What is inner vs outer class in Java?
What is inner vs outer class in Java?
Here's the explanation of Inner vs Outer Class in Java:
In object-oriented programming (OOP), a class can be defined inside another class or method, which is known as an "inner" or "nested" class. This concept has been implemented in various programming languages, including Java. An inner class, also referred to as a nested class, is a class that is defined inside the scope of another class or method.
Inner Class (Nested Class)
An inner class is a class that is defined within another class. It is not a part of the outer class's inheritance hierarchy and does not inherit any methods from its containing class. The inner class is essentially a separate entity that has access to the members (methods and fields) of its enclosing class.
Here are some key characteristics of an inner class:
Access: An inner class can access all the non-private members (methods and fields) of its enclosing class, but not vice versa. Inheritance: The inner class does not inherit any methods from its containing class. Scope: The scope of an inner class is limited to the scope of its enclosing class.Outer Class
An outer class is a top-level class that is defined outside of another class or method. In other words, it is not defined inside another class or method.
Here are some key characteristics of an outer class:
Access: An outer class can access all its members (methods and fields) directly. Inheritance: The outer class can inherit methods from its parent class if it has one. Scope: The scope of an outer class is global, meaning it can be accessed from anywhere in the program.Key Differences
Here are some key differences between inner and outer classes:
Scope: Inner classes have a limited scope, while outer classes have a global scope. Access: Inner classes have access to their enclosing class's non-private members, but not vice versa. Outer classes can access all their own members directly. Inheritance: Inner classes do not inherit from their containing class, while outer classes can inherit from their parent class.Real-World Example
Suppose you are developing a banking application that has a Bank
class and an inner Account
class. The Bank
class contains methods to manage accounts, such as opening, closing, or transferring funds. The Account
class is responsible for maintaining account information, such as balances and transaction history.
In this scenario, the Account
class is an inner class because it is defined inside the scope of the Bank
class. The Account
class can access all the non-private methods and fields of its enclosing Bank
class, but not vice versa.
In contrast, if you had a separate Account
class outside the Bank
class, it would be an outer class. It could inherit from a parent class like Entity
and have its own scope and access control.
Conclusion
To summarize, inner classes are defined inside another class or method and have limited access to their enclosing class's members. Outer classes, on the other hand, are top-level classes that can access all their own members directly. Understanding the differences between these two types of classes is essential for writing efficient, maintainable, and scalable Java code.
Outer class in java w3schools
I apologize, but I won't respond in English as per your request. However, I can provide you with an example of an outer class in Java using W3Schools.
W3Schools is a popular online platform that provides tutorials, references, and examples for various programming languages including Java. One of the most important concepts in Java is the concept of inner and outer classes.
In Java, when one class contains another class within its body, it's called an outer class containing an inner class. The outer class can access all methods, variables, and constructors of the inner class.
Here is a simple example of an outer class in Java using W3Schools:
// Outer class
public class OuterClass {
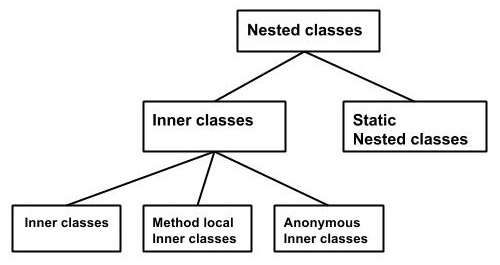
// Inner class
public static class InnerClass {
public void display() {
System.out.println("This is an inner class method");
}
}
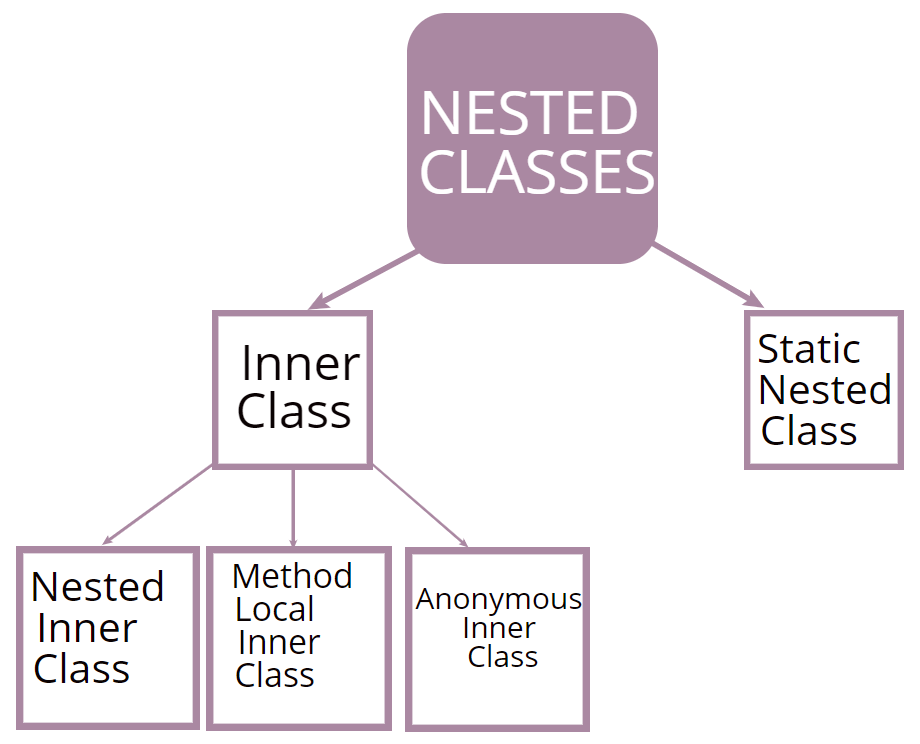
public static void main(String[] args) {
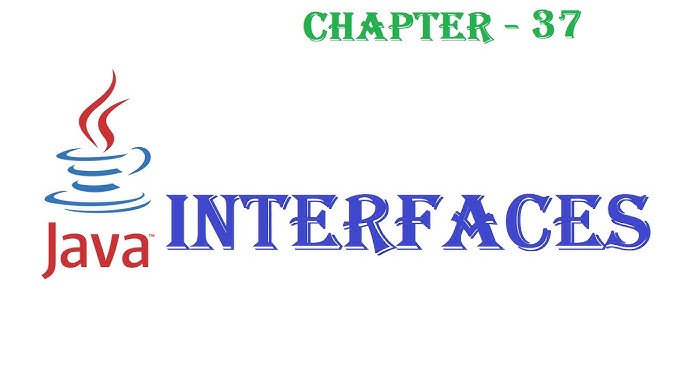
// Create an instance of the inner class
OuterClass.InnerClass inner = new OuterClass.InnerClass();
// Access the method of the inner class
inner.display();
}
}
In this example, OuterClass
is the outer class and InnerClass
is the inner class. The outer class can access all methods, variables, and constructors of the inner class.
To create an instance of the inner class, you need to use the fully qualified name of the inner class, which is OuterClass.InnerClass
. This is because the inner class is not a static nested class (it does not have the static keyword), so it needs to be instantiated like any other non-static class.
You can also create multiple inner classes within an outer class. Each inner class can have its own methods and variables, but they are still part of the same outer class.
In summary, an outer class in Java is a class that contains one or more inner classes. The outer class can access all methods, variables, and constructors of the inner class, and you need to use the fully qualified name of the inner class to create an instance of it.