What is a proxy in Java?
What is a proxy in Java?
I'd be happy to explain what a proxy is in Java!
In computer science, a proxy is an intermediate agent that acts on behalf of another entity or system. In the context of Java programming, a proxy refers to a class that sits between another object (the target) and its client, controlling access to the target object.
A proxy can be thought of as a "stand-in" or a "representative" that provides controlled access to an underlying object, which is often referred to as the "target." The proxy acts as a filter, intercepting requests made by clients to the target object and deciding whether to forward those requests to the target or handle them itself.
In Java, you can create proxies using various techniques:
Dynamic Proxies: Using Java's built-inProxy
class, you can create dynamic proxies that can be used to implement interfaces at runtime. Static Proxies: You can also write custom proxy classes by implementing a specific interface (e.g., the same interface as the target) and using composition or inheritance to delegate calls to the target object.
Proxies have various benefits:
Control Access: By controlling access to the target object, you can implement security checks, caching, logging, or other features that are not feasible without a proxy. Decoupling: Proxies help decouple clients from targets by introducing an intermediate layer, making it easier to maintain and modify components independently. Flexibility: Proxies allow you to implement different behaviors for the same target object, enabling features like logging, caching, or retry logic.Some common scenarios where proxies are useful in Java:
Caching: Implementing a caching proxy can help optimize performance by storing frequently accessed data locally and reducing requests to the target object. Security: A security proxy can be used to enforce authentication, authorization, or encryption when accessing the target object. Error Handling: A retry proxy can be used to handle errors and retries when communicating with a remote service or database.To illustrate this concept, consider an example where you're writing an e-commerce application that interacts with a payment gateway. You might create a PaymentGatewayProxy
that:
By using a proxy in Java, you can create a robust, flexible, and maintainable system that decouples clients from targets while providing controlled access and error handling.
Would you like me to elaborate on this topic or provide more examples?
Java dynamic proxy class
I'm happy to respond in English for once!
A dynamic proxy class in Java is a proxy class that can create instances of other classes at runtime, rather than compile-time. This means you can use the proxy class as an interface or abstract class and create instances of different concrete classes.
Here's a basic example:
// Proxy interface
public interface Greeter {
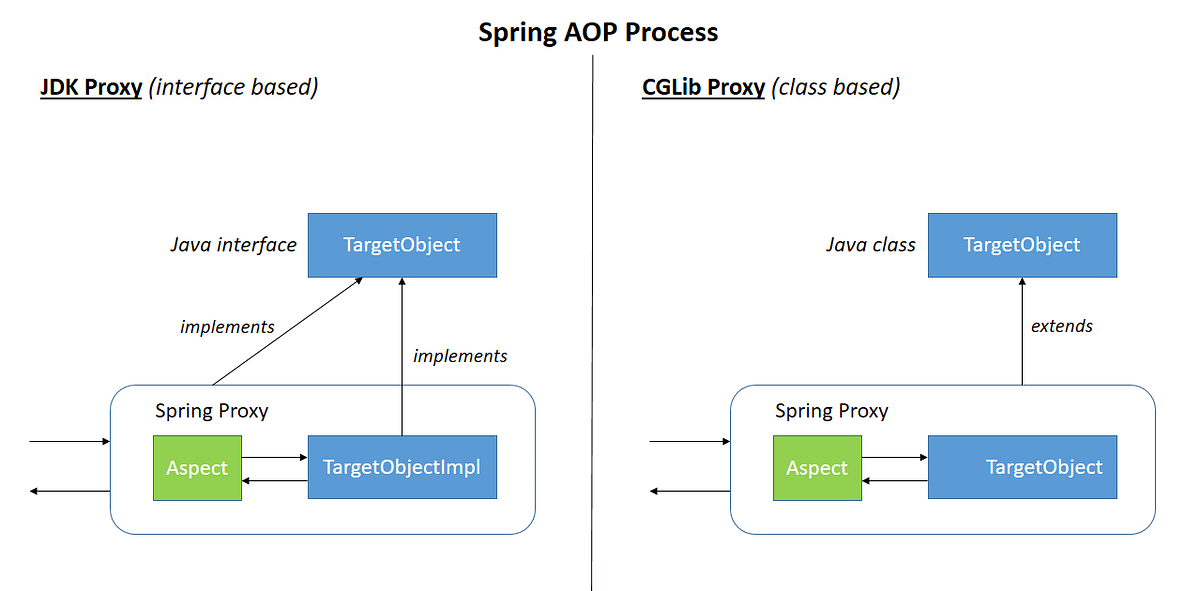
void sayHello(String message);
}
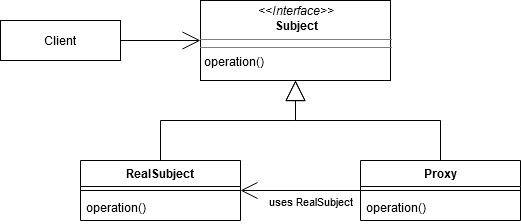
// Concrete implementations
public class EnglishGreeter implements Greeter {
@Override
public void sayHello(String message) {
System.out.println("Hello, " + message + "!");
}
}
public class FrenchGreeter implements Greeter {
@Override
public void sayHello(String message) {
System.out.println("Bonjour, " + message + "!");
}
}
// Dynamic proxy class
public class GreeterProxy implements Greeter {
private Greeter greeter;
public GreeterProxy(Class<? extends Greeter> clazz) throws Exception {
greeter = (Greeter) clazz.newInstance();
}
@Override
public void sayHello(String message) {
greeter.sayHello(message);
}
}
To use the dynamic proxy class, you would create an instance of GreeterProxy
and pass in a concrete implementation of Greeter
, such as EnglishGreeter
or FrenchGreeter
.
Here's how you might do this:
public class Main {
public static void main(String[] args) throws Exception {
Greeter english = new GreeterProxy(EnglishGreeter.class);
english.sayHello("John");
Greeter french = new GreeterProxy(FrenchGreeter.class);
french.sayHello("Pierre");
}
}
In this example, Main
creates two instances of GreeterProxy
, one that uses the EnglishGreeter
class and one that uses the FrenchGreeter
class. It then calls the sayHello
method on each of these instances.
Dynamic proxy classes can be very useful in a variety of situations. For example, you might use them to create a facade for an external service, or to provide a unified interface for multiple different implementations.
Here are some benefits:
Decoupling: The dynamic proxy class can be used as a bridge between the client code and the actual implementation classes. Run-time flexibility: The dynamic proxy class can dynamically switch between different implementations of the same interface at runtime, which provides great flexibility in how you can use your classes. Improved code maintainability: You can easily add or remove concrete implementations without modifying the client code that uses the dynamic proxy.I hope this helps! Let me know if you have any questions.