AWS RDS Proxy Java example
AWS RDS Proxy Java example
I'd be happy to help!
AWS RDS Proxy is a feature that allows you to connect your application to Amazon Aurora and other RDS database instances, using standard JDBC or ODBC protocols. It provides a scalable and reliable way to access your databases without having to worry about the underlying infrastructure.
Here's an example of how you can use AWS RDS Proxy with Java:
First, you need to add the AWS SDK for Java to your project. You can do this by adding the following dependency to your pom.xml
file (if you're using Maven):
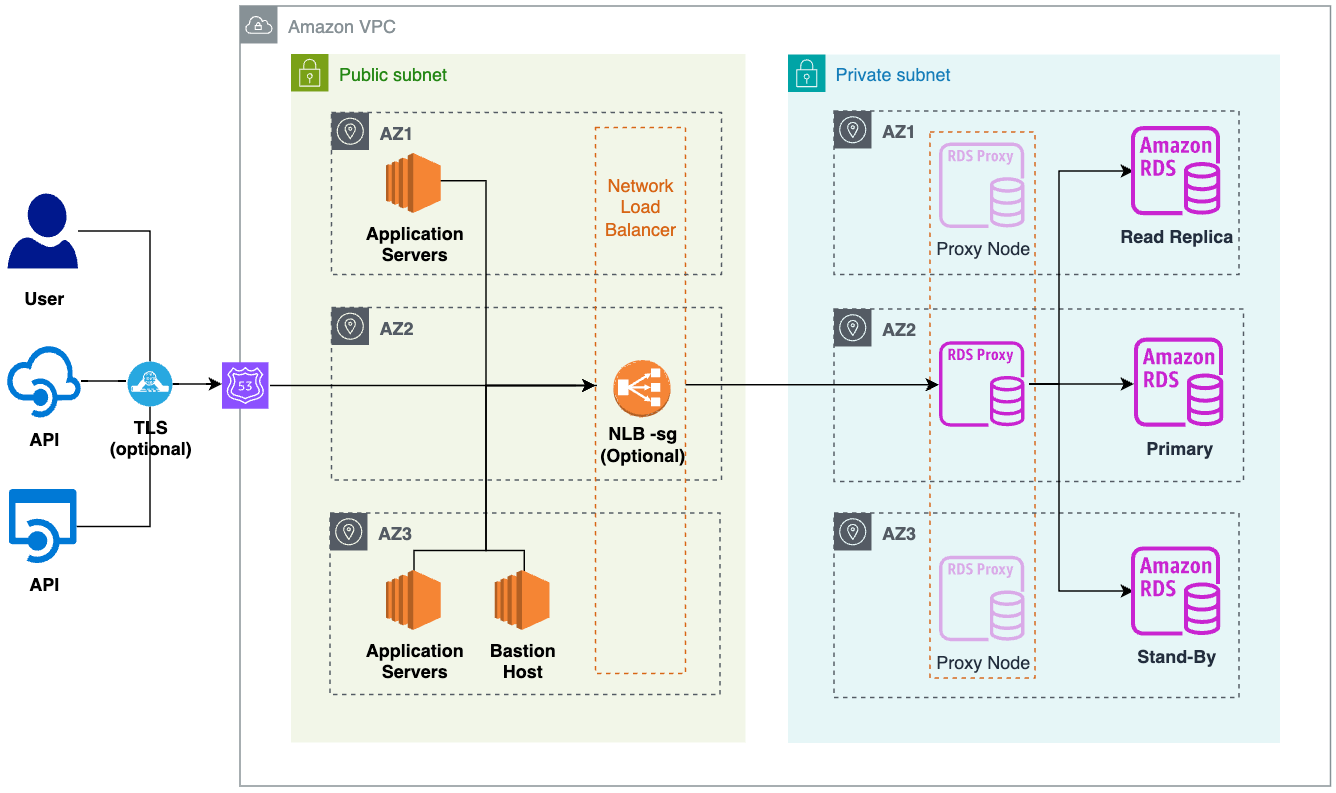
com.amazonaws
aws-java-sdk
2.17.64
Or, if you're not using a build tool like Maven, you can manually download the JAR file from the AWS SDK for Java GitHub repository and add it to your project.
