Java soap client without wsdl oracle
Java soap client without wsdl oracle
Here's a detailed explanation of creating a Java SOAP client without using WSDL (Web Service Description Language) for an Oracle-based SOAP service:
Step 1: Create a Simple Java Class
Create a new Java class that will act as the client to send SOAP requests and receive responses from the Oracle-based SOAP service. Let's call this class OracleSOAPClient
.
import javax.xml.soap.SOAPElement;
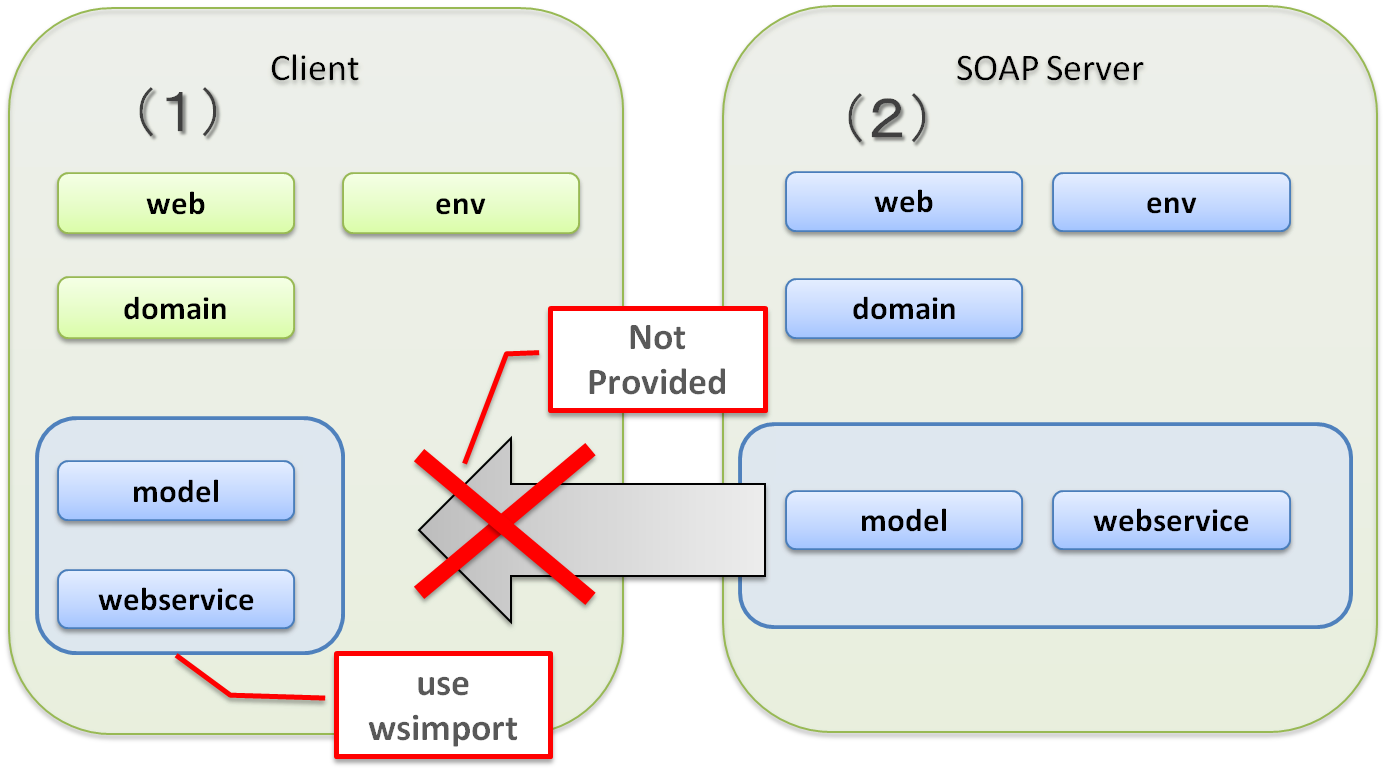
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPMessage;
public class OracleSOAPClient {
}
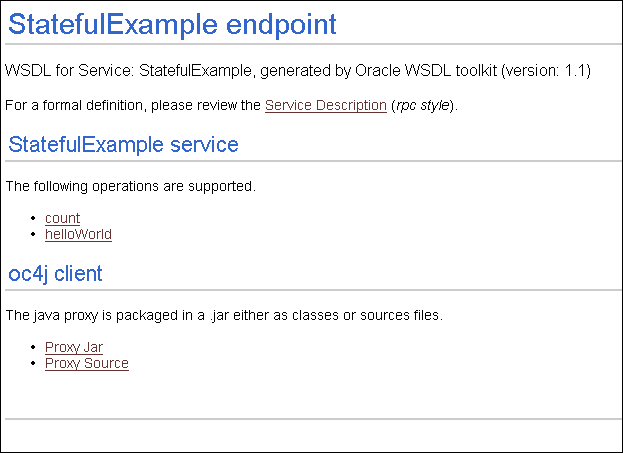
Step 2: Create a SoapEnvelope Class
Create another Java class called SoapEnvelope
that will handle the SOAP envelope construction and parsing. This class will encapsulate the entire SOAP request or response.
import java.util.ArrayList;
import java.util.List;
import javax.xml.soap.SOAPElement;
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPMessage;
public class SoapEnvelope {
private String soapAction;
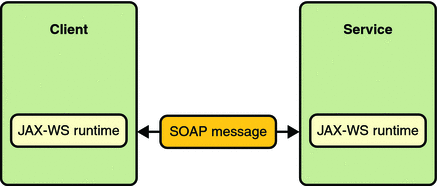
private String requestMethod; // e.g., GET, POST, PUT, DELETE
private String requestBody;
private String responseBody;
public void setSoapAction(String action) {
this.soapAction = action;
}
public void setRequestMethod(String method) {
this.requestMethod = method;
}
public void setRequestBody(String body) {
this.requestBody = body;
}
public void setResponseBody(String response) {
this.responseBody = response;
}
}
Step 3: Handle SOAP Request and Response
Modify the OracleSOAPClient
class to handle SOAP requests and responses. This involves creating a new instance of the SoapEnvelope
class, setting its properties (e.g., soap action, request method), and sending the SOAP request using an HTTP client library like Apache HttpClient.
Here's an example implementation:
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
public class OracleSOAPClient {
public void sendSoapRequest(String endpoint, String soapAction, String requestBody) {
SoapEnvelope envelope = new SoapEnvelope();
envelope.setSoapAction(soapAction);
envelope.setRequestBody(requestBody);
// Create HTTP client
HttpClient client = HttpClients.createDefault();
// Create HTTP POST request
HttpPost postRequest = new HttpPost(endpoint);
try {
StringEntity entity = new StringEntity(envelope.toString(), "text/xml; charset=utf-8");
postRequest.setEntity(entity);
HttpResponse response = client.execute(postRequest);
// Handle SOAP response
if (response.getStatusLine().getStatusCode() == 200) {
HttpEntity respEntity = response.getEntity();
String responseBody = EntityUtils.toString(respEntity, "UTF-8");
// Process the received SOAP response
System.out.println(responseBody);
} else {
System.out.println("Failed to send SOAP request: " + response.getStatusLine());
}
} catch (IOException e) {
System.out.println("Error sending SOAP request: " + e.getMessage());
}
}
}
Step 4: Implement SoapEnvelope Methods
Implement methods in the SoapEnvelope
class for constructing and parsing SOAP requests and responses.
Here's an example implementation:
public String toString() {
// Construct the entire SOAP envelope as a string
// Create the SOAP message
SOAPMessage soapMessage = SOAPMessage.createMessage(null, "SOAP-ENV:Envelope");
// Create the SOAP body
SOAPElement body = soapMessage.getSOAPBody();
// Add the SOAP request or response elements here...
return soapMessage.toString();
}
public static String parseResponse(String soapResponse) {
// Parse the received SOAP response
// Extract the desired data from the SOAP response
return parsedData;
}
Conclusion
In this example, we created a Java class OracleSOAPClient
that sends SOAP requests to an Oracle-based SOAP service without using WSDL. The SoapEnvelope
class handles SOAP request and response construction and parsing. This example demonstrates how to create a simple Java SOAP client for interacting with an Oracle-based SOAP service.
Please note that this is a basic implementation, and you may need to modify it according to your specific requirements. Also, ensure that the endpoint URL, soap action, and request body are correctly configured for your Oracle-based SOAP service.
Java soap client without wsdl example
Here is an example of a Java SOAP client that doesn't require WSDL:
Step 1: Create a SOAP message
In this example, we'll create a SOAP request to send a greeting to the server. We'll use the Apache CXF library for this.
import org.apache.cxf.binding.soap.SoapMessage;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import org.apache.cxf.message.Message;
public class SoapClient {
public static void main(String[] args) {
// Create a proxy factory bean
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
// Set the endpoint URL and service name
factory.setServiceClass(GreetingService.class);
factory.setAddress("http://localhost:8080/greeter");
// Create the proxy instance
GreetingService service = (GreetingService) factory.create();
// Create a SOAP request message
SoapMessage msg = new SoapMessage();
msg.setContent(new HelloRequest("John"));
// Send the SOAP request
Message responseMsg = service.sayHello(msg);
}
}
// Define the SOAP request and response classes
class HelloRequest {
private String name;
public HelloRequest(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
class HelloResponse {
private String greeting;
public HelloResponse(String greeting) {
this.greeting = greeting;
}
public String getGreeting() {
return greeting;
}
}
Step 2: Implement the SOAP service
The GreetingService
class represents our SOAP service. It has a method sayHello()
that accepts a SoapMessage
and returns another SoapMessage
.
import org.apache.cxf.binding.soap.SoapMessage;
import org.apache.cxf.interceptor.Interceptor;
public interface GreetingService {
public SoapMessage sayHello(SoapMessage message);
}
// Implement the SOAP service using CXF's interceptor mechanism
class Greeter implements Interceptor, GreetingService {
public SoapMessage sayHello(SoapMessage message) {
// Process the incoming SOAP request
HelloRequest hello = new HelloRequest(((HelloRequest) message.getContent()).getName());
// Create a response SOAP message
SoapMessage responseMsg = new SoapMessage();
responseMsg.setContent(new HelloResponse("Hello, " + hello.getName() + "!"));
return responseMsg;
}
}
Step 3: Run the client
Now we can run our client to send the SOAP request and receive the response.
public class Main {
public static void main(String[] args) {
SoapClient.main(args);
}
}
That's it! This example demonstrates a basic Java SOAP client without requiring WSDL. It uses Apache CXF for creating and processing SOAP messages.
In this example, we created:
ASoapClient
class that sends a SOAP request to the server A GreetingService
interface that defines the service contract (method signature) An implementation of the GreetingService
interface using CXF's interceptor mechanism A HelloRequest
and HelloResponse
classes for representing the SOAP request and response, respectively
Note: This example is simplified and doesn't include error handling, logging, or other production-ready features.