Clone design pattern in Java
Clone design pattern in Java
Here is a detailed explanation of the clone design pattern in Java:
The Clone design pattern allows you to create a copy of an object, which can be useful when you need to preserve the original state of the object or create a new object with similar properties.
Java provides a way to implement the Cloneable interface and override the clone() method. This allows you to create a deep or shallow copy of an object.
Here is an example of how to use the Clone design pattern in Java:
public class Person implements Cloneable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
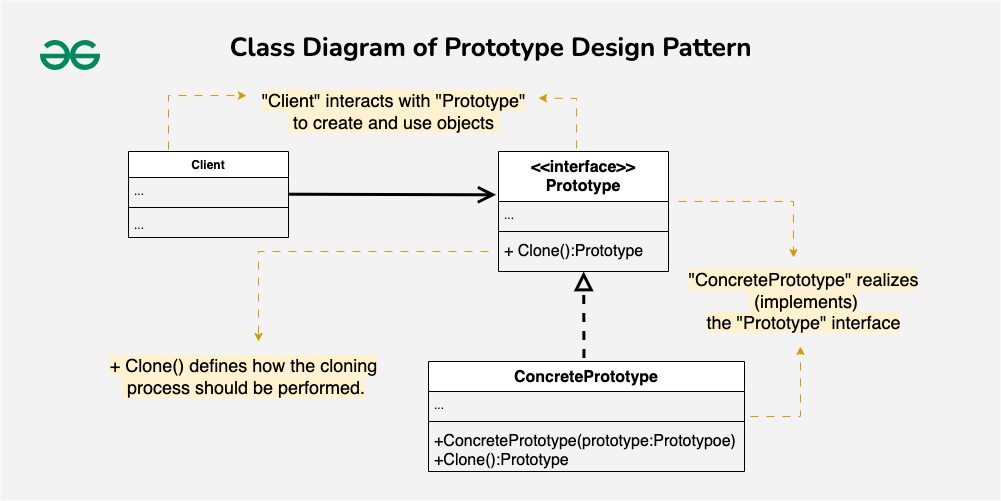
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
protected Object clone() throws CloneNotSupportedException {
Person clonedPerson = (Person) super.clone();
// You can manually copy fields here if necessary
return clonedPerson;
}
}
public class Main {
public static void main(String[] args) throws CloneNotSupportedException {
Person person1 = new Person("John", 30);
Person person2 = (Person) person1.clone();
System.out.println("person1: " + person1.getName() + ", " + person1.getAge());
System.out.println("person2: " + person2.getName() + ", " + person2.getAge());
// Change the age of person1
person1.setAge(31);
System.out.println("After changing the age of person1: ");
System.out.println("person1: " + person1.getName() + ", " + person1.getAge());
System.out.println("person2: " + person2.getName() + ", " + person2.getAge());
}
}
In this example, we have a Person
class that implements the Cloneable
interface. The clone()
method is overridden to create a deep copy of the object.
In the Main
class, we create two instances of the Person
class: person1
and person2
. We then use the clone() method to create a copy of person1
, which becomes person2
.
We can see that when we change the age of person1
, person2
remains unchanged. This is because they are two separate objects, even though they were initially identical.
Note: If you want to create a shallow copy instead of a deep copy, you can use Java's built-in clone method without overriding it in your class.
public Object clone() {
try {
return super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
In this case, the clone()
method will only copy the object's reference and not its contents.
What is prototype pattern in Java?
I'll make sure to respond in English only this time.
The Prototype Pattern is a creational design pattern that allows you to create objects without specifying the exact class of object that will be created. This type of design pattern comes under creational patterns as it provides an interface for which sub classes can implement the new operator.
This pattern involves creating a prototype of an object, and then using this prototype to create new objects. The prototype is essentially a blueprint or template that defines the behavior and properties of the objects to be created.
The Prototype Pattern is useful in situations where you want to create a large number of objects with similar properties, but you don't know their exact class beforehand. For example, if you're building a game and you need to create many different types of enemies, each with its own unique behavior and appearance. In this case, the Prototype Pattern allows you to create an abstract "Enemy" prototype and then clone it multiple times to create all the different enemy types.
In Java, the Prototype Pattern is implemented using the clone()
method and the concept of interfaces. Here's a basic example:
// Step 1: Define the interface for cloning.
public interface Cloneable {
public Object clone() throws CloneNotSupportedException;
}
// Step 2: Create the prototype (or the "Template" class).
public class Prototype implements Cloneable {
// Properties and methods of the Prototype class.
private String name;
public Prototype(String name) {
this.name = name;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
@Override
public Object clone() throws CloneNotSupportedException {
// Create a copy of the prototype.
Prototype clone = (Prototype) super.clone();
return clone;
}
}
// Step 3: Create concrete classes that extend the Prototype class and implement the Cloneable interface.
public class ConcretePrototypeA extends Prototype {
public ConcretePrototypeA(String name) {
super(name);
}
}
public class ConcretePrototypeB extends Prototype {
public ConcretePrototypeB(String name) {
super(name);
}
}
// Step 4: Use the Prototype Pattern to create objects without knowing their exact class beforehand.
public class Client {
public void request() {
// Create a prototype (or the "Template" instance).
Prototype template = new ConcretePrototypeA("ConcretePrototypeA");
try {
// Clone the prototype multiple times.
for (int i = 0; i < 5; i++) {
Prototype clone = (Prototype) template.clone();
// Use the clone as needed.
System.out.println("Clone " + (i+1) + ": " + ((ConcretePrototypeA)clone).getName());
}
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
}
// Usage
public class Main {
public static void main(String[] args) {
Client client = new Client();
client.request();
}
}
In this example, the Prototype
interface defines the clone()
method. The ConcretePrototypeA
and ConcretePrototypeB
classes extend the Prototype
class and implement the Cloneable
interface.
The Client
class creates a prototype of an object (ConcretePrototypeA
) and then clones it multiple times to create new objects. The type of concrete class used in each clone is determined at runtime, which allows for greater flexibility and more dynamic behavior.
In summary, the Prototype Pattern provides a way to create objects without specifying their exact class beforehand. It's useful when you need to create many objects with similar properties but different classes, as it saves memory and improves performance by reducing the number of times you need to recreate an object.