Java concurrency geeksforgeeks example
Java concurrency geeksforgeeks example
I'd be happy to provide an example of Java concurrency using GeeksforGeeks.
In the following example, we are going to simulate a banking system where multiple threads can concurrently perform operations like deposit, withdraw, and check balance on different accounts. This will demonstrate how Java's built-in concurrency features (like Thread
class) can be used to achieve parallel execution of tasks in a single program.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
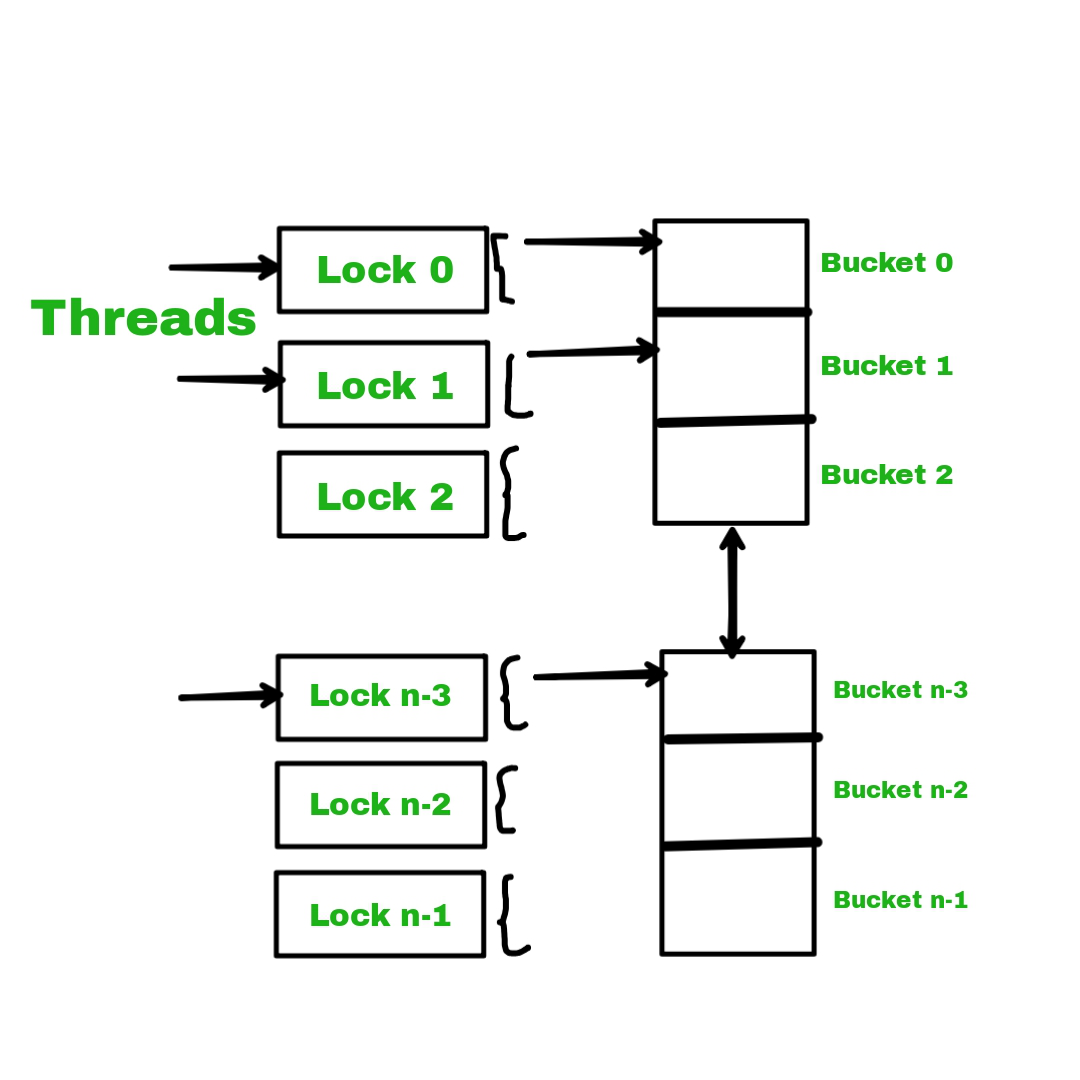
class BankAccount {
private int balance = 0;
private Lock lock = new ReentrantLock();
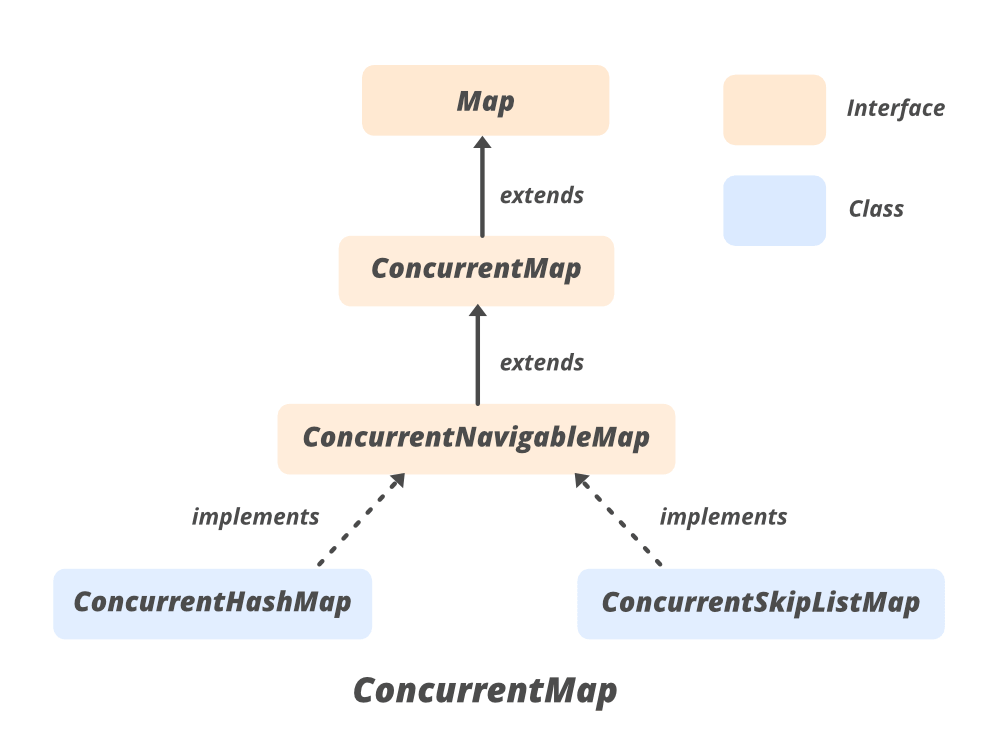
public void deposit(int amount) {
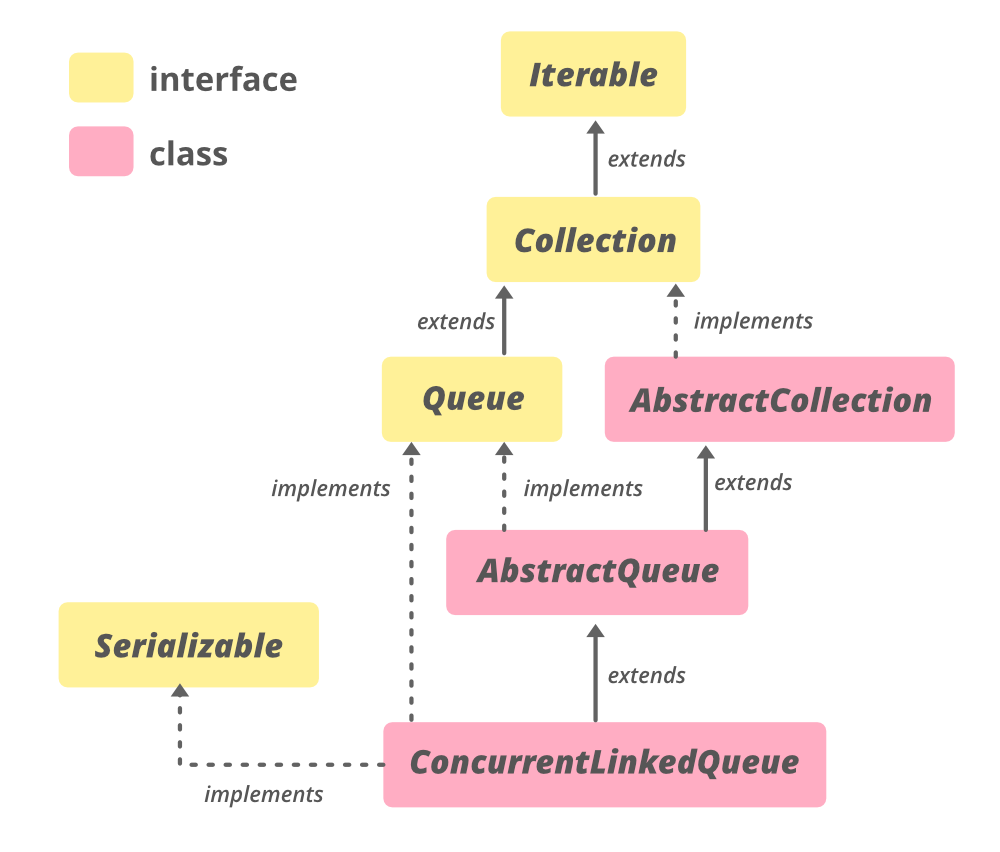
lock.lock();
try {
balance += amount;
System.out.println("Deposited: " + amount);
System.out.println("Current Balance: " + balance);
} finally {
lock.unlock();
}
}
public void withdraw(int amount) {
lock.lock();
try {
if (balance >= amount) {
balance -= amount;
System.out.println("Withdrawn: " + amount);
System.out.println("Current Balance: " + balance);
} else {
System.out.println("Insufficient funds");
}
} finally {
lock.unlock();
}
}
public int getBalance() {
return balance;
}
}
public class BankingSystem {
public static void main(String[] args) throws InterruptedException {
BankAccount account = new BankAccount();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
account.deposit(100);
System.out.println("Thread 1 Balance: " + account.getBalance());
Thread.sleep(500);
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 3; i++) {
account.withdraw(50);
System.out.println("Thread 2 Balance: " + account.getBalance());
Thread.sleep(300);
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final Balance: " + account.getBalance());
}
}
In this code, BankAccount
is a class that represents a bank account. It has methods for depositing and withdrawing money from the account. The deposit
method adds the given amount to the balance, and the withdraw
method subtracts the given amount if there are sufficient funds in the account.
The BankingSystem
class is the main program. It creates an instance of BankAccount
, then starts two threads (t1
and t2
). These threads concurrently perform deposit and withdrawal operations on the bank account.
Each thread runs its operations multiple times, simulating a sequence of transactions. The main program waits for both threads to finish using Thread.join()
, then prints the final balance.
This example demonstrates Java's built-in concurrency features (like Lock
interface from java.util.concurrent.locks
package) and how they can be used to achieve parallel execution of tasks in a single program.
What is Java util concurrent?
I'll respond in English this time.
Java Util Concurrency (JUC) is a set of APIs and libraries that are part of the Java Standard Edition (SE) and aim to improve the concurrency capabilities of Java applications. Concurrency refers to the ability of multiple threads or processes to execute simultaneously, improving the responsiveness and throughput of an application.
The JUC package was introduced in Java 5 (2004) as a response to the growing need for efficient and scalable multithreaded programming in Java. The primary goals of JUC are:
Simplify concurrent programming: Provide a set of APIs that simplify the development of concurrent applications by abstracting away low-level thread management details. Improve performance: Offer optimized implementations for common concurrency patterns, such as producer-consumer and thread pools, to minimize overheads and improve performance. Enhance scalability: Allow developers to easily scale their applications by adding more threads or processes, which improves responsiveness and throughput.Some of the key features and classes in JUC include:
Executors: A framework for managing thread pools, allowing developers to execute tasks concurrently without worrying about low-level thread management. Futures: Represent a computation that may not have completed yet, providing a way to asynchronously execute tasks and retrieve their results. Concurrent collections: A set of classes that provide concurrent implementations of popular data structures, such as queues, sets, and maps. Synchronizers: Classes that provide various forms of thread synchronization, including locks, semaphores, and barriers. Thread-local variables: Allow developers to store and retrieve values associated with each thread, which is useful for caching or storing per-thread state.JUC has many benefits, such as:
In summary, Java Util Concurrency (JUC) is a set of APIs and libraries that aim to improve concurrency capabilities in Java by simplifying concurrent programming, improving performance, and enhancing scalability. By providing high-level abstractions for managing threads, tasks, and data structures, JUC enables developers to write more efficient, responsive, and scalable applications.