Next, you need to create an RDS proxy client in your Java code. You can do this by creating a new instance of com.amazonaws.services.rdsproxy.RDSSession
and passing in the necessary configuration parameters:
RDSSession session = new RDSSession(
"my-rds-proxy", // The name of the RDS proxy
"jdbc:mysql://localhost:3306/mydb", // The JDBC URL for your database
"username", // Your database username
"password", // Your database password
10 // The maximum number of connections to keep open
);
Once you have an RDS proxy client, you can use it to connect to your database and execute SQL queries. For example:
Statement stmt = session.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
while (rs.next()) {
System.out.println(rs.getString(1) + " " + rs.getString(2));
}
This code creates a new statement object using the RDS proxy client, executes a SQL query, and then prints out the results.
You can also use the RDSSession
class to execute batch updates:
PreparedStatement pstmt = session.prepareStatement("INSERT INTO mytable VALUES (?)");
for (int i = 0; i < 10; i++) {
pstmt.setInt(1, i);
pstmt.executeUpdate();
}
This code creates a new prepared statement object using the RDS proxy client, executes a batch update of 10 rows, and then commits the changes.
Finally, don't forget to close your RDS proxy client when you're done with it:
session.close();
Here's the complete example code:
import com.amazonaws.services.rdsproxy.RDSSession;
import com.amazonaws.services.rdsproxy.model.DBInstanceStatusInfo;
import com.amazonaws.services.rdsproxy.model.DBParameterGroup;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class RDSProxyExample {
public static void main(String[] args) {
// Create an RDSSession instance
RDSSession session = new RDSSession(
"my-rds-proxy", // The name of the RDS proxy
"jdbc:mysql://localhost:3306/mydb", // The JDBC URL for your database
"username", // Your database username
"password", // Your database password,
10 // The maximum number of connections to keep open
);
try {
// Execute a SQL query
Statement stmt = session.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
while (rs.next()) {
System.out.println(rs.getString(1) + " " + rs.getString(2));
}
// Execute batch updates
PreparedStatement pstmt = session.prepareStatement("INSERT INTO mytable VALUES (?)");
for (int i = 0; i < 10; i++) {
pstmt.setInt(1, i);
pstmt.executeUpdate();
}
} catch (SQLException e) {
System.out.println("Error: " + e.getMessage());
} finally {
// Close the RDSSession instance
session.close();
}
}
}
I hope this helps! Let me know if you have any questions or need further assistance.
How to connect java with aws mac
I'd be happy to help! Here's a step-by-step guide on how to connect Java with AWS (Amazon Web Services) on Mac:
Prerequisites:
Java Development Kit (JDK) installed on your Mac. Eclipse or any other Integrated Development Environment (IDE) of your choice. AWS Access Key ID and Secret Access Key: You need to have an AWS account and generate the access key pair. Follow this link for instructions: https://docs.aws.amazon.com/general/latest/gr/authenticating-as-an-aws-user.html Amazon S3 bucket: Create an Amazon S3 bucket where you'll store your files.Step 1: Set up Eclipse with AWS SDK
Download and install the AWS SDK for Java from this link: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/getting-started.html Extract the downloaded zip file to a directory of your choice. Open Eclipse, go to File > New Project..., select Java as the project type, and name it (e.g., "AWS-S3-Upload"). In the new project directory, create a folder named "aws-sdk-java" and copy the extracted SDK files into this folder. Right-click on your Eclipse project and choose Add External JARs..., then select all the SDK jar files from the "aws-sdk-java" folder.Step 2: Configure AWS Credentials in Eclipse
In Eclipse, go to Window > Preferences. Navigate to AWS SDK for Java > Credentials Provider and click Add. Select Environment Variables as the credential provider type. Set the environment variables:AWS_ACCESS_KEY_ID
to your AWS Access Key ID AWS_SECRET_ACCESS_KEY
to your AWS Secret Access Key
Step 3: Configure S3 Client in Java
Create a new Java class (e.g., "S3Uploader") and add the following code:import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
public class S3Uploader {
public static void main(String[] args) {
// Create an S3 client instance
S3Client s3Client = S3Client.create();
// Upload a file to the S3 bucket
PutObjectRequest request = PutObjectRequest.builder()
.bucket("your-bucket-name")
.key("example.txt")
.build();
// Upload the file using the S3 client
RequestBody requestBody = RequestBody.fromString("Hello, World!");
s3Client.putObject(request, requestBody);
}
}
Replace "your-bucket-name"
with your actual AWS S3 bucket name.
Step 4: Run Your Java Program
Right-click on theS3Uploader
class and choose Run As > Java Application. The program will upload a file named "example.txt" to your specified S3 bucket.
That's it! You've successfully connected Java with AWS using Eclipse and uploaded a file to an Amazon S3 bucket.