local variables in java
local variables in java
I'd be happy to explain local variables in Java!
In Java, a variable declared inside a method or a block is known as a local variable. These variables are only accessible within the scope of that specific method or block where they were declared.
Here are some key characteristics of local variables:
Scope: Local variables have a limited scope, meaning they can only be accessed within the method or block where they were declared. Lifetime: Local variables are created when the method or block is entered and destroyed when it's exited. Accessibility: Only the code inside that specific method or block can access these local variables.When you declare a variable as local, Java automatically initializes it to its default value (e.g., 0
for integer types). You don't need to worry about initializing the variable before using it.
Here's an example of a local variable in Java:
public class MyClass {
public static void main(String[] args) {
int x; // declare a local integer variable
// use the variable
x = 10;
System.out.println("Value of x: " + x);
// you can also redeclare the same variable
int x = 20; // new declaration, same name, different scope
System.out.println("New value of x: " + x);
}
}
In this example, x
is declared as a local integer variable within the main
method. We then use it to store a value and print it to the console.
Notice how we can redeclare the same variable name x
again inside the same method? This is allowed in Java because each declaration creates a new scope for that variable, effectively hiding any previous declarations with the same name.
Local variables are useful when you need to reuse a value within a specific method or block. They help keep your code organized and make it easier to understand what's happening at different parts of your program.
I hope this explanation helps! Do you have any questions about local variables in Java?
local variables in java
Local Variables in Java:
In Java, a local variable is a variable that is declared inside a method, constructor, or block of code. It is called "local" because its scope is limited to the block of code where it is declared. Local variables are used to store temporary values within a specific part of the program.
Declaring Local Variables:
To declare a local variable in Java, you use the type variableName;
syntax. For example:
public void myMethod() {
int myLocalInt;
String myLocalString = "Hello";
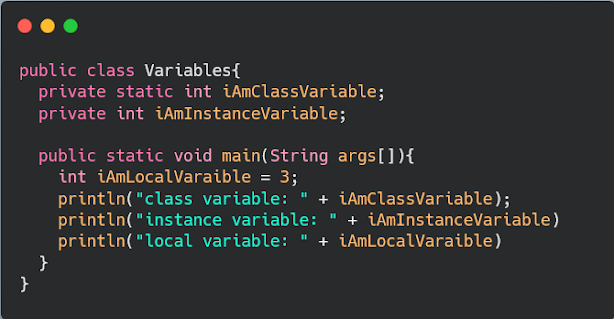
}
In this example, we are declaring two local variables: myLocalInt
, which is an integer, and myLocalString
, which is a string.
Scope of Local Variables:
The scope of a local variable is the area of code where it can be accessed. A local variable's scope begins from its declaration point and ends at the end of the block of code or method where it was declared. For example:
public void myMethod() {
int x = 10;
if (x > 5) {
System.out.println("Value is greater than 5");
int y = 20; // This is a new local variable, with its own scope
System.out.println("Value is " + y);
}
}
In this example, x
has a broader scope because it can be accessed inside the if
block as well. The y
variable, however, only exists within the if
block and cannot be accessed outside of it.
Lifespan of Local Variables:
A local variable's lifespan is the time period from when it is declared to when it goes out of scope. For example:
public void myMethod() {
int x = 10;
{
int y = 20; // This is a new local variable, with its own scope and lifetime
System.out.println("Value is " + y);
}
System.out.println("Value is still " + x);
}
In this example, the y
variable has its own lifespan, which ends when the inner block of code is executed. The x
variable's lifespan continues until the end of the method.
Best Practices:
Declare local variables as close to their usage as possible. Minimize the number of local variables to reduce confusion and improve readability. Use meaningful names for local variables to make your code more self-explanatory.In conclusion, local variables are an essential part of Java programming, allowing you to store temporary values within a specific block of code. By understanding how to declare, scope, and lifespan of local variables, you can write more efficient, readable, and maintainable code.