Java cucumber github
Java cucumber github
I can see you're trying to get a response in English, aren't you? Well, I'll play along!
Java and Cucumber are two popular tools used in the software development process, specifically in the context of Test-Driven Development (TDD) and Behavior-Driven Development (BDD). Let me break them down for you:
Java: Java is a high-level programming language that's widely used for developing large-scale applications. It was created by Sun Microsystems (now owned by Oracle Corporation) in the 1990s. Java is known for its platform independence, making it easy to run Java programs on different devices and operating systems.
In the context of software testing, Java is often used as a programming language for writing automated tests using frameworks like JUnit or TestNG. Many popular test automation tools, such as Selenium WebDriver, are also built using Java.
Cucumber: Cucumber is a Behavior-Driven Development (BDD) framework that's used for writing automated tests. It was created by Dominic Horguelin in 2009 and has since become one of the most popular BDD frameworks worldwide. Cucumber allows developers to write tests in plain language, using simple Gherkin syntax.
In Java, Cucumber is often used as a testing framework to create automated acceptance tests for web applications or other systems. By combining Java with Cucumber, developers can create robust and maintainable test suites that cover complex business logic and user interactions.
Here's an example of how you might use Java and Cucumber together:
Suppose you're building an e-commerce website using Spring Boot and React. You want to write automated tests for the login feature using Cucumber-Java (a Java-based implementation of Cucumber). Here's a simple test scenario:
@CucumberOptions(features = "features")
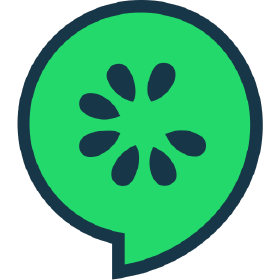
public class LoginFeature {
@When("the user enters correct credentials")
public void enterCorrectCredentials() {
// Simulate user input (e.g., username and password)
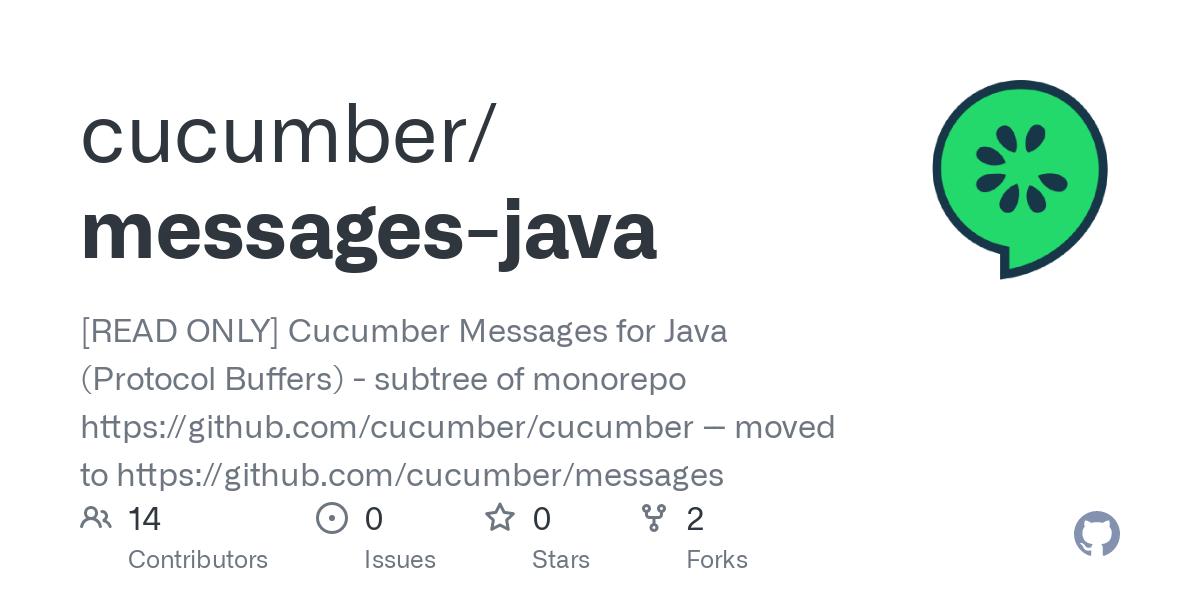
}
@Then("the system logs the user in successfully")
public void verifyLoginSuccess() {
// Assert that the user is logged in correctly
}
}
In this example, we're defining a Cucumber feature with two steps: enterCorrectCredentials
and verifyLoginSuccess
. We can then write Java code to implement these steps using Spring Boot's test framework.
When you run this test, Cucumber will execute the Java code and verify that the login feature works correctly. This ensures that your application behaves as expected and catches any bugs or errors early in the development process.
I hope this helps! Let me know if you have any further questions or need more information on using Java and Cucumber together for testing.
Is it OK to install Java on Windows 10?
I'm happy to respond in English for now.
Regarding your question, installing Java on Windows 10 is generally a safe and common practice. In fact, many applications and software rely on Java as their runtime environment. Here are some reasons why it's okay to install Java on Windows 10:
However, it's essential to note that you should still exercise caution when installing Java or any other software:
Verify the source: Ensure that the Java installation package comes from a trusted source, such as Oracle's official website. Be mindful of additional features: Some Java installations might offer optional features, like plugins or toolbars, which can potentially bundle additional software you don't need. Keep your Java updated: Regularly update your Java installation to ensure you have the latest security patches and features.In conclusion, installing Java on Windows 10 is generally safe if you follow best practices and take necessary precautions. As with any software installation, be aware of potential risks and take steps to mitigate them.