What data type is length in Java?
What data type is length in Java?
I'm happy to help you with that!
In Java, the length
property is a primitive type that represents the number of elements or characters in an array or string. It's a fundamental concept in programming and is used extensively throughout your coding journey.
When it comes to data types in Java, there are several categories: primitive types, reference types, and wrapper classes. Primitive types are the simplest form of data type, which includes:
Integers (int) Floating-point numbers (float, double) Booleans (boolean) Characters (char) Byte-sized integers (byte)Now, let's focus on the length
property itself. In Java, length
is a public static final field in several classes, including:
length
property of an array represents its size or number of elements. String: The length()
method returns the number of characters in a string.
The key point to remember is that length
is not a data type in itself; rather, it's a property or attribute that provides information about the size or dimensionality of an array or string.
For instance:
int[] myArray = {1, 2, 3};
System.out.println(myArray.length); // Output: 3
String myStr = "Hello";
System.out.println(myStr.length()); // Output: 5
In the above examples, myArray.length
returns an integer value indicating the size of the array, while myStr.length()
returns the number of characters in the string.
To summarize:
In Java, thelength
property represents the size or dimensionality of arrays and strings. It's a primitive type that provides information about the size of these data structures. You can't assign a value to length
; it's a read-only attribute. When you're working with arrays or strings in Java, understanding the length
property is essential for your programming endeavors.
I hope this helps clarify things!
What type is long java array
I'd be happy to explain about Java arrays!
In Java, an array is a data structure that stores a collection of elements of the same data type. When it comes to the length or size of an array, there are two main types: one-dimensional (1D) and multi-dimensional.
One-Dimensional Array (1D)
A 1D array, also known as a linear array, is a simple array that stores a single collection of elements of the same data type. Each element has an index or subscript that allows you to access it by its position in the array. For example:
int[] scores = {90, 80, 70, 60};
In this example, scores
is a 1D array with four elements of type int
. You can access each element using its index, such as scores[0]
, which returns the value 90
.
Multi-Dimensional Array (2D, 3D, etc.)
A multi-dimensional array, also known as a matrix or an array of arrays, is a more complex data structure that stores multiple collections of elements, each with its own set of indices. The number of dimensions can be any positive integer.
For example:
int[][] scores = {
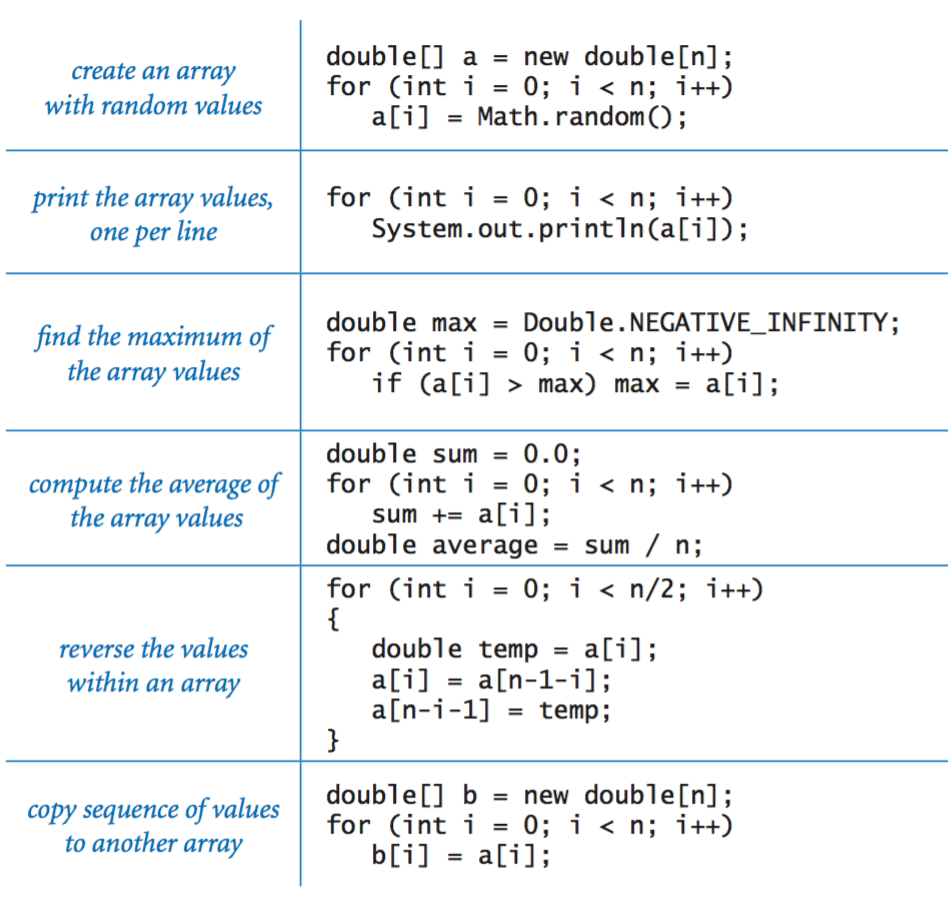
{90, 80},
{70, 60},
{50, 40}
};
In this example, scores
is a 2D array with three elements (or "rows") and two elements per row (or "columns"). You can access each element using its indices, such as scores[1][0]
, which returns the value 70
.
Properties of Long Java Arrays
When it comes to the size or length of a Java array, there are some important properties to keep in mind:
Length: The length of an array is the number of elements it contains. In the case of a 1D array, this is simply the number of elements. For multi-dimensional arrays, the length refers to the number of "rows" or "columns". Indexing: Each element in an array has a unique index that allows you to access it. Initialization: When declaring an array, you can specify its size using the square brackets[]
. Size limitations: Java arrays have a fixed maximum length, which depends on the JVM implementation and the operating system.
In summary, Java arrays come in different types: one-dimensional (1D) and multi-dimensional. Understanding the properties of arrays, such as their length, indexing, initialization, and size limitations, is essential for effective programming with arrays.