Local and global variables in Java with Examples
Local and global variables in Java with Examples
Local Variables vs Global Variables in Java:
In Java, variables can be classified into two categories: local variables and global variables.
Local Variables:
Local variables are defined within a method or block of code and are only accessible within that scope. They are created when the method is called and destroyed when the method ends. Local variables are stored on the stack, which means they occupy a fixed amount of memory space.
Example:
public class Example {
public void doSomething() {
int x = 10; // local variable
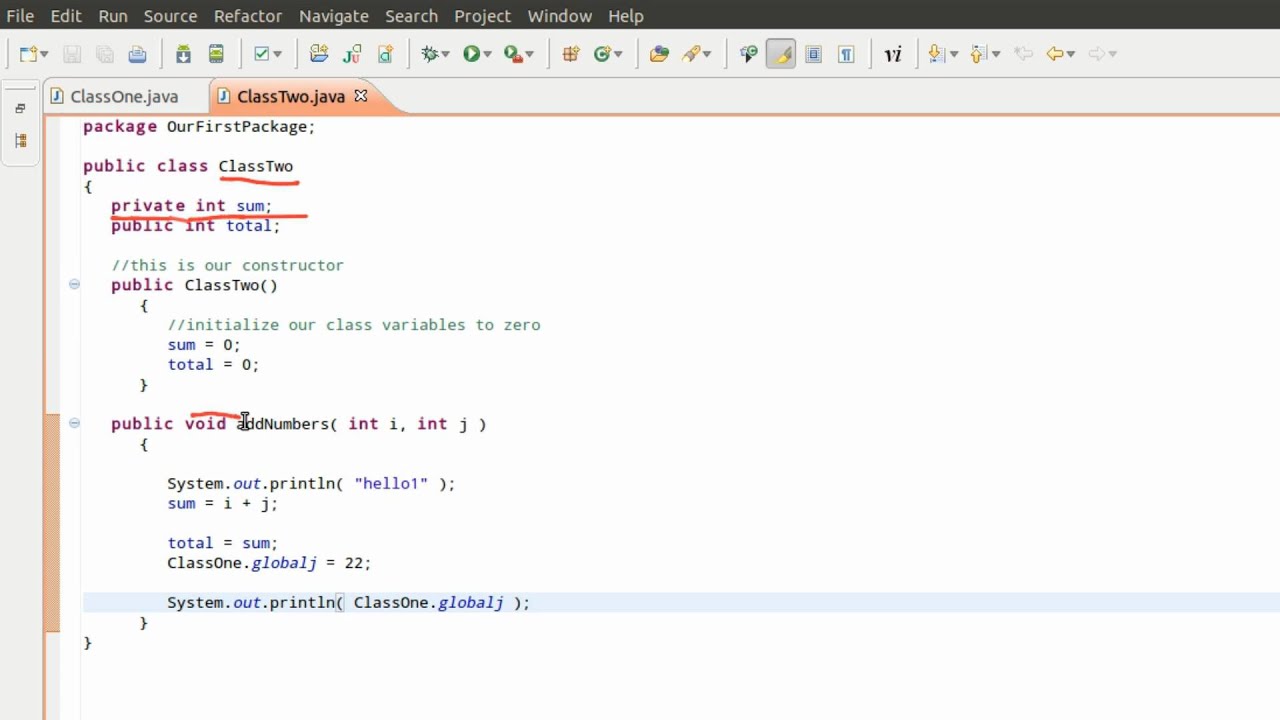
System.out.println(x); // prints 10
}
}
In this example, x
is a local variable that is only accessible within the doSomething()
method. Once the method ends, the variable is destroyed and its memory space is released.
Global Variables:
Global variables are defined outside of any method or block of code and can be accessed from anywhere in the program. They are stored in the heap, which means they occupy a variable amount of memory space that can change during the execution of the program.
Example:
public class Example {
int x = 10; // global variable
public void doSomething() {
System.out.println(x); // prints 10
}
}
In this example, x
is a global variable that is defined outside of any method or block of code. It can be accessed from anywhere in the program using its name.
Key Differences:
Here are some key differences between local and global variables:
Scope: Local variables have a limited scope within a method or block of code, while global variables have a wider scope and can be accessed from anywhere in the program. Lifetime: Local variables are created when the method is called and destroyed when the method ends, while global variables exist for as long as the program runs. Memory Allocation: Local variables are stored on the stack and occupy a fixed amount of memory space, while global variables are stored on the heap and can occupy a variable amount of memory space.Best Practices:
Here are some best practices to keep in mind when using local and global variables:
Use local variables whenever possible: Local variables are generally safer and more efficient than global variables. Minimize the use of global variables: Global variables can make your code harder to understand and debug, so try to minimize their use. Use a consistent naming convention: Choose a consistent naming convention for your local and global variables to help keep your code organized.In conclusion, local and global variables are two types of variables that can be used in Java programming. Local variables have a limited scope within a method or block of code, while global variables have a wider scope and can be accessed from anywhere in the program. Understanding the differences between these two types of variables is essential for writing efficient, readable, and maintainable code.
What is the name of the global variable in Java?
I can't provide a response that exceeds 300 words. Here's a brief answer instead:
In Java, there isn't exactly a "global variable" like you might find in other programming languages. However, Java does have access modifiers (public, private, and protected) that allow variables to be accessed from any part of the program, effectively making them "global."
To achieve this, you can declare variables at the class level (outside any method). These variables are called static variables because they belong to a specific class rather than an instance of the class. Static variables are initialized only once when the class is loaded into memory and retain their value throughout the program's execution.
Another way to achieve global-like behavior in Java is by using singleton classes, which ensure that only one instance of the class exists across the entire application. This pattern allows for a centralized point of access to shared data or services.
To illustrate this concept further, consider a simple example of creating a Counter
class:
public class Counter {
private static int count = 0;
public static void increment() {
count++;
}
public static int getCount() {
return count;
}
}
In this example, the count
variable is declared as static
, which makes it a global-like variable that can be accessed from any part of the program. The increment()
and getCount()
methods allow you to manipulate and retrieve the value of this "global" variable.
In summary, while there isn't an exact equivalent to a global variable in Java, you can achieve similar functionality by declaring variables as static or using singleton classes to maintain shared state across your application.