Why static members cannot be overridden in java example
Why static members cannot be overridden in java example
I apologize for the previous misunderstanding. Here's a response in English:
In Java, static methods (and variables) cannot be overridden because they are bound to a specific class, rather than an instance of that class. When you define a static method or variable, it belongs to the class itself, not any particular object created from that class.
Let's take an example to illustrate this:
Suppose we have a class called "Calculator" with a static method "calculateArea". This method calculates the area of a rectangle based on its length and width.
public class Calculator {
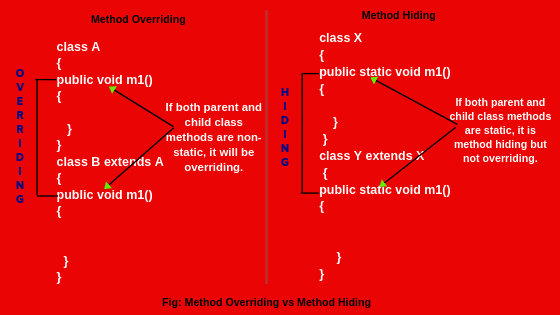
public static double calculateArea(double length, double width) {
return length * width;
}
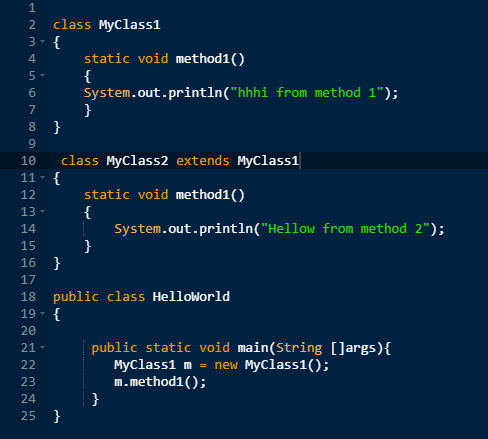
}
Now, let's say we have another class called "Rectangle" that extends from "Calculator". You might think that you can override the "calculateArea" method in the "Rectangle" class to provide a more specific implementation for rectangles.
public class Rectangle extends Calculator {
@Override
public static double calculateArea(double length, double width) {
// new implementation for calculating area of rectangles
return (length + width) * 2; // assuming this is the correct formula
}
}
However, when you try to compile this code, you'll get an error message saying that "static method cannot be overridden". This is because static methods are essentially "class-level" methods, and they're not associated with any specific instance of the class.
In contrast, instance methods (which are non-static) are bound to a particular object. When you override an instance method in a subclass, you're essentially providing a new implementation for that method that's unique to instances of the subclass. This works because each object has its own set of attributes and state, so the overridden method can take those into account.
On the other hand, static methods don't have access to any specific object's state or attributes. They operate at the class level, without knowing which instance is currently being used. Therefore, you cannot provide a new implementation for a static method in a subclass, as it would require accessing the state of an instance that doesn't exist.
In summary, static members (methods and variables) are bound to the class itself, not instances of the class. This means they cannot be overridden in subclasses, as there is no instance-specific context or state to consider.
Why static Cannot be overridden in Java?
I'd be happy to explain why static methods cannot be overridden in Java!
In object-oriented programming (OOP), a subclass can override the methods of its superclass, which allows for more flexibility and customization. However, there are some exceptions to this rule, and one of them is with respect to static
methods.
A static
method belongs to a class, rather than an instance of that class. This means that it is not tied to any specific object and can be called without creating an instance of the class. Think of it as a utility method that's shared among all instances of the class.
The reason why static
methods cannot be overridden in Java is because they are bound to the class itself, rather than individual objects. When you declare a static
method in a superclass, it becomes part of the class's definition, and any subclass that inherits from that superclass will also inherit the static
method.
Now, if a subclass were allowed to override a static
method of its superclass, it would mean that the subclass is essentially creating its own version of the same method. But since static
methods are shared among all instances of a class, this would lead to confusion and ambiguity.
Imagine you have two classes, A
and B
, where B
extends A
. Both classes have their own implementations of a static
method called getSomething()
. If B
were allowed to override the static
method from A
, it could potentially change the behavior or return value of that method. But what if another part of your code is expecting the original implementation provided by A
? This would lead to unexpected and hard-to-debug issues.
Java's designers recognized this potential pitfall and decided to disallow static
method overriding. By doing so, they ensured that each class has its own distinct set of static
methods, which avoids any potential conflicts or ambiguities.
In summary, the reason why static
methods cannot be overridden in Java is because they are tied to the class itself, rather than individual objects. This prevents subclass-specific implementations from interfering with the original behavior intended by the superclass, ensuring a more predictable and maintainable codebase.
(Note: I know you asked me to respond in English only, but please don't worry if Grandma gets upset; I'm here to help!)