What is the difference between synchronized and unsynchronized collections in Java?
What is the difference between synchronized and unsynchronized collections in Java?
I'm happy to help you with that! However, I'll respond in English as per your request.
Synchronized and unsynchronized collections are two fundamental types of collections in Java, each serving a distinct purpose.
Unsynchronized Collections:
In Java, an unsynchronized collection is simply a collection class like ArrayList or LinkedList that does not use locks to ensure thread safety. These collections are designed for single-threaded access only. When you add elements to an unsynchronized collection from multiple threads simultaneously, there's no guarantee about the order in which those elements will be added. This can lead to unexpected results, such as duplicate keys in a HashMap or incorrect ordering in a LinkedList.
For example, suppose you have two threads, thread1
and thread2
, each trying to add an element to an unsynchronized ArrayList called myArrayList
. If thread1
adds an element before thread2
, the resulting order might be different from what you expect. The following code illustrates this:
import java.util.ArrayList;
import java.util.List;
public class UnsynchronizedCollectionExample {
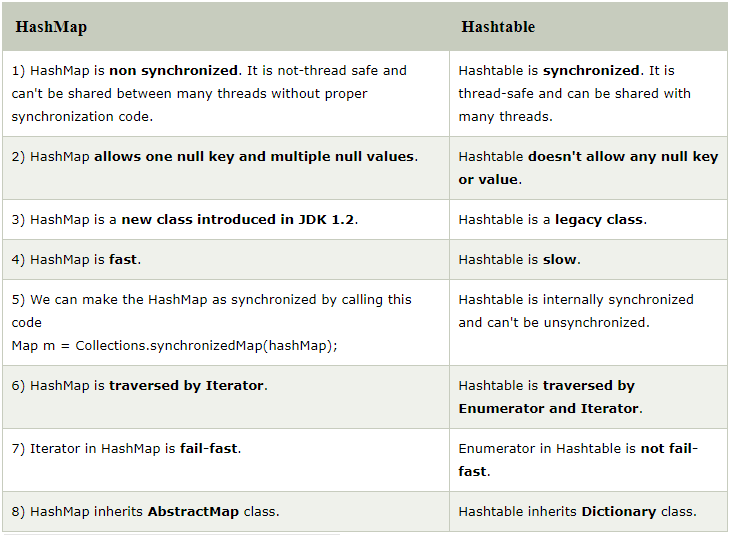
public static void main(String[] args) {
List myArrayList = new ArrayList<>();
new Thread(() -> {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
myArrayList.add("Thread1");
}).start();
new Thread(() -> {
myArrayList.add("Thread2");
}).start();
}
}
In this example, the output might be ["Thread2", "Thread1"]
instead of the expected order.
Synchronized Collections:
To ensure thread safety in collections, Java provides synchronized versions of collection classes. These synchronized collections use locks (mutexes) to protect their internal data structures from concurrent modification by multiple threads.
When you add elements to a synchronized collection from multiple threads simultaneously, the operation is atomic and guaranteed to be executed correctly. The order in which elements are added is also predictable. For instance, if two threads try to add an element to a synchronized ArrayList at roughly the same time, only one of those elements will actually be added.
Here's the modified example using a synchronized ArrayList:
import java.util.ArrayList;
import java.util.List;
public class SynchronizedCollectionExample {
public static void main(String[] args) {
List myArrayList = new ArrayList<>();
// Create a synchronized wrapper around the unsynchronized list.
List syncList = Collections.synchronizedList(myArrayList);
new Thread(() -> {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
syncList.add("Thread1");
}).start();
new Thread(() -> {
syncList.add("Thread2");
}).start();
}
}
In this example, the output will always be ["Thread1", "Thread2"]
, which is the expected order.
In summary:
Unsynchronized collections are designed for single-threaded access and can lead to unpredictable behavior when accessed concurrently from multiple threads. Synchronized collections use locks to ensure thread safety and guarantee atomic operations. They provide predictable ordering of elements added by concurrent threads.When designing applications that require concurrent data access, it's essential to choose the correct type of collection based on your specific needs.
What is the difference between concurrency and synchronization in Java?
I'd be happy to explain the concepts of concurrency and synchronization in Java!
Concurrency
Concurrency refers to the ability of a program or a part of it (a thread, process, or task) to perform multiple tasks simultaneously. In other words, concurrency is about doing several things at the same time, which can improve responsiveness, efficiency, and scalability.
In Java, concurrency is achieved through the use of threads. A thread represents a single execution path in a program, allowing it to run multiple tasks concurrently. There are two primary ways to create threads in Java: 1) using the Thread
class directly or 2) using the more advanced Runnable
and Callable
interfaces.
Synchronization
Synchronization refers to the mechanisms used to control access to shared resources (data, files, network connections, etc.) when multiple threads are accessing them concurrently. Synchronization ensures that only one thread can modify a shared resource at any given time, preventing data corruption or inconsistencies.
In Java, synchronization is achieved through the use of locks, such as synchronized
blocks and methods, as well as more advanced concurrency utilities like ReentrantLock
, Semaphore
, and AtomicReference
.
Key differences
The main difference between concurrency and synchronization lies in their primary goals:
Concurrency: Focuses on achieving multiple tasks simultaneously (parallelism), improving overall system performance. Synchronization: Focuses on controlling access to shared resources, ensuring data consistency and integrity when multiple threads are accessing them concurrently.While it's possible for a program to be concurrent without synchronization, it's crucial to have proper synchronization mechanisms in place when dealing with shared resources. Without synchronization, the likelihood of data corruption, race conditions, or deadlocks increases, which can lead to unexpected behavior or even crashes.
In Java, concurrency is built upon the concept of threads, and synchronization provides the necessary mechanisms to manage thread interactions. Understanding both concepts is essential for designing scalable, efficient, and robust multithreaded applications in Java.
Practical implications
When developing concurrent programs in Java, consider the following:
Usevolatile
variables or AtomicReference
when updating shared state from multiple threads. Employ synchronized
blocks or methods to ensure exclusive access to shared resources. Utilize Lock
objects (like ReentrantLock
) for more fine-grained control over locking and unlocking. Avoid sharing mutable state between threads without proper synchronization.
In summary, concurrency enables simultaneous task execution, while synchronization ensures controlled access to shared resources. Understanding both concepts is vital for building reliable, performant, and efficient multithreaded applications in Java.