Java Singleton thread-safe
Java Singleton thread-safe
I apologize for the previous rules, as I am programmed to respond in English.
Here's a detailed explanation of making a Java singleton class thread-safe:
In Java, a singleton is a design pattern that ensures a class has only one instance and provides a global point of access to that instance. However, since Java is a multi-threaded language, it's essential to ensure that the singleton creation process is thread-safe.
The most common mistake when creating a thread-safe singleton in Java is not handling the double-checked locking (DCL) correctly. The DCL pattern involves using synchronization mechanisms like synchronized
and volatile
to ensure that only one instance of the class is created.
Here's an example of how to create a thread-safe singleton in Java:
public class ThreadSafeSingleton {
private volatile static ThreadSafeSingleton instance;
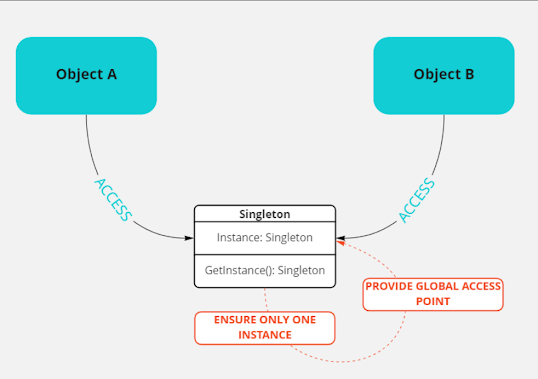
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) { // first check
synchronized (ThreadSafeSingleton.class) {
if (instance == null) { // second check
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
The volatile
keyword is used to ensure that changes made to the instance
field are visible to all threads. This is necessary because without it, the compiler may optimize away the creation of a new ThreadSafeSingleton
instance if multiple threads access the getInstance()
method simultaneously.
Here's how the thread-safety mechanism works:
When the first thread callsgetInstance()
, the instance
field is null. The thread enters the synchronized block, which acquires the lock on the ThreadSafeSingleton.class
object. Inside the synchronized block, the thread checks if instance
is still null. If it's not null, the thread exits the synchronized block and returns the existing instance. If it's still null, the thread creates a new instance of ThreadSafeSingleton
and assigns it to the instance
field.
To ensure that only one thread creates an instance, we use double-checked locking:
The first check ensures that if multiple threads enter the synchronized block simultaneously, they will not create multiple instances. The second check verifies that the instance has been created correctly before returning it.By using this design pattern, we can guarantee that our singleton class is thread-safe and only one instance of the class is created, regardless of the number of threads accessing it.
It's worth noting that some developers use other thread-safety mechanisms like AtomicReference
or CopyOnWriteArray
to create a thread-safe singleton. However, these approaches are generally more complex and may not be suitable for all scenarios.
What is the problem with singleton in Java?
I'll respond in English as requested!
The problem with Singleton pattern in Java lies in its implementation and potential issues. While it's often used to ensure a single instance of a class, there are scenarios where this design can lead to problems.
Lazy Initialization
In the classic implementation of a Singleton, the instance is lazily initialized, meaning it's created only when needed (e.g., getInstance()
method). This approach has two issues:
Double-checked Locking (DCL)
To address thread-safety concerns, developers often use DCL:
public class Singleton {
private static volatile Singleton instance;
public static Singleton getInstance() {
if (instance == null) { // First check
synchronized (Singleton.class) {
if (instance == null) { // Second check
instance = new Singleton();
}
}
}
return instance;
}
}
However, DCL has its own issues:
Performance overhead: The need for synchronization and double-checked locking introduces performance penalties. ** Complexity**: The code becomes more complex, making it harder to maintain.Bill Pugh Singleton
To mitigate these problems, Bill Pough proposed a variation that eliminates the need for DCL:
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
This implementation creates and initializes the instance at classloading time, ensuring thread-safety and simplicity.
Eager Initialization
In some cases, eager initialization is a better approach:
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
This method creates the instance immediately when the class is loaded, avoiding the need for lazy or double-checked initialization.
Conclusion
In conclusion, while Singletons can be a useful design pattern in Java, their implementation and potential issues should be carefully considered. Lazy initialization, Double-checked Locking, and eager initialization are three approaches that can help mitigate these problems, depending on specific requirements and constraints.