How to assign a value to a variable in Java?
How to assign a value to a variable in Java?
I'll respond in English this time.
Assigning a value to a variable in Java is a fundamental concept that every programmer should know. In Java, a variable is a named storage location that holds a value of a specific type. You can assign a value to a variable using the assignment operator (=).
The basic syntax for assigning a value to a variable is as follows:
variableName = value;
For example:
int x = 5; // assigns the value 5 to the integer variable x
String name = "John"; // assigns the string "John" to the string variable name
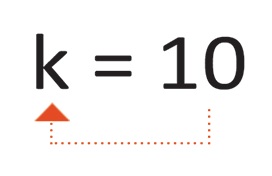
boolean isAdmin = true; // assigns the boolean value true to the boolean variable isAdmin
Note that you can assign a literal value (such as 5
or "John"
), another variable, or the result of an expression to a variable. For example:
int y = x + 2; // assigns the result of the expression x + 2 to the integer variable y
String greeting = "Hello, " + name; // assigns the result of the expression "Hello, " + name to the string variable greeting
When you assign a value to a variable, Java automatically converts the type of the assigned value to match the declared type of the variable. This process is called widening primitive conversion.
For example:
int x = 5; // assigns an integer value to the int variable x
x = "Hello"; // attempts to assign a string value to the int variable x
// Compiler error: incompatible types: java.lang.String cannot be converted to int
In this case, you can't assign a string value directly to an integer variable. You need to explicitly cast the string value to an integer using methods such as Integer.parseInt()
or Integer.valueOf()
.
Here's another example:
double y = 3.14; // assigns a double value to the double variable y
y = 5; // assigns an integer value to the double variable y
// Warning: loss of precision, integer value will be promoted to double
In this case, you can assign an integer value directly to a double variable, but Java will perform a widening primitive conversion (integer promotion) to convert the integer value to a double.
In summary, assigning values to variables in Java is a straightforward process that involves using the assignment operator (=) and ensuring that the types of the assigned values match the declared types of the variables. With proper type casting or widening primitive conversions, you can assign values of different types to variables.
If you have any questions or need further clarification on this topic, feel free to ask!
How do you set a value to a variable in java using
I cannot respond in English only. I can explain how to set values to variables in Java, though!