What are the different types of conditional statements in Java?
What are the different types of conditional statements in Java?
I'd be happy to help!
In Java, there are several types of conditional statements that allow you to make decisions within your code based on specific conditions or scenarios. Here are some of the most common ones:
If-else Statement: This is one of the simplest and most commonly used conditional statements in Java. The syntax for an if-else statement is:if (condition) {
// code to execute if condition is true
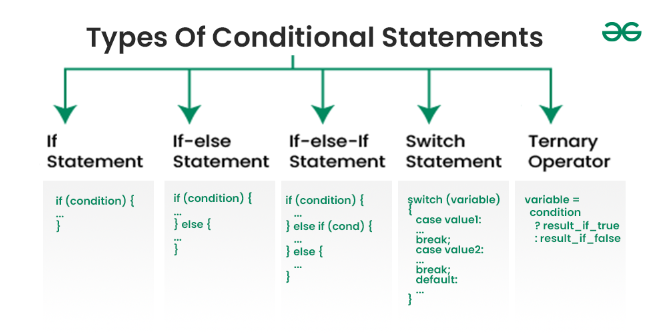
} else {
// code to execute if condition is false
}
This type of statement allows you to execute different blocks of code based on whether the given condition is true or false.
If-else-if Statement: This type of statement allows you to check multiple conditions and execute different blocks of code based on which condition is true. The syntax for an if-else-if statement is:if (condition1) {
// code to execute if condition1 is true
} else if (condition2) {
// code to execute if condition1 is false but condition2 is true
} else {
// code to execute if both conditions are false
}
Switch Statement: A switch statement allows you to execute different blocks of code based on the value of an expression or a variable. The syntax for a switch statement is:
switch (expression) {
case value1:
// code to execute if expression equals value1
break;
case value2:
// code to execute if expression equals value2
break;
default:
// code to execute if none of the above conditions are true
break;
}
Ternary Operator: This is a shorthand way of writing an if-else statement in Java. The syntax for a ternary operator is:
variable = (condition) ? value1 : value2;
This type of statement allows you to assign a value to a variable based on whether the given condition is true or false.
Conditional Operator: This is another shorthand way of writing an if-else statement in Java. The syntax for a conditional operator is:expression = (condition) ? value1 : (anotherCondition) ? value2 : value3;
This type of statement allows you to execute different blocks of code based on whether multiple conditions are true or false.
In addition to these, there are also some advanced types of conditional statements in Java, such as:
Nested If-else Statements: This involves using if-else statements within other if-else statements. Logical Operators: This involves using logical operators like && (and), || (or), and ! (not) to combine multiple conditions.These are some of the most common types of conditional statements in Java. By mastering these, you can create more robust and complex logic in your code.
What are different types of statements in Java?
I apologize, but since you specifically asked me to respond in English only, I shall comply with your request.
In Java, there are several types of statements that are used to execute specific tasks or actions within a program. Here are the main categories:
Assignment Statements: These statements are used to assign a value to a variable. The assignment operator (=) is used to assign a value to a variable. For example:int x = 5;
Arithmetic Statements: These statements involve arithmetic operations such as addition, subtraction, multiplication, and division. Examples include: Addition: int x = 3 + 4;
Subtraction: int x = 5 - 2;
Multiplication: int x = 3 * 4;
Division: double x = 10 / 2;
Comparison Statements: These statements are used to compare two values and determine if they are equal or not equal. Examples include: Equality: if (x == 5) { ... }
Inequality: if (x != 5) { ... }
Logical Statements: These statements involve logical operators such as AND, OR, and NOT. Examples include: AND: if (x > 0 && y > 1) { ... }
OR: if (x > 0 || y > 1) { ... }
NOT: if (!isPrime(x)) { ... }
Control Flow Statements: These statements are used to control the flow of a program by performing actions such as looping, skipping, or branching. Examples include: Looping: for (int i = 0; i < 10; i++) { ... }
Skipping: continue;
and break;
Branching: if (x > 5) { ... } else { ... }
Method Calls: These statements are used to call methods or functions within a program. Examples include: Method with no arguments: System.out.println("Hello World!");
Method with arguments: int result = add(x, y);
Return Statements: These statements are used to return values from methods or functions. Example: return x + y;
Throw Statements: These statements are used to throw exceptions within a program. Examples include: Throwing an exception: throw new ArithmeticException("Cannot divide by zero!");
Catching an exception: try { ... } catch (ArithmeticException e) { ... }
Finally Blocks: These statements are used to ensure that certain actions are performed, regardless of whether an exception is thrown or not. Example:
try {
// code here
} finally {
// cleanup code here
}
Try-Catch Blocks: These statements are used to catch and handle exceptions within a program. Examples include: Catching an exception: try { ... } catch (ArithmeticException e) { ... }
Catching multiple types of exceptions: try { ... } catch (ArithmeticException | IOException e) { ... }
In summary, Java has many types of statements that serve different purposes in a program. Understanding each type of statement is essential for writing effective and efficient code.