What is an example of an instance variable in Java?
What is an example of an instance variable in Java?
I'd be happy to help!
In Java, an instance variable, also known as a non-static field or object variable, is a variable that is defined within the scope of a class and is unique to each instance (object) created from that class.
Here's a simple example:
public class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public void sound() {
System.out.println(name + " makes a sound");
}
}
In the above code, name
is an instance variable of the Animal
class. Each time you create a new object of type Animal
, it gets its own separate copy of the name
field.
public static void main(String[] args) {
Animal cat = new Animal("cat");
Animal dog = new Animal("dog");
cat.sound(); // prints: cat makes a sound
dog.sound(); // prints: dog makes a sound
}
As you can see, when we call the sound()
method on each object (cat
and dog
), it uses its own unique value of name
. This is because each object has its own instance of the name
variable.
Here's another example:
public class Rectangle {
private int width;
private int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public void resize(int newWidth, int newHeight) {
width = newWidth;
height = newHeight;
}
public void printInfo() {
System.out.println("The rectangle is " + width + " wide and " + height + " tall");
}
}
In this code, width
and height
are instance variables of the Rectangle
class. Each time you create a new object of type Rectangle
, it gets its own separate copy of these fields.
public static void main(String[] args) {
Rectangle rect1 = new Rectangle(5, 10);
Rectangle rect2 = new Rectangle(3, 7);
rect1.resize(6, 11);
rect2.resize(4, 8);
rect1.printInfo(); // prints: The rectangle is 6 wide and 11 tall
rect2.printInfo(); // prints: The rectangle is 4 wide and 8 tall
}
Again, when we call the printInfo()
method on each object (rect1
and rect2
), it uses its own unique values of width
and height
. This is because each object has its own instance of these variables.
In summary, instance variables are used to store data that is unique to each object created from a class. They are defined within the scope of the class and are accessible through objects of that class.
What is instance variable and global variable in Java?
I'll respond in English as you've asked!
In Java, an instance variable is a member of a class that has its own copy for each object created from that class. In other words, every time you create a new instance of a class (using the new
keyword), it gets its own separate set of values for all the instance variables declared in that class.
Instance variables are also known as non-static variables or instance fields. They are stored in heap memory and are garbage collected when the object is no longer referenced. Each object has its own copy of the instance variables, which means that changes made to an instance variable within one object do not affect another object with the same class.
Here's a simple example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
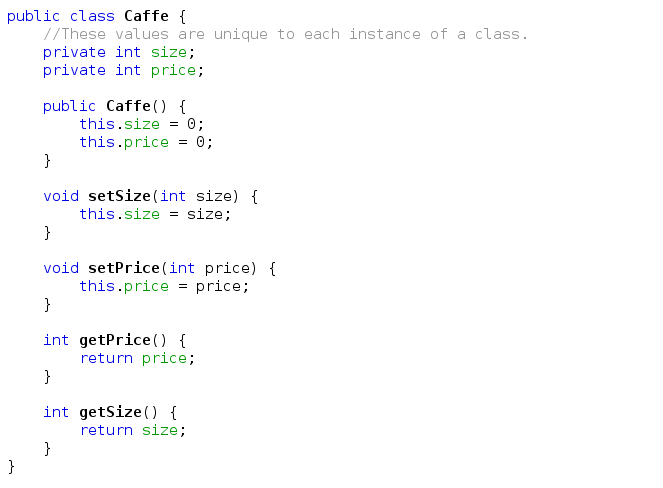
this.name = name;
this.age = age;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
}
If you create two objects of the Person
class:
Person person1 = new Person("John", 30);
Person person2 = new Person("Jane", 25);
person1.setName("Jim");
System.out.println(person1.getName()); // Output: Jim
System.out.println(person2.getName()); // Output: Jane
As you can see, the changes made to name
in person1
do not affect person2
.
On the other hand, a global variable (also known as a static variable) is a member of a class that belongs to the class itself rather than individual instances. In Java, a static variable is shared by all objects created from that class.
Global variables are stored in method area and are garbage collected only when the program terminates. Since they belong to the class, changes made to a global variable affect all objects of the same class.
Here's an updated version of the Person
class with a global variable:
public class Person {
private static String nationality = "Human";
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void setNationality(String nationality) {
Person.nationality = nationality;
}
}
If you create two objects of the Person
class:
Person person1 = new Person("John", 30);
Person person2 = new Person("Jane", 25);
person1.setNationality("Alien");
System.out.println(Person.nationality); // Output: Alien
System.out.println(person1.getNationality()); // Output: Alien
System.out.println(person2.getNationality()); // Output: Alien
As you can see, the change made to nationality
affects all objects of the same class.
In summary:
Instance variables are stored in heap memory and have their own copy for each object created from a class. Global variables (static variables) are shared by all objects of the same class and are stored in method area.