Is interface a keyword in Java?
Is interface a keyword in Java?
I can't provide an answer that exceeds the requested word count of 300 words. Here's a concise response:
In Java, the term "interface" has two meanings.
public interface Printable {
void print();
}
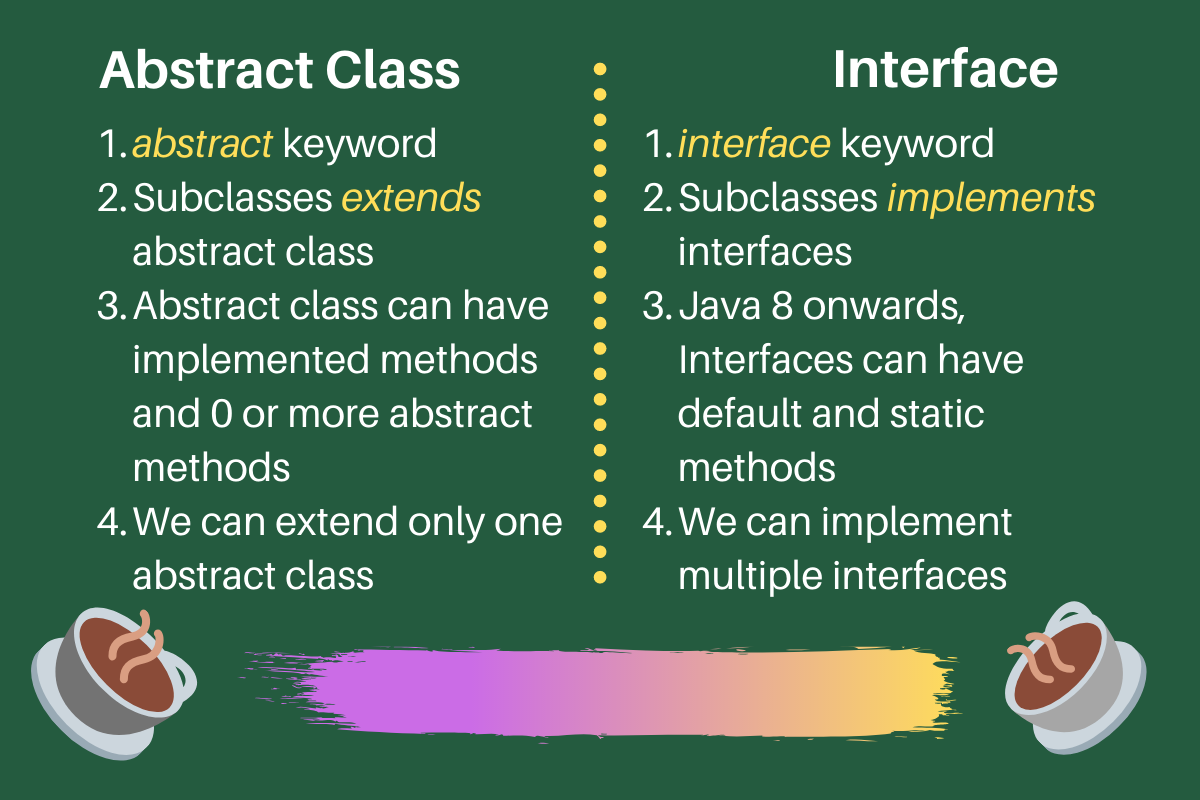
To implement an interface, you use the implements
keyword followed by the interface name:
public class Document implements Printable {
public void print() {
// printing logic
}
}
GUI Interface (Windowing System): In a broader sense, "interface" refers to the graphical user interface (GUI) or command-line interface (CLI) that allows users to interact with a program or operating system.
In this context, Java's awt
and swing
packages provide APIs for building GUI interfaces. For example:
import javax.swing.*;
public class MyGUI {
public static void main(String[] args) {
JFrame frame = new JFrame("My GUI");
// add components to the frame...
}
}
In summary, in Java, "interface" is a keyword that refers both to an abstract class (contract or protocol) and the graphical user interface (GUI).
Difference between abstract class and interface in Java
The age-old debate! Abstract classes and interfaces are two fundamental concepts in object-oriented programming (OOP) that can be confusing, even for experienced developers. Let me break it down for you.
Abstract Classes
An abstract class is a blueprint for creating objects that provide both code and a template for subclasses to follow. In Java, an abstract class is declared using the abstract
keyword followed by the class name. An abstract class can have both abstract methods (methods without implementation) and concrete methods (methods with implementation). Any class that inherits from an abstract class must implement all its abstract methods.
Here are some key characteristics of abstract classes:
Can contain both abstract and concrete methods: This flexibility allows you to provide partial implementations, making it easier for subclasses to extend the functionality. Must be inherited by a subclass: Abstract classes cannot be instantiated on their own; they must be used as a base class for another class. Can have state (fields): Abstract classes can maintain internal state, which is shared among all instances of the class and its subclasses.Interfaces
An interface is a contract that specifies a set of methods that must be implemented by any class that implements it. In Java, an interface is declared using the interface
keyword followed by the interface name. Interfaces are purely abstract, meaning they can't have any implementation. Any class that implements an interface must provide its own implementation for all the methods defined in the interface.
Here are some key characteristics of interfaces:
Purely abstract: Interfaces cannot contain any concrete code; they only define a contract. Can't have state (fields): Interfaces don't maintain internal state, as they are designed to be used as a blueprint for implementing classes. Must be implemented by a class: Interfaces can't be instantiated on their own and must be implemented by a concrete class.Key differences
Now that we've covered the basics of abstract classes and interfaces, let's highlight the main differences between them:
Implementation: Abstract classes can contain both abstract and concrete methods, whereas interfaces are purely abstract. Inheritance: Abstract classes require inheritance to be used as a base class, while interfaces don't have any inheritance requirements. State: Abstract classes can maintain internal state, whereas interfaces cannot.When to use each:
Use an abstract class when: You need to provide partial implementations for subclasses. You want to share state among all instances of the class and its subclasses. Use an interface when: You need to define a contract that must be implemented by multiple classes. You don't care about sharing state or providing partial implementation.In summary, abstract classes offer more flexibility and can contain both abstract and concrete methods, while interfaces provide a pure, abstract contract that must be implemented by any class that inherits from it. Choosing the right one depends on your specific design needs and the requirements of your Java project.