Java heap tuning
Java heap tuning
Java Heap Tuning:
The Java Virtual Machine (JVM) provides various mechanisms for tuning the garbage collection (GC) and heap settings to optimize memory usage and application performance. Heap tuning involves adjusting the JVM's heap size, young generation, survivor spaces, and other settings to achieve optimal GC performance.
Why is heap tuning important?
Heap tuning is crucial because:
Memory efficiency: Properly tuned heaps reduce memory waste and fragmentation, leading to more efficient memory usage. Performance: Optimized GC reduces pause times, which improves application responsiveness and overall system performance. Scalability: Well-tuned heaps enable large-scale Java applications to run smoothly on multiple machines or distributed environments.Key JVM heap settings:
-Xmx (Maximum Heap Size): Set the maximum heap size in bytes. -XX:NewSize (Young Generation Size): Determine the initial size of the young generation, where objects are first allocated. -XX:SurvivorRatio: Control the ratio of Eden space to Survivor space for promoting objects from the young generation. -XX:MaxTenuringThreshold: Specify the maximum number of garbage collections before an object is promoted to the old generation.Heap tuning strategies:
Monitor and analyze GC logs: Use tools like VisualVM, GC Logs, or Java Mission Control to inspect GC activity, pause times, and heap utilization. Start with default settings: Begin with the JVM's default heap settings (e.g., -Xmx1g, -XX:NewSize4m) and adjust as needed. Increase heap size incrementally: Gradually increase the maximum heap size until GC pauses or memory usage becomes excessive. Experiment with different GC algorithms: Try various GC algorithms (e.g., Concurrent Mark-and-Sweep (CMS), Garbage-First (G1), or the low-pause-time G1) to find the most suitable one for your application.Example JVM heap settings:
java -Xmx2048m -XX:NewSize=256m -XX:SurvivorRatio=6 -XX:MaxTenuringThreshold=0 MyJavaApplication
Additional tips:
Test and profile: Verify the impact of changed heap settings on application performance using profiling tools like VisualVM or YourKit. Monitor system resources: Track CPU, memory, and network usage to ensure heap tuning doesn't negatively affect overall system performance. Consider heap-less JVMs: For applications requiring large amounts of contiguous memory, consider using a heap-less JVM, such as Zing or OpenJDK's Zero.By understanding the importance of heap tuning, identifying key JVM settings, and employing effective strategies for tuning, you can optimize your Java application's performance and scalability.
How do you tune the heap size in Java?
I'd be happy to help!
Tuning the heap size in Java is crucial for optimizing memory usage and improving program performance. The JVM (Java Virtual Machine) dynamically adjusts the heap size based on the application's memory requirements, but you can also manually configure it to achieve better results.
Why tune the heap size?
Memory consumption: A large heap size can lead to increased memory usage, which may cause issues such as out-of-memory errors or slower performance. Garbage collection: Adjusting the heap size can impact garbage collection frequency and pause times, affecting application responsiveness. Performance: An optimal heap size can improve program speed by reducing garbage collection overhead.How to tune the heap size?
There are several ways to configure the heap size in Java:
-Xmx option: Pass the-Xmx
flag followed by the desired maximum heap size (in megabytes) when running your Java application:
java -Xmx1024m YourApplication
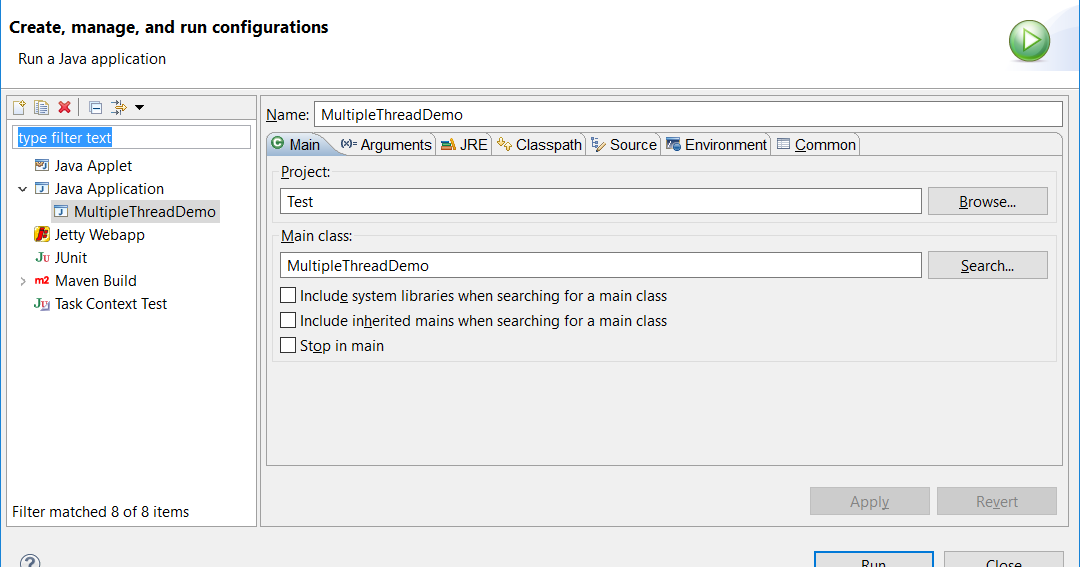
This sets the maximum heap size to 1024 MB.
-XX:NewSize option: Specify the initial and maximum heap sizes using the-XX:NewSize
flag:
java -XX:NewSize=128m -XX:MaxNewSize=256m YourApplication
This sets the initial heap size to 128 MB and the maximum heap size to 256 MB.
-Xms option: Set the minimum heap size using the-Xms
flag:
java -Xms64m YourApplication
This sets the minimum heap size to 64 MB.
Java Configuration Files (jvm.config): Edit thejvm.config
file located in the JDK's conf
directory to set the default heap size for future runs:
# conf/jvm.config
Default maximum heap size
max.heap.size=1024m
Eclipse or IntelliJ IDEA: In your IDE, go to the "Run" configurations and update the "VM arguments" to include the desired heap size settings (e.g., -Xmx512m
).
Best practices for tuning the heap size
Monitor memory usage: Use tools like VisualVM, JConsole, or YourKit to monitor your application's memory consumption and identify areas for optimization. Start with a small heap size: Begin with a smaller heap size (e.g., 128 MB) and gradually increase it as needed to avoid excessive memory usage. Monitor garbage collection: Use tools like Garbage Collector Logs or VisualVM to analyze garbage collection frequency and pause times, helping you identify optimal heap sizes. Test and iterate: Run your application with different heap size configurations and measure its performance, memory consumption, and responsiveness to find the sweet spot.In conclusion, tuning the heap size in Java requires a combination of monitoring memory usage, experimenting with different settings, and analyzing performance metrics. By following these best practices, you can optimize your application's memory usage and improve overall performance.