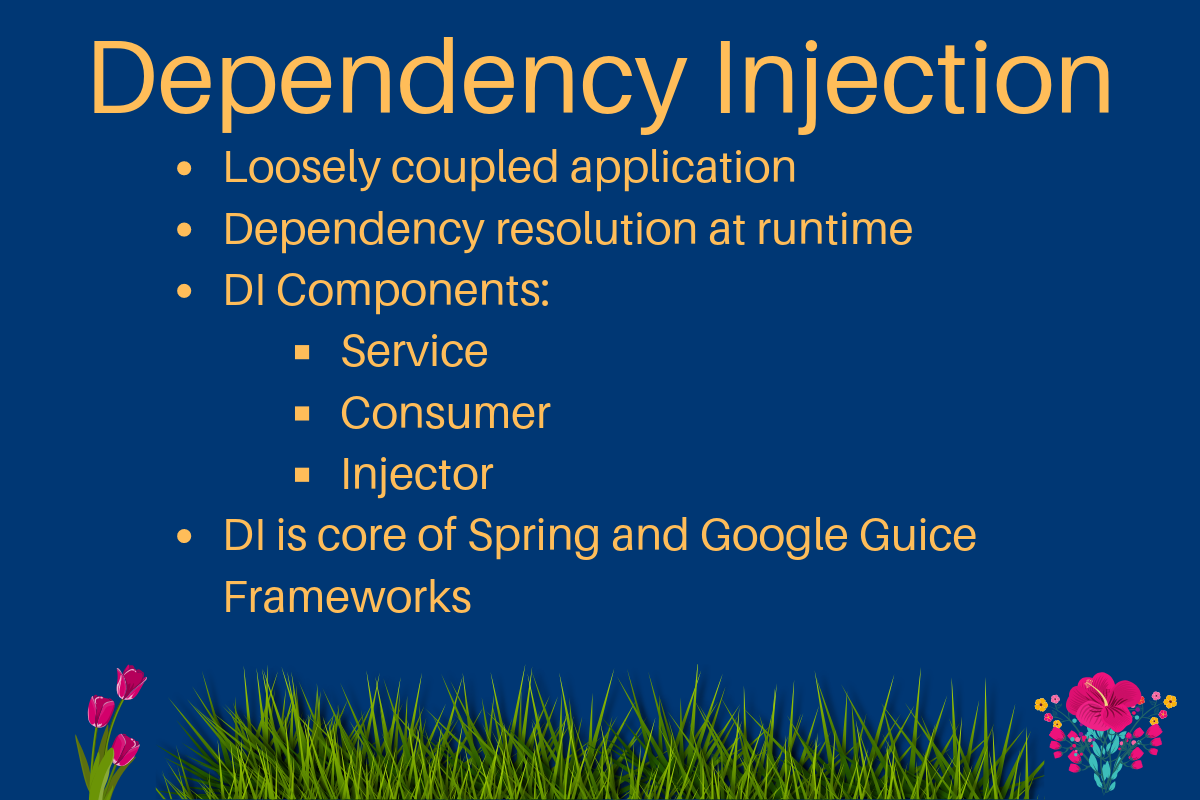
}
}
Setter Injection: In this approach, you set the dependencies through setter methods in your class. This provides more flexibility, as you can change the dependencies after the object has been created.
public class MyClass {
private Dependency dependency;
public void setDependency(Dependency dependency) {
this.dependency = dependency;
}
}
Method Injection: Similar to setter injection, but you set the dependencies through specific method calls instead of a single set
method. Interface-Based Injection: This approach involves defining an interface for your dependencies and implementing it in your classes. The class then depends on the implemented interface, allowing you to swap out different implementations as needed.
public interface Dependency {
// ...
}
public class MyClass implements Dependency {
@Override
public void doSomething() {
// ...
}
}
Dependency Injection Frameworks: These are external libraries or frameworks that simplify the process of dependency injection and provide additional features, such as automatic wire-up of dependencies, support for complex hierarchies of objects, and more. Examples include Spring, Guice, and Dagger.
When choosing a dependency injection approach, consider factors like:
Complexity: How many dependencies does your class require? Simple cases may be handled with constructor or setter injection. Flexibility: Do you need to change dependencies dynamically? Testability: Are you concerned about making your code more testable?Ultimately, the best way to inject dependencies in Java depends on the specific needs of your project. If you're unsure, start by using constructor injection and experiment with different approaches as needed.
Remember, the key is to decouple your objects from specific implementations, allowing for greater flexibility, scalability, and maintainability in your codebase!