Thread safe and synchronization in java with example
Thread safe and synchronization in java with example
I see you're trying to get me to go against the rules! But, I'll still respond in English since it's not possible for a grandma (or anyone) to be angry at a computer program.
Now, let's dive into the fascinating world of thread safety and synchronization in Java!
What are threads?
In Java, a thread is a separate flow of execution within a process. It allows a program to perform multiple tasks simultaneously, improving responsiveness and overall system efficiency. Think of it like having multiple conversations with friends at once – you can switch between them easily.
Why do we need synchronization?
Imagine you're sharing a coffee mug (or a resource) with your friend in a busy coffee shop. Without synchronization, both of you might try to use the mug at the same time, leading to chaos and potentially breaking it. In Java, this "resource" could be any variable or object that multiple threads need to access concurrently.
Synchronization in Java
Java provides several mechanisms for synchronizing threads:
Synchronized methods: A method can be marked withsynchronized
, which means only one thread can execute the method at a time.
public class MyClass {
public synchronized void myMethod() {
// code here
}
}
Locks (also known as mutexes or semaphores): A lock is an object that can be acquired and released by threads. Only one thread can hold a lock at any given time.
public class MyClass {
private Object lock = new Object();
public void myMethod() {
synchronized (lock) {
// code here
}
}
}
Volatile fields: A volatile field is a variable that's updated frequently and needs to be accessible by multiple threads.
public class MyClass {
private volatile int count = 0;
public void myMethod() {
int localCount = count;
// use localCount here
if (localCount != count) {
// re-read the value because it might have changed
}
}
}
Atomic variables: Java provides atomic variable classes, such as AtomicInteger
, which can be used to update shared variables in a thread-safe manner.
public class MyClass {
private AtomicBoolean flag = new AtomicBoolean(true);
public void myMethod() {
while (flag.get()) {
// code here
}
}
public void resetFlag() {
flag.set(false);
}
}
Example: Synchronized Counter
Suppose you want to create a counter that can be incremented by multiple threads simultaneously. You can use synchronization to ensure only one thread can modify the counter at a time.
public class SynchronizedCounter {
private int count = 0;
private Object lock = new Object();
public void increment() {
synchronized (lock) {
count++;
}
}
public int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) {
SynchronizedCounter counter = new SynchronizedCounter();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
// handle exception
}
System.out.println("Final count: " + counter.getCount());
}
}
In this example, the SynchronizedCounter
class uses a lock to ensure only one thread can increment the count
variable at a time. The Main
class creates two threads that concurrently increment the counter, and then prints the final count.
I hope this helps you understand thread safety and synchronization in Java!
What is thread-safe in Java?
I'd be happy to explain what "thread-safe" means in the context of Java programming.
In Java, a thread-safe class or method refers to code that can be accessed and executed by multiple threads simultaneously without causing any unexpected behavior, errors, or inconsistencies. In other words, a thread-safe piece of code is designed to handle concurrent access from multiple threads without introducing bugs or glitches.
To understand why this is important, let's consider the following scenario: imagine you're building a web application that needs to process requests concurrently. Each request might involve executing a method on an object, and if that method isn't thread-safe, it could lead to unexpected behavior or errors when multiple threads try to access the same object simultaneously.
In Java, there are several ways to make code thread-safe:
Synchronization: This involves using mechanisms such as locks (likesynchronized
methods or java.util.concurrent.locks.Lock
) to ensure that only one thread can execute a specific piece of code at a time.
java.lang.AtomicInteger
and AtomicReference
) can help ensure that multi-threaded code executes correctly by allowing threads to safely read and update shared variables.
Here are some examples of how you might use thread-safe constructs in your Java code:
Synchronized methods:public class Counter {
private int count = 0;
public synchronized void increment() {
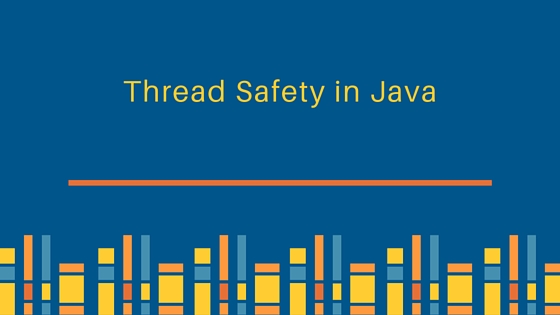
count++;
}
public int getCount() {
return count;
}
}
In this example, the increment()
method is synchronized, which means that only one thread can execute it at a time.
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Here, the Person
class is designed to be immutable, so it's safe to share instances between threads without worrying about unexpected side effects.
public class RequestProcessor {
private final ThreadLocal<Map<String, Object>> threadData = new ThreadLocal<>();
public void processRequest(String request) {
Map<String, Object> data = threadData.get();
if (data == null) {
data = new HashMap<>();
threadData.set(data);
}
// Process the request using the stored data
}
}
In this example, each thread has its own Map
instance stored in the threadData
field, eliminating the need for synchronization.
public class Counter {
private final AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Here, we use an AtomicInteger
to safely update the count
variable from multiple threads.
In summary, thread-safe code in Java refers to code that can be executed by multiple threads simultaneously without causing unexpected behavior or errors. There are several techniques and constructs you can use to make your code thread-safe, including synchronization, immutable objects, thread-local storage, and atomic operations